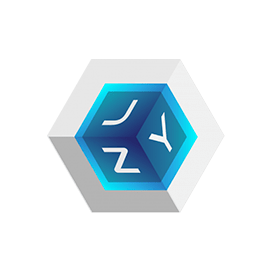
Jzy3d
Open Source Java Library for Working with 3D Charts
Java Library That has Provided Functionality for Drawing 3D Charts and Scientific Data. It Supports Various Chart Types like Surface, Bar and Scatter Charts as well as other Chart Families.
What is Jzy3d?
With just a few lines of Java code, software developers can construct their own apps that can draw charts and 3D scientific data using the open source zy3d plotting framework. Numerous significant features pertaining to surfaces, scatter plots, bar charts, and numerous more 3D primitives are supported by the library. Only a few Java commands are needed to readily alter the axis and chart layout.
The API is incredibly reliable and simple to use into any project, whether personal or professional. Support for a number of significant chart kinds has been added, including surface charts, bar charts, scatter charts, 2D and 3D graph charts, extensive chart settings, and simple chart types like polygons, triangles, and spheres.
Easy-to-use chart layout settings, including color bars, contour functions, tooltips, lights, color mappers for coloring objects, backdrop pictures, 2D post renderers, and much more, are included in the library's extremely versatile layout. The chart is also simple to incorporate into your AWT, Swing, and SWT applications. Several complex features include 2d envelopes, Dual depth peeling, 3d line strip interpolation to smooth pathways, Mouse interaction with objects, Thread Controllers, animation, and much more.
Getting Started with Jzy3d
The easiest way to install Jzy3d is by using GitHub.Please use the following command for a smooth installation.
Install Jzy3d via GitHub
git clone --depth=1 https://github.com/jzy3d/jzy3d-api.git
Draw Surface Chart via Java
The open source Jzy3d library gives software developers the capability to draw surface charts using Java commands. First of all, you need to define the range for the function to plot the chart. After that, you can create the object to represent the function over the given range. Now you can easily draw charts. You draw a simple surface, big surface, Delaunay tesselation, no wireframe surface, and more.
How to Draw a Surface Chart using Java API ?
import org.jzy3d.chart.Chart;
import org.jzy3d.chart.factories.AWTChartComponentFactory;
import org.jzy3d.colors.Color;
import org.jzy3d.maths.Range;
import org.jzy3d.plot3d.builder.Mapper;
import org.jzy3d.plot3d.builder.SurfaceBuilder;
import org.jzy3d.plot3d.primitives.Shape;
public class SurfaceChartExample {
public static void main(String[] args) {
// Define the function to be plotted
Mapper mapper = new Mapper() {
@Override
public double f(double x, double y) {
return Math.sin(x) * Math.cos(y);
}
};
// Define the range of the plot
Range range = new Range(-Math.PI, Math.PI);
// Build the surface
SurfaceBuilder surfaceBuilder = new SurfaceBuilder();
Shape surface = surfaceBuilder.buildOrthonormal(new OrthonormalGrid(range, 50, range, 50), mapper);
// Set surface color
surface.setColor(Color.GREEN);
// Create a chart
Chart chart = AWTChartComponentFactory.chart();
// Add the surface to the chart
chart.getScene().getGraph().add(surface);
// Show the chart
chart.open("Surface Chart Example");
}
}
Create 3D Polar Charts
The Jzy3d library gives software programmers the power to create 3D Polar Charts with ease inside their apps. You can need to set the values for the chart as a dedicated polar dataset allows you to easily make operations on the coordinates. You can define the chart title, the dataset for the chart, chart legend, tooltips, and URLs.
How to Create 3D Polar Charts using Java Library?
import org.jzy3d.chart.Chart;
import org.jzy3d.chart.factories.AWTChartComponentFactory;
import org.jzy3d.colors.Color;
import org.jzy3d.maths.Range;
import org.jzy3d.plot3d.builder.Mapper;
import org.jzy3d.plot3d.builder.SurfaceBuilder;
import org.jzy3d.plot3d.primitives.Shape;
public class SurfaceChartExample {
public static void main(String[] args) {
// Define the function to be plotted
Mapper mapper = new Mapper() {
@Override
public double f(double x, double y) {
return Math.sin(x) * Math.cos(y);
}
};
// Define the range of the plot
Range range = new Range(-Math.PI, Math.PI);
// Build the surface
SurfaceBuilder surfaceBuilder = new SurfaceBuilder();
Shape surface = surfaceBuilder.buildOrthonormal(new OrthonormalGrid(range, 50, range, 50), mapper);
// Set surface color
surface.setColor(Color.GREEN);
// Create a chart
Chart chart = AWTChartComponentFactory.chart();
// Add the surface to the chart
chart.getScene().getGraph().add(surface);
// Show the chart
chart.open("Surface Chart Example");
}
}
Build and Manage 3D Charts via Java
The open source Jzy3d library gives software developers the capability to build and manage 3D charts inside their application using Java commands. The Jzy3d Logarithm Toolbox can be very helpful in drawing 3d charts with log scales very easily. You can also easily edit your charts with ease. It provides dedicated axis, views, ordering strategies, and colormaps suitable for clean logarithmic charts with ease.
Edit Surface Mesh via Java
The Jzy3d library gives software developers the capability to edit their surface meshes via Java code. It has provided SDK that has provided interactive surface editor which enables users to model a surface by grabbing its mesh points with the mouse or trackpad. The surface is presented in an excel-like table that is synchronized with drawing.
How to Edit Surface Mesh inside Java Apps?
import javax.swing.*;
public class Jzy3dSurfaceMeshExample {
public static void main(String[] args) {
// Define the surface function
Mapper mapper = new Mapper() {
@Override
public double f(double x, double y) {
return Math.sin(x) * Math.cos(y);
}
};
// Define the range for x and y
Range range = new Range(-Math.PI, Math.PI);
// Create a surface mesh
Surface surface = new Surface(mapper, range, 50, Color.RED);
// Create a chart
Chart chart = new Chart(Quality.Advanced);
// Add the surface to the chart
chart.getScene().getGraph().add(surface);
// Create a frame for the chart
JFrame frame = new JFrame("Jzy3d Surface Mesh Example");
frame.setSize(800, 600);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Add the chart to the frame
frame.getContentPane().add(chart.getCanvas());
// Make the frame visible
frame.setVisible(true);
// Edit the surface function after a delay
SwingUtilities.invokeLater(() -> {
// Modify the surface function
Mapper newMapper = new Mapper() {
@Override
public double f(double x, double y) {
return Math.sin(x) * Math.sin(y); // Modified function
}
};
// Update the surface with the new function
surface.setMapper(newMapper);
chart.render();
});
}
}