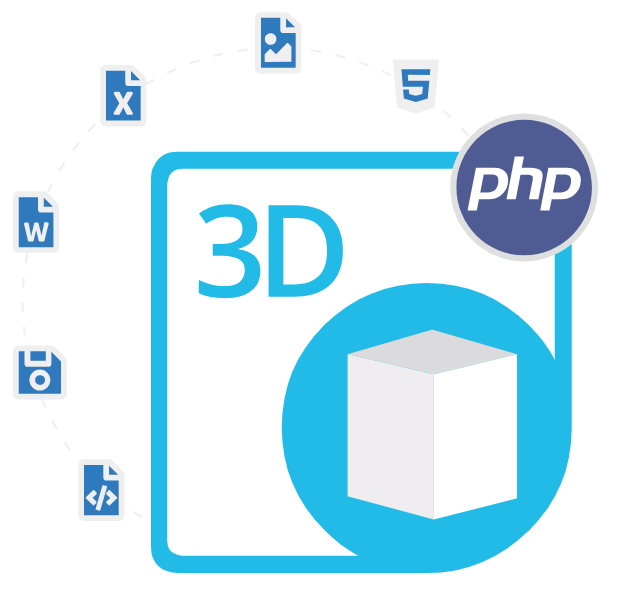
Aspose.3D Cloud PHP SDK
Swift SDK to Generate, Edit & Convert 3D Files
A Powerful 3D software development kit (SDK) that allows Swift developers to create, modify, render, manipulate, and convert 3D file formats.
Aspose.3D Cloud PHP SDK is a powerful and compressive solution that simplifies 3D modeling creation and handling for software developers using PHP commands. With this SDK, programmers can create, modify, and convert 3D models in various 3D file formats. The PHP SDK is based on the cloud, which means software developers can use it from anywhere in the world, as long as the internet connection is available to them. The SDK is also very helpful for game development, as it allows software developers to create animated 3D models for games with ease.
Aspose.3D Cloud PHP SDK is very easy to use, even for software developers who are not familiar with 3D modeling. The SDK provides a user-friendly interface that allows computer programmers to create, modify, view, render, build and convert 3D models quickly. There are several important features part of the library for managing 3D models with ease, such as create 3D entities, export 3D files to other file formats, convert a particular part of a 3D models, 3D scenes extraction, triangulate meshes handing, extracting raw data from a password-protected 3D PDF, 3D modeling & data processing and many more.
Aspose.3D Cloud PHP SDK enables software developers to create 3D models from scratch or modify existing models inside their own cloud PHP applications. It has included supports working with numerous popular 3D file formats such as FBX, STL, OBJ, 3DS, U3D, DAE, GLTF, DRC, RVM, PDF, AMF, PLY, X, JT, DXF, 3MF, ASE, VRML, HTML and many more. The SDK is scalable, which means developers can use it to create 3D models of any size and complexity. The SDK can handle large and complex 3D models without compromising performance.
Getting Started with Aspose.3D Cloud PHP SDK
The easiest way to install Aspose.3D Cloud PHP SDK stable release is using Composer. Please use the following command for a smooth installation.
Install Aspose.3D Cloud PHP SDK via Composer
composer require aspose/3d-cloud-sdk-php
You can download the compiled shared library from Aspose.3D Cloud PHP SDK product page.
Create 3D Entities using PHP REST API
Aspose.3D Cloud PHP SDK provides capability allowing software developers crate and add a new entity with custom size to their 3D models inside PHP applications. The library supports various entity types creation such as Box, Cylinder, Sphere, Torus, Plane and so on. The SDK support some useful features for updating the entity properties or delete the unwanted entities. It is also possible to delete unwanted nodes from a scene with just a couple of lines of PHP code. The following example shows how to create 3D entities using PHP commands.
How to Create a 3D Sphere using PHP API?
use Aspose\ThreeD\Api\ThreeDApi;
use Aspose\ThreeD\Model\Sphere;
$threeDApi = new ThreeDApi();
// Set up sphere parameters
$sphere = new Sphere();
$sphere->setRadius(10);
$sphere->setSegments(20);
// Create sphere
$result = $threeDApi->postCreateOrUpdate(new \SplFileObject("sphere.fbx"), $sphere);
Extract 3D Scene & Save It in Other Format via PHP
Aspose.3D Cloud PHP SDK has included complete functionality for programmatically loading existing 3D files and extract a part of the 3D scene and Save it in other supported file formats in the cloud. The PHP SDK has included support for conversion to various 3D documents such as 3DS, AMF, RVM, DRC, FBX, gLTF, OBJ, PDF, PLY, STL, U3D, DXF, 3MF, and several others. Software developers can also extract raw data from a password protected PDF File and use it according to their needs.
Uploaded 3D File & Convert It to STL Format via PHP
// Upload the 3D file
$filename = "yourfilename";
$localFile = realpath("yourfilepath");
$response = $client->uploadFile(
new \SplFileObject($localFile, 'rb'),
$filename,
null
);
//Convert the uploaded file to the desired format
$result = $client->threeDApi->convertByFormat(
$filename,
"stl", //desired output format
null,
null,
null,
null
);
// Download the converted file
$outputFilePath = "/path/to/output/stlfile.stl";
$client->downloadFile($result, $outputFilePath);
Create & Manage 3D Meshes via PHP API
Aspose.3D Cloud PHP SDK allows software developers to create and manage 3D meshes inside their own PHP applications. It enables software developers to handle meshes and its properties with ease. There are several important features part of the library that can help developers to manage meshes such as loading existing meshes, reading a triangle mesh, cropping meshes, print its vertices and triangles, mesh subdivision, mesh simplification, vertex clustering, mesh decimation and many more. The following simple example shows how to work with 3D meshes using PHP code.
Work with 3D Meshes using PHP Code
$asposeApp = new Aspose\Cloud\Common\AsposeApp('client_id', 'client_secret');
$product = new Aspose\Cloud\Common\Product('3D');
$utils = new Aspose\Cloud\Common\Utils();
$utils->setBasePath('https://api.aspose.cloud/v3.0');
$converterApi = new Aspose\Cloud\3D\ConverterApi($asposeApp, $product);
$folder = new Aspose\Cloud\Storage\Folder();
$folder->uploadFile('model.obj', 'path/to/model.obj');
$result = $converterApi->convertByFormat('model.obj', 'stl');
$folder->downloadFile('model.stl', 'path/to/model.stl');
Working with 3D Scene via PHP API
Aspose.3D Cloud PHP SDK is a powerful tool for working with 3D scenes in the cloud. The API has included several important features for working with 3D sciences, such as accessing and reading existing 3D scenes, modify existing scenes, 3D file format detection, convert 3D scene in the PDF, opening an existing scenes from a password-protected PDF, extracting 3D contents from a PDF file, and many more. The following example shows how software developers can upload a 3D file and use the SDK to convert 3D files to different formats in the cloud.
Load & Convert OBJ File to FBX Format via PHP API
// upload a 3D file
use Aspose\ThreeD\Configuration;
use Aspose\ThreeD\ThreeDCloudApi;
$config = new Configuration();
$config->setAppKey("your App Key");
$config->setAppSid("your App SID");
$apiInstance = new ThreeDCloudApi(null, $config);
$fileName = "example.3ds"; // file to upload
try {
$result = $apiInstance->uploadFile($fileName);
print_r($result);
} catch (Exception $e) {
echo 'Exception when calling ThreeDCloudApi->uploadFile: ', $e->getMessage(), PHP_EOL;
}
// convert a file from OBJ to FBX format:
use Aspose\ThreeD\Configuration;
use Aspose\ThreeD\ThreeDCloudApi;
$config = new Configuration();
$config->setAppKey("your App Key");
$config->setAppSid("your App SID");
$apiInstance = new ThreeDCloudApi(null, $config);
$inputFile = "example.obj"; // file to convert
$format = "fbx"; // desired output format
try {
$result = $apiInstance->postConvertByFormat($inputFile, $format);
print_r($result);
} catch (Exception $e) {
echo 'Exception when calling ThreeDCloudApi->postConvertByFormat: ', $e->getMessage(), PHP_EOL;