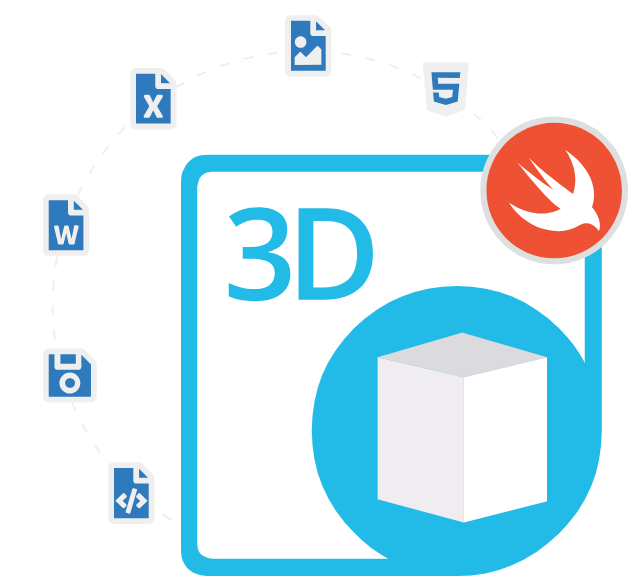
Aspose.3D Cloud Perl SDK
Perl SDK to Create, Edit, Manipulate & Convert 3D Files
A Powerful REST API to Create, Edit, Manipulate & Convert 3D Models including FBX, STL, OBJ, 3DS, OBJ and Extract 3D Scene inside Cloud Apps.
What is Aspose.3D Cloud Perl SDK ?
In the ever-changing world of software development, the need for strong tools to make difficult tasks easier keeps increasing. With technology progressing, it’s vital to include advanced features like 3D modeling in applications. If you’re a software developer exploring 3D modeling and processing, the Aspose.3D Cloud Perl SDK can be your trusted partner. It acts as a smooth connection between Perl applications and cutting-edge 3D features. The SDK encapsulate security best practices, ensuring that software developers are using secure methods to communicate with the Aspose.3D Cloud API.
One great thing about the Aspose.3D Cloud Perl SDK is how easily it works with the Aspose.3D Cloud API. This software tool makes it simple for developers to add powerful 3D features to their Perl apps. The SDK acts like a connector, making it easier for developers to use the API for 3D modeling. The library includes key features like creating a 3D scene from the beginning, 3D scenes saving in PDF, 3D modeling manipulation, extracting scenes from a 3D file, convert 3D file to other formats, parametric modeling support, Create 3D entities, working with triangulate meshes and many more.
The Aspose.3D Cloud Perl SDK is super user-friendly. It comes with built-in support for various top 3D file formats like FBX, STL, VRML, ASE, OBJ, 3DS, U3D, DAE, DRC, GLTF, RVM, PDF, AMF, PLY, X, JT, DXF, 3MF, HTML, and more. Plus, it’s designed to work smoothly on different platforms. So, no matter which platform you’re using, you can easily add 3D features to your Perl applications. To wrap up, the Perl SDK is like a guiding light for software developers exploring the exciting world of 3D modeling and processing. By smoothly integrating with APIs, being adaptable across different platforms, being easy to use, and offering continuous support, this SDK enables you to enhance your Perl applications with the amazing capabilities of 3D technology.
Getting Started with Aspose.3D Cloud Perl SDK
The recommended way to install the Aspose.3D Cloud Perl SDK is using Comprehensive Perl Archive Network (CPAN). Please use the following command for a smooth installation.
Install Aspose.3D Cloud Perl SDK via CocoaPods
cpan AsposeCloud::3D
You can download the compiled shared library from Aspose.3D Cloud Perl SDK product page.
3D Files Conversion to PDF in Clouds
Aspose.3D Cloud Perl SDK has provided complete support for loading and converting 3D models to other supported file formats like PDF, XPS, JPEG, PNG and many more. There are several important features part of the library helping users to smoothly complete the conversion process. It supports converting whole of the 3D file as well converting a specific part only to PDF. The following example demonstrates how Perl developers can convert an existing 3D file to PDF file using Perl commands.
How to Convert 3D Model to PDF using Perl SDK?
# Get your ClientId and ClientSecret from https://dashboard.aspose.cloud (free registration required).
my $config = AsposeThreeDCloud::Configuration->new('base_url' => 'https://api.aspose.cloud');
my $client = AsposeThreeDCloud::ApiClient->new( $config);
my $oauth_api = AsposeThreeDCloud::OAuthApi->new($client);
my $grant_type = 'client_credentials';
my $client_id = 'MY_CLIENT_ID';
my $client_secret = 'MY_CLIENT_SECRET';
my $result = $oauth_api->o_auth_post(grant_type => $grant_type, client_id => $client_id, client_secret => $client_secret);
my $access_token = $result->access_token;
my $new_config = AsposeThreeDCloud::Configuration->new('access_token' => $access_token, 'base_url' => 'https://api.aspose.cloud/v3.0');
$new_client = AsposeThreeDCloud::ApiClient->new( $new_config);
my $api = AsposeThreeDCloud::ThreeDCloudApi->new($new_client)
my $name = 'Aspose.Upload.3d';
my $newformat = 'pdf';
my $newfilename = 'saveasformat.pdf';
my $folder = '3DTest';
my $is_overwrite = 'true';
my $storage = undef;
my $result = $api->post_convert_by_format(name => $name, newformat => $newformat, newfilename => $newfilename, folder => $folder, is_overwrite => $is_overwrite, storage => $storage);
Create & Manage 3D Entities via Perl
Aspose.3D Cloud Perl SDK enables software developers to create new 3D entities from the scratch inside 3D model programmatically using Perl code. The SDK supports generation of popular entities like Box, Sphere, Plane, Torus, Cylinder, and so on. Developers can easily adjust the parameters such as width, height, depth, name, and folder according to their own requirements. Below is a simple example that shows how developers can a basic 3D box using Perl code.
How to Create a Basic 3D Box via Perl API?
use AsposeCloud::3D::ApiClient;
use AsposeCloud::3D::Configuration;
my $config = AsposeCloud::3D::Configuration->new(
app_sid => 'YourAppSID',
app_key => 'YourAppKey',
);
my $api_client = AsposeCloud::3D::ApiClient->new(config => $config);
use AsposeCloud::3D::ObjectByThreeDsModelApi;
use AsposeCloud::3D::CreateEntityRequest;
use AsposeCloud::3D::Box;
my $object_api = AsposeCloud::3D::ObjectByThreeDsModelApi->new(api_client => $api_client);
my $box = AsposeCloud::3D::Box->new(
width => 5.0, # Replace with your desired width
height => 5.0, # Replace with your desired height
depth => 5.0, # Replace with your desired depth
);
my $create_entity_request = AsposeCloud::3D::CreateEntityRequest->new(
name => 'Box', # Replace with your entity name
box => $box,
folder => 'your_folder', # Replace with your desired folder
);
my $result = $object_api->post_create_entity(request => $create_entity_request);
if ($result->status eq 'OK') {
print "Entity created successfully.\n";
} else {
print "Error: " . $result->status . "\n";
}
Extract 3D Scene to Other Formats via Perl API
Aspose.3D Cloud Perl SDK makes it easy for software developers to extract a 3D scene and convert it to other formats inside Perl applications. This features can be predominantly useful when developing applications that require access to metadata or specific attributes of a model. Moreover, the SDK provides useful functions for retrieving details regarding mesh statistics, vertex count, material information, and much more. Below is a simple example that shows how to extracts a 3D scene from a file and converts it to a different format like GLTF.
How to Extract a 3D Scene from a File and Convert It to GLTF Format?
use AsposeCloud::3D::SceneApi;
use AsposeCloud::3D::SaveOptions;
my $scene_api = AsposeCloud::3D::SceneApi->new(api_client => $api_client);
my $input_path = 'input_scene.obj'; # Replace with the path to your input 3D scene file
my $output_path = 'output_scene.gltf'; # Replace with the desired output format and path
my $save_options = AsposeCloud::3D::SaveOptions->new(
save_format => 'gltf', # Replace with your desired output format
file_name => $output_path,
);
my $result = $scene_api->post_export_scene(file => $input_path, save_options => $save_options);
if ($result->status eq 'OK') {
print "Scene extracted and converted successfully. Output saved at $output_path\n";
} else {
print "Error: " . $result->status . "\n";
}
Security & Cross-Platform Flexibility
Security is paramount in the digital landscape, and the Aspose.3D Cloud Perl SDK doesn't compromise. Built-in security measures ensure that data exchanges between the SDK and the Aspose.3D Cloud API adhere to best practices. This commitment to security enables developers to build robust and secure applications that leverage the power of 3D modeling. Recognizing the diverse development environments in today's tech ecosystem, the SDK ensures cross-platform compatibility. Developers can seamlessly incorporate 3D functionalities into their Perl applications, regardless of the platform they are working on. This flexibility enhances the SDK's accessibility, catering to a wide audience of developers with varying preferences.