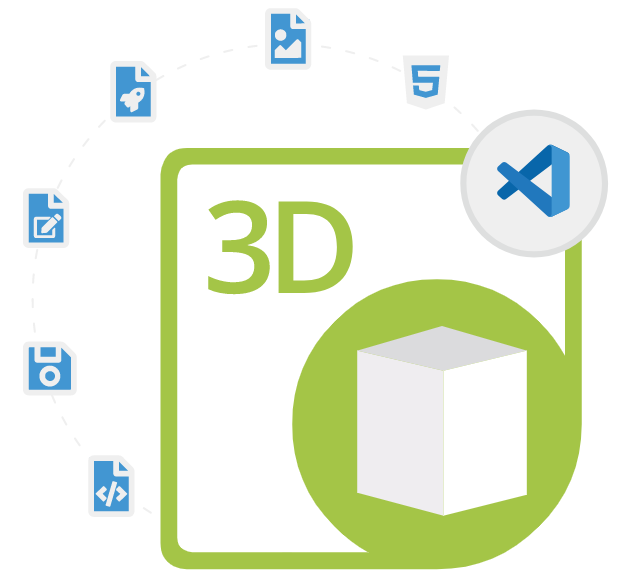
Aspose.3D for Java
Java API to Generate & Convert 3D Diagrams
A useful Computer-Aided-Designing (CAD) API that enables software developers to create, manipulate, and export 3D files in a variety of formats.
Aspose.3D for Java is a powerful and easy-to-use 3D file formats processing library that allows software developers to create, modify, load, manipulate and save 3D formats without any without installing any 3D modeling and rendering software. The library allows software programmers to easily create, modify, and convert 3D models in a variety of formats, including STL, OBJ, FBX, DAE, glTF, DRC, AMF, PLY, 3DS and many more. The library also supports importing 3D models from a variety of sources, such as CAD software, and then modifies them as needed.
Aspose.3D for Java allows software developers to create scenes from scratch without the dependency of any external modeling software. There are several important features parts of the library for handling 3D documents, such as creating 3D document from scratch, reading 3D document, 3D file format detection, customizing Non-PBR to PBR materials conversion, export scene to compressed AMF format, converting 3D scene to HTML, sharing mesh geometry data within multiple nodes, adding animation property to the scene file, split meshes by material, working with geometry and scene hierarchy and many more.
Aspose.3D for Java has included very useful features for working with 3D models, such as 3D mesh optimization, file compression, and file conversion, as well as tools for working with large or complex 3D models. The library also included complete support for 3D graphics rendering and has included a number of powerful rendering tools that allow developers to create high-quality 3D graphics, including lighting effects, texture mapping, and shading. It helps developers to create realistic 3D models that can be used in a variety of different applications, such as video games, architectural design, and engineering simulations.
Getting Started with Aspose.3D for Java
The recommend way to install Aspose.3D for Java is using NuGet. Please use the following command for a smooth installation.
//First, you need to specify the Aspose Maven Repository configuration/location in your Maven pom.xml as follows:
<repositories>
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://releases.aspose.com/java/repo/</url>
</repository>
</repositories>
//Define Aspose.PDF for Java API Dependency
<dependencies>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-3d;/artifactId>
<version>21.4</version>
</dependency>
</dependencies>
You can also download it directly from Aspose product page.3D File Format Conversion via Java API
Aspose.3D for Java gives software developers the power to inter-convert 3D file formats inside their own Java applications without any external dependencies or installing any 3rd party 3D modeling or rendering software. The library has included support for saving documents to various 3D file formats, such as 3DS, 3MF, DAE, DFX, gITF, Collada, glTF, GLB, Draco, RVM, U3D and many more. The library also supports 3D scene conversion to PDF, HTML and more.
How to Save a 3D Scene as HTML via Java API
Scene scene = new Scene();
// Initialize a node
Node node = scene.getRootNode().createChildNode(new Cylinder());
// Set child node properites
LambertMaterial mat = new LambertMaterial();
mat.setDiffuseColor(new Vector3(0.34,0.59, 0.41));
node.setMaterial(mat);
Light light = new Light();
light.setLightType(LightType.POINT);
scene.getRootNode().createChildNode(light).getTransform().setTranslation(10, 0, 10);
// Initialize HTML5SaveOptions
HTML5SaveOptions opt = new HTML5SaveOptions();
// Turn off the grid
opt.setShowGrid(false);
//Turn off the user interface
opt.setShowUI(false);
scene.save(RunExamples.getDataDir() + "html5SaveOption.html", FileFormat.HTML5);
Create 3D Scene via Java API
Aspose.3D for Java has included functionality for creating and handling 3D Scene inside their own Java applications. The library allows saving 3D Scenes in numerous supported file formats such as STL, FBX, Discreet3DS, WavefrontOBJ, Collada, Universal3D, and many more. The library supports several important features for working with 3D scene, such as create empty 3D Scene, load existing 3D Scene, export 3D scenes to other support file format, save 3D scene as HTML, load existing properties, modify existing properties, and many more.
Creating an Empty 3D Scene via Java API
// The path to the documents directory.
String MyDir = RunExamples.getDataDir();
MyDir = MyDir + "document.fbx";
// Create an object of the Scene class
Scene scene = new Scene();
// Save 3D scene document
scene.save(MyDir, FileFormat.FBX7500ASCII);
Working with 3D Mesh in Java Apps
Aspose.3D is a Java API that allows software developers to work with 3D models in a variety of formats, including OBJ, STL, and FBX. The library allows the creation of cylinders with just a couple of lines of Java code. Users can add a material to the cylinder; you can use the setMaterial method, which is part of the Mesh class. The library allows developers to export the cylinder to a different file formats such as OBJ, STL, FBX and many more. The following example shows how to export the cylinder to an OBJ file with ease.
How to Triangulate Mesh via Java API
// The path to the documents directory.
String MyDir = RunExamples.getDataDir();
// Initialize scene object
Scene scene = new Scene();
scene.open(MyDir + "document.fbx");
scene.getRootNode().accept(new NodeVisitor() {
@Override
public boolean call(Node node) {
Mesh mesh = (Mesh)node.getEntity();
if (mesh != null)
{
// Triangulate the mesh
Mesh newMesh = PolygonModifier.triangulate(mesh);
// Replace the old mesh
node.setEntity(newMesh);
}
return true;
}
});
MyDir = MyDir + RunExamples.getOutputFilePath("document.fbx");
scene.save(MyDir, FileFormat.FBX7400ASCII);
Create & Manage Cylinders via Java API
Aspose.3D for Java allows software developers to working with Visio in different ways inside Visio Diagrams using .NET library. The C# library has include different features for handling text in shapes, such as inserting text shape, customize text shape in the Visio diagram, update the shape’s text, find and replace the shape’s text, apply Built-in or custom style-sheet to text, apply different style on the each text value of a shape, extract plain Text from the Visio diagram page and many more.
How to Export the Cylinder to OBJ File via Java API?
import com.aspose.threed.*;
Cylinder cylinder = new Cylinder();
cylinder.setHeight(5);
cylinder.setRadius(2);
Material material = new Material();
material.setAmbientColor(Color.fromArgb(255, 0, 0));
material.setDiffuseColor(Color.fromArgb(255, 0, 0));
material.setSpecularColor(Color.fromArgb(255, 255, 255));
material.setShininess(20);
Mesh mesh = cylinder.toMesh();
mesh.setMaterial(material);
Scene scene = new Scene();
scene.getRootNode().createChildNode("cylinder").attachMesh(mesh);
scene.save("cylinder.obj", FileFormat.OBJ);