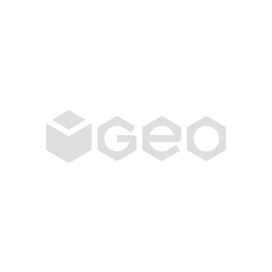
iGeo
Create & Manage 3D Models via Open Source Java API
A Powerful Open Source Java Library that Included Support for Geometries Transformation, Generating NURBS Curves & Surfaces, Manage 3D Vectors & Much more inside Java Applications.
What is iGeo Library?
iGeo is an open source Java 3D modeling library that allows software developers to create and manage 3D models inside their own apps. It has provided components for vector math operations, NURBS curve and surface geometries, managing polygon meshes, 3D display and navigation, and 3D model file I/O. The library has included different shading modes such as wireframe, shaded, transparent shade, shade without edges, and more. The library has the best computational design in architecture, product design, interaction design, and more. One of the standout features of iGeo is its simplicity and ease of use. With just a few lines of code, developers can integrate iGeo into their projects and start visualizing geospatial data in 3D.
The iGeo library is freely available under the GNU Lesser General Public License as published by the free software foundation, version 3. The library has also supported an interface called PiGeon specialized in processing. Tourism is another area where iGeo shines, allowing developers to create interactive maps that showcase points of interest in a compelling 3D format. The library has included features for navigating 3D view, save files, such as zoom, toggle view, rotation, pan, and much more. It has provided several functions for managing panelization such as rectangular panelization, triangulation, diagrid triangulation, diamond panelization, grid lines, diagonal lines & points on the Surface, grid points on the surface, and many more. Whether you're a seasoned developer or a newcomer to the world of geospatial technology, iGeo offers an accessible and powerful solution for bringing your ideas to life in three dimensions.
Getting Started with iGeo
The easiest way to install iGeo is by using GitHub. Please use the following command for a smooth installation.
Install iGeo via GitHub
git clone https://github.com/sghr/iGeo.git
Geometries Transformation via Java API
The open source iGeo API has included functionality for geometrical transformation inside their Java applications. There are several important methods available for geometrical transformation such as duplicating geometry, rotating geometries, reflecting geometry, moving for a specified amount of movement, scaling up or down geometries, scaling geometries only in one direction, shear geometries, and much more.
How to Perform Geometries Transformation using Java API?
import sghr.igeo.Geometry;
import sghr.igeo.Transformation;
public class GeometryTransformationExample {
public static void main(String[] args) {
// Create a sample geometry (e.g., a point)
Geometry point = Geometry.createPoint(0, 0);
System.out.println("Original Point: " + point);
// Apply translation transformation
Transformation translation = Transformation.translation(10, 10, 0);
Geometry translatedPoint = point.transform(translation);
System.out.println("Translated Point: " + translatedPoint);
// Apply rotation transformation
Transformation rotation = Transformation.rotation(90, 0, 0, 1);
Geometry rotatedPoint = point.transform(rotation);
System.out.println("Rotated Point: " + rotatedPoint);
// Apply scaling transformation
Transformation scaling = Transformation.scaling(2, 2, 1);
Geometry scaledPoint = point.transform(scaling);
System.out.println("Scaled Point: " + scaledPoint);
}
}
Generating NURBS Curves and Surfaces
NURBS stands for Non-Uniform Rational B-Spline and it is a mathematical model to define geometries in space. It is one of the most popular mathematical models of geometries and is used in many CAD programs. The iGeo API has included support for creating NURBS Curves and Surfaces using Java commands. The ICurve and ISurface can be used to generate URBS curves and surfaces by providing an array or a 2-dimensional array of IVec and degrees.
Managing 3D Vectors via Java API
The open source iGeo library enables software developers to create and manage their vectors inside their own Java apps. The library has provided several useful functionalities related to vector management such as adding or subtracting vectors, duplicating a vector variable to another variable, multiplying or dividing vectors, flipping vectors, measuring the distance between two vectors, setting the length of a vector, generating one scalar value out of two vectors, reflect a vector on 3-dimensional plane and many more.
How to Manage 3D Vectors inside Java Applications?
import com.sghr.igeo.Vector3;
public class Vector3DExample {
public static void main(String[] args) {
// Creating vectors
Vector3 vector1 = new Vector3(1.0, 2.0, 3.0);
Vector3 vector2 = new Vector3(-2.0, 3.0, 5.0);
// Printing vectors
System.out.println("Vector 1: " + vector1.toString());
System.out.println("Vector 2: " + vector2.toString());
// Adding vectors
Vector3 sum = vector1.add(vector2);
System.out.println("Sum of vectors: " + sum.toString());
// Subtracting vectors
Vector3 difference = vector1.subtract(vector2);
System.out.println("Difference of vectors: " + difference.toString());
// Calculating dot product
double dotProduct = vector1.dot(vector2);
System.out.println("Dot product of vectors: " + dotProduct);
// Calculating cross product
Vector3 crossProduct = vector1.cross(vector2);
System.out.println("Cross product of vectors: " + crossProduct.toString());
// Normalizing vectors
Vector3 normalizedVector1 = vector1.normalize();
Vector3 normalizedVector2 = vector2.normalize();
System.out.println("Normalized Vector 1: " + normalizedVector1.toString());
System.out.println("Normalized Vector 2: " + normalizedVector2.toString());
// Calculating distance between vectors
double distance = vector1.distanceTo(vector2);
System.out.println("Distance between vectors: " + distance);
}
}
Map Images on Geometries
The free iGeo library enables software developers to map images on geometries with ease using Java code. You can control the line length by image, control the depth of the surface by image, control the width of the panel, control rotation by image, control scaling by image, sample color of the image, and so on.
How to Map Images on Geometries using Java API?
import com.sghr.igeo.IG;
import com.sghr.igeo.Map;
import com.sghr.igeo.Model;
import com.sghr.igeo.Sphere;
public class ImageMappingExample {
public static void main(String[] args) {
// Initialize iGeo
IG.init();
// Create a map
Map map = new Map();
// Create a model
Model model = new Model();
// Create a sphere geometry
Sphere sphere = new Sphere(10); // Radius of the sphere
// Load an image to be mapped onto the sphere
String texturePath = "path/to/your/image.jpg"; // Replace this with your image path
model.loadTexture(texturePath);
// Map the image onto the sphere
model.setTexture(sphere, texturePath);
// Add the sphere to the model
model.addGeometry(sphere);
// Add the model to the map
map.addModel(model);
// Set up the camera position and controls
map.setCameraPosition(0, 0, 50); // Set the camera position
map.enableDefaultControls(); // Enable default mouse controls
// Render the map
map.render();
}
}