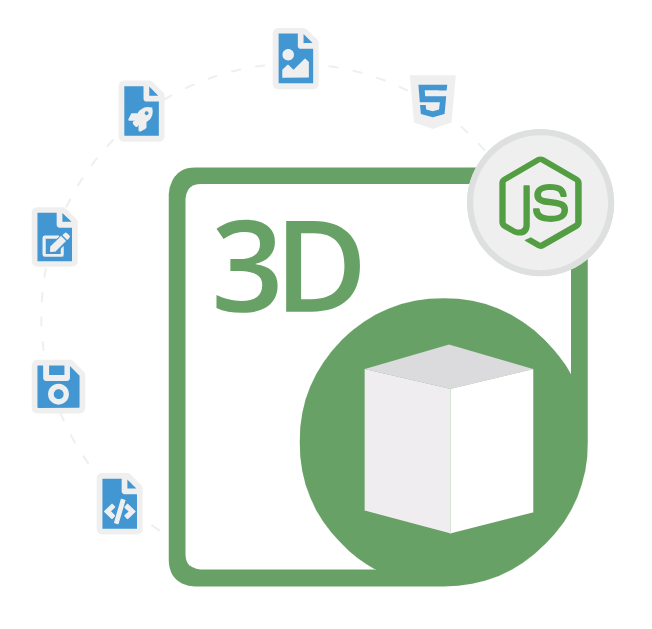
Aspose.3D for Node.js via Java
Node.js API to Generate & Convert 3D Mdoels & Charts
A Useful Node.js 3D API that Enables Software Developers to Create, Edit, Read Manipulate and Convert 3D Models and Charts with Popular Formats, including STL, OBJ, FBX, 3DS, & More.
What is Aspose.3D for Node.js?
Aspose.3D for Node.js using Java API is changing the game in 3D development. It provides a robust set of tools to help you create, read, edit, manipulate, and convert 3D files. This API works across different platforms and can handle many 3D formats such as STL, OBJ, FBX, 3DS, Universal3D, Collada, glTF, GLB, PLY, DirectX, Google Draco, and more. This versatility makes it suitable for a wide range of applications. With advanced scene manipulation, high performance, and easy integration, developers can quickly bring sophisticated 3D models and animations to life.
Developers can easily use Aspose.3D for Node.js with Java to craft new 3D scenes or tweak existing ones. With this tool, you have the power to manipulate objects, fine-tune camera angles, and animate models to breathe life into your ideas. Leveraging Node.js offers the advantage of asynchronous programming, boosting efficiency and responsiveness when managing intricate 3D information. One great thing about Aspose.3D for Node.js is how it works well on different platforms.
This tool is designed to handle 3D tasks effectively, even with intricate models and big sets of data. By offering a user-friendly interface and detailed guides, developers can smoothly add 3D features to their Node.js apps using only a few lines of Java code. By using the Java API, you can smoothly integrate and operate on various operating systems like Windows, macOS, and Linux. This adaptability means developers can create strong 3D applications without concerns about compatibility problems. Whether you’re into developing games, virtual reality apps, or architectural designs, this API offers a wide range of tools to make your work easier. Its intuitive interface and extensive documentation make it easy for developers to get started and achieve desired results efficiently.
Getting Started with Aspose.3D for Node.js via Java
The recommend way to install Aspose.3D for Node.js via Java is using npm. Please use the following command for a smooth installation.
Install Aspose.3D for Node.js via Java using npm
npm install aspose.3d
Install Aspose.3D for Node.js via Java using Maven Repository
//First, you need to specify the Aspose Maven Repository configuration/location in your Maven pom.xml as follows:
<repositories>
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://releases.aspose.com/java/repo/</url>
</repository>
</repositories>
// Then define Aspose.3D for Node.js via Java API dependency in your pom.xml as follows:
<dependencies>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-3d;/artifactId>
<version>23.11.0</version>
</dependency>
</dependencies>
You can also download it directly from Aspose product page.3D Scene Creation & Editing inside Node.js App
Aspose.3D for Node.js via Java allows software developers to create a 3D scenes from the scratch and modify existing 3D scenes inside Node.js applications. Software Developers can load, add, modify, and delete 3D objects, set up lighting, and adjust camera views with ease. This flexibility is crucial for applications ranging from game development to architectural visualization. Here is a very useful example that shows how software developers can create an empty 3D scene with just few Java commands inside Node.js applications.
How to Create an Empty 3D Scene in Node.js Apps?
// The path to the documents directory.
String MyDir = RunExamples.getDataDir();
MyDir = MyDir + "document.fbx";
// Create an object of the Scene class
Scene scene = new Scene();
// Save 3D scene document
scene.save(MyDir, FileFormat.FBX7500ASCII);
Convert 3D Files to Other Formats via Node.js API
One of the standout features of Aspose.3D for Node.js via Java is its ability to load and convert between different leading 3D file formats inside Node.js environment. This ensures that models can be shared and used across various applications without compatibility issues. The library is very easy to handle and has provided complete support for reading and converting 3D file formats to many other supported file formats such as 3DS, 3MF, DAE, DFX, gITF, Collada, glTF, GLB, Draco, RVM, U3D and so on, inside Node.js applications. Here is a very simple example that shows how software developers can load and convert a 3D file inside Node.js applications.
How to Load and Convert a 3D File to other Formats inside Node.js Apps?
const aspose3d = require('aspose3d-node');
// Load a 3D file
let scene = aspose3d.Scene.fromFile('model.obj');
// Convert and save to a different format
scene.save('model.glb', aspose3d.FileFormat.GLTF);
console.log('3D model loaded from OBJ and saved as GLTF.');
Apply Animation to 3D Objects in Node.js
Aspose.3D for Node.js via Java makes it easy for software developers to create and apply animation to 3D objects within 3D scenes inside Node.js applications. Software developers can create and manage animations within 3D scenes. The API supports keyframe animations and can interpolate transformations to produce smooth animations. This feature is particularly useful for game development and interactive applications. The following example shows how software developers can animate a 3D object inside their own Node.js applications.
How to Animate a 3D Object inside Node.js apps?
const aspose3d = require('aspose3d-node');
// Initialize scene and box mesh
let scene = new aspose3d.Scene();
let box = new aspose3d.BoxMesh(1, 1, 1);
let boxNode = new aspose3d.Node(box);
scene.rootNode.addChildNode(boxNode);
// Create an animation clip
let clip = new aspose3d.AnimationClip();
scene.animationClips.add(clip);
// Animate the box: rotate 90 degrees over 1 second
let rotationTrack = clip.createNodeTrack(boxNode);
let quaternionKeyframe = new aspose3d.QuaternionKeyframe();
quaternionKeyframe.time = 1;
quaternionKeyframe.value = new aspose3d.Quaternion.fromEuler(90, 0, 0);
rotationTrack.keyframes.add(quaternionKeyframe);
// Save the animated scene
scene.save('animatedBox.fbx', aspose3d.FileFormat.FBX7400ASCII);
console.log('3D box animated and saved.');
Mesh and Geometry Operations Support
Aspose.3D for Node.js via Java provides tools for creating and editing meshes and geometries. With just a few lines of code the library simplify and optimize meshes for better performance. This includes operations such as mesh smoothing, morphing, decimation, mesh splitting, and Boolean operations, allowing for detailed and complex 3D model manipulation. Moreover, it also supports geometry operations like add transformation to a node, share geometric data of Mesh among multiple nodes of 3D scene, expose geometric transformation and so on.