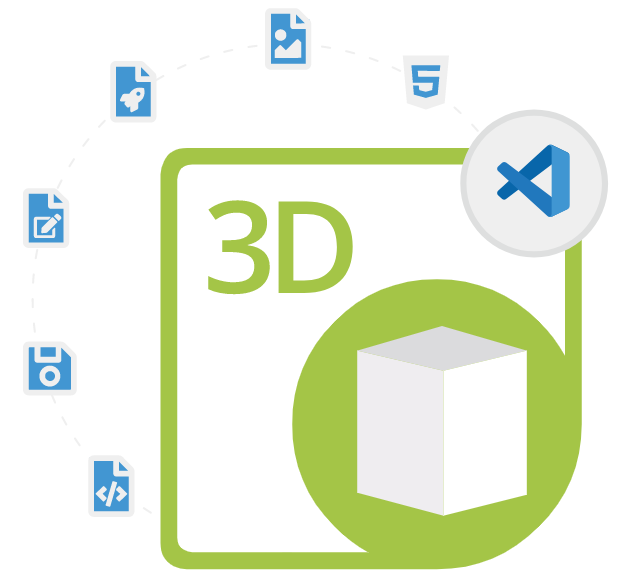
Aspose.3D for .NET
C# .NET API to Create, Edit & Convert 3D Diagrams
A Useful Computer-Aided-Designing (CAD) API that enables Software Developers to Generate 3D Scenes, Manage 3D Mesh, and Export 3D Files in a Variety of Formats.
What is Aspose.3D for .NET?
A feature-rich Computer-Aided-Designing (CAD) API, Aspose.3D for .NET enables .NET developers to produce and work with files in a range of file formats without the need to install 3D modeling and rendering software on the computer. The library's .NET applications may effortlessly incorporate 3D capability, enabling the creation of breathtaking designs and visualizations. Without the need for sophisticated coding or 3D knowledge, developers can quickly and simply incorporate 3D capability into their .NET apps using an easy-to-use API.
Numerous fundamental and sophisticated 3D functions, including mesh geometry processing, animation interpolation, and hierarchical transformations, are supported by Aspose.3D for .NET. The library can open, read, write, modify, and convert 3D files in a number of widely used file types, such as FBX, STL, Collada, AMF, PLY, GLTF, OBJ, and 3DS. The library is incredibly user-friendly and is built to function just as well on the client side as it does on the server. Additionally, it makes it simple for developers to include 3D functionality into their apps by enabling them to interact with a wide range of 3D models and assets.
Creating a 3D scene, loading files from the stream, saving and converting files to a stream, working with geometry, sharing mesh geometry data between multiple nodes, adding animation properties to the scene document, adding a target camera to the scene, splitting meshes by material, rendering a 3D view, creating a cylinder, creating geometry by extruding shapes, and many other features are all made possible by the library. Aspose.3D is always the best for software developers looking for generating stunning 3D visualizations, design interactive 3D applications, or simply add some 3D functionality to their existing .NET applications.
Getting Started with Aspose.3D for .NET
The recommend way to install Aspose.3D for .NET is using NuGet. Please use the following command for a smooth installation.
Install Aspose.3D for .NET via NuGet
dotnet add package Aspose.3D --version 23.2.0
You can also download it directly from Aspose product page.Generate & Save 3D Scene Programmatically via C# .NET API
Aspose.3D for .NET gives software developers the capability to generate new 3D scenes from the scratch and then save in any supported file format inside their own C# applications. The library allows loading existing 3D scenes, add asset information, changing plane orientation, accessing its properties, make changes to it and save it in various popular file formats such as PDF, HTML, and so on. The library also allows saving 3D document in different 3D formats, such as FBX, STL, DAE, RVM, OBJ, 3DS, DRC and so on.
How to Generate a 3D Scene Document via .NET API?
var output = RunExamples.GetOutputFilePath("document.fbx");
// Create an object of the Scene class
Scene scene = new Scene();
// Save 3D scene document
scene.Save(output, FileFormat.FBX7500ASCII);
Convert 3D Document to Other File Formats via .NET
Aspose.3D for .NET allows computer programmers to open and convert 3D document in various supported file formats inside their own .NET applications. Using C# 3D document processing library you just need to load a 3D document in any supported file formats and call its save method with an appropriate FileFormat parameter. The library supports 3D scene conversion to PDF, HTML, FBX, DAE, 3DS, DRC, GLTF, OBJ, STL, RVM and many more.
How to Export 3D Document to Other File Formats via C# API?
// load the file to be converted
var scn = new Aspose.ThreeD.Scene(dir + "template.fbx");
// save in different formats
scn.Save(dir + "output.stl", Aspose.ThreeD.FileFormat.STLASCII);
scn.Save(dir + "output.obj", Aspose.ThreeD.FileFormat.WavefrontOBJ);
Create and Manage 3D Mesh via .NET API
Aspose.3D for .NET gives software developers the capability to work with 3D Meshes inside their own C# applications. The library allows generating a mesh of various 3D geometric shapes, defining control points and polygons in the simplest way to create meshes. The library also supports sharing mesh geometry data with multiple nodes which will help in better memory management. It support several important features such as merging and splitting meshes in 3D file, generating normal data for all meshes in a 3D file, encoding 3D mesh in the Google Draco file, converting mesh of a single 3D object in PLY file, convert mesh to triangle mesh and primitive shape to mesh and so on.
How to Split All Meshes of a Scene per Material via .NET API?
// Create a mesh of box(A box is composed by 6 planes)
Mesh box = (new Box()).ToMesh();
// Create a material element on this mesh
VertexElementMaterial mat = (VertexElementMaterial)box.CreateElement(VertexElementType.Material, MappingMode.Polygon, ReferenceMode.Index);
// And specify different material index for each plane
mat.Indices.AddRange(new int[] { 0, 1, 2, 3, 4, 5 });
// Now split it into 6 sub meshes, we specified 6 different materials on each plane, each plane will become a sub mesh.
// We used the CloneData policy, each plane will has the same control point information or control point-based vertex element information.
Mesh[] planes = PolygonModifier.SplitMesh(box, SplitMeshPolicy.CloneData);
mat.Indices.Clear();
mat.Indices.AddRange(new int[] { 0, 0, 0, 1, 1, 1 });
// Now split it into 2 sub meshes, first mesh will contains 0/1/2 planes, and second mesh will contains the 3/4/5th planes.
// We used the CompactData policy, each plane will has its own control point information or control point-based vertex element information.
planes = PolygonModifier.SplitMesh(box, SplitMeshPolicy.CompactData);
Insert & Edit Text in Visio Diagrams via .NET API
Aspose.3D for .NET allows software developers to working with Visio in different ways inside Visio Diagrams using .NET library. The C# library has include different features for handling text in shapes, such as inserting text shape, customize text shape in the Visio diagram, update the shape’s text, find and replace the shape’s text, apply Built-in or custom style-sheet to text, apply different style on the each text value of a shape, extract plain Text from the Visio diagram page and many more.
How to Setup the Target Camera in 3D File via .NET API?
// Initialize scene object
Scene scene = new Scene();
// Get a child node object
Node cameraNode = scene.RootNode.CreateChildNode("camera", new Camera());
// Set camera node translation
cameraNode.Transform.Translation = new Vector3(100, 20, 0);
cameraNode.GetEntity().Target = scene.RootNode.CreateChildNode("target");
var output = RunExamples.GetOutputFilePath("camera-test.3ds");
scene.Save(output, FileFormat.Discreet3DS);