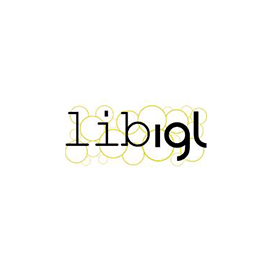
libigl
Open Source C++ Library for 3D Geometry Processing
Free C++ API that provides supports for managing and manipulating 3D shapes. Load, Render and convert 3D meshes inside C++ apps.
libigl is an award-winning open source C++ library for geometry processing that is very easy to use and provides a rich set of data structures and functions for managing and manipulating 3d shapes. The libigl library is a header-only library which means you need to include igl headers before compiling any file. Moreover, libigl is designed with efficiency and ease of use in mind. Its clean and modular codebase allows users to quickly integrate it into their projects and experiment with different algorithms without getting bogged down by implementation details.
The library provides a simple and powerful 3d viewer as well as fully supports loading, rendering, and converting 3d meshes inside C++ applications. libigl is the winner of the Software Award at the Eurographics Symposium on Geometry Processing 2015. The library has included support for some very powerful features such as mesh Booleans, quad remeshing, parameterization, and shape deformation.
The library gives extreme importance to ease of use and experimentation. It has included support for several important features related to loading and handling 3D shapes, such as loading and manipulation of 3d triangle meshes, scaling of 3D shapes, reading triangle meshes, computing affine transformations, 3D scene animating, computing affine transformations, and much more.
Getting Started with libigl
The easiest way to install libigl is by using GitHub. Please use the following command for a successful installation.
Install libigl via GitHub
git clone --https://github.com/libigl/libigl.git
Load and Render 3D Meshes via C++
In 3D computer graphics, a mesh is a collection of vertices, edges, and faces that defines the shape of a 3D object. A vertex is a single point and an edge is a straight line segment connecting two vertices. The open source libigl library enables software developers to programmatically load and render 3D meshes using a couple of lines of C++ code. It provides numerous functions to read and write many common mesh formats with ease. It just requires one-liner code to read a mesh from a file. The following example shows how software developers can load and manipulate mesh data inside C++ applications.
How to Load and Manipulate Mesh using C++ Library?
#include
#include
int main(int argc, char *argv[])
{
// Load a mesh from an OFF file
Eigen::MatrixXd V;
Eigen::MatrixXi F;
igl::readOFF("bunny.off", V, F);
// Translate the mesh
V.col(0) += 1.0;
// Visualize the mesh
igl::viewer::Viewer viewer;
viewer.data.set_mesh(V, F);
viewer.launch();
return 0;
}
Shape Deformation Support
The Shape deformation techniques help you to swiftly optimize existing geometries, and surfaces or meshes. The award-winning libigl library gives software programmers the power to apply different state-of-the-art deformation techniques inside their own applications. It has included support for techniques, ranging from quadratic mesh-based energy minimizers to skinning methods, to non-linear elasticity-inspired techniques.
Apply Animation to 3D Scenes
The Easy3D library has provided functionality for creating and visualizing drawable without associating it with any 3D models. Usually, the drawable are usually created for rendering 3D models or loaded from files. The Easy3D library has included support for visualizing 3D data without explicitly defining a model or you can generate it for a specific rendering purpose or use the viewer to visualize the drawable. Please remember that you need to create a viewer before creating any drawable. The following example shows how to animate a simple transformation, such as rotation, using C++ commands.
How to Apply Animate to Simple Transformation (Rotation) via C++ API?
#include
#include
#include
int main(int argc, char *argv[])
{
// Create a viewer
igl::opengl::glfw::Viewer viewer;
// Load a mesh (replace "bunny.obj" with your own mesh file)
Eigen::MatrixXd V;
Eigen::MatrixXi F;
igl::readOBJ("bunny.obj", V, F);
// Add the mesh to the viewer
viewer.data().set_mesh(V, F);
// Initialize rotation angle
double angle = 0.0;
double angular_speed = igl::PI / 10.0; // 18 degrees per second
// Register a callback function to update the scene
viewer.callback_pre_draw = [&](igl::opengl::glfw::Viewer &) -> bool {
// Update rotation angle
angle += angular_speed * viewer.elapsed_seconds();
// Apply rotation to the mesh
Eigen::MatrixXd V_rotated;
igl::rotate(V, angle, Eigen::Vector3d(0.0, 1.0, 0.0), V_rotated);
// Update mesh in the viewer
viewer.data().set_vertices(V_rotated);
return false;
};
// Launch the viewer
viewer.launch();
return 0;
}