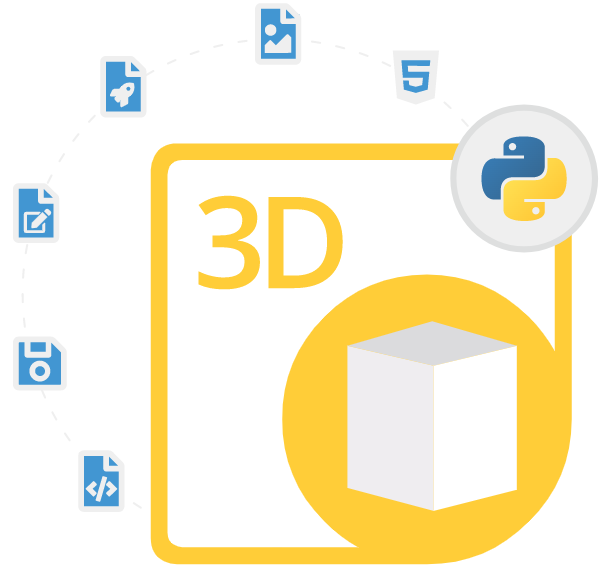
Aspose.3D for Python via .NET
Python API to Create, Edit & Convert 3D Models
A Useful Python Library for 3D File Format and 3D Scenes Creation, Editing, Processing & Conversion to FBX, STL, Discreet3DS, Universal3D, Collada & more.
What is Aspose.3D for Python via .NET ?
Aspose.3D for Python via .NET is a comprehensive and stable library that enables software developers to work with 3D files and models inside Python applications without 3D modeling and rendering software being installed. The library helps software developers to create applications that can easily create, read, render, manipulate, and convert 3D models in various file formats, including FBX, 3DS, U3D, DAE, glTF, OBJ, STL, DRC, PDF, AMF, PLY, and many others. The library uses a variety of techniques to ensure that 3D operations are performed efficiently and quickly, even on large and complex 3D models.
Aspose.3D for Python via .NET has included several important features for working with 3D animations, such as creating 3D and manipulating 3D animations, such as key-frame animations, skeletal animations, and morph animations. This makes it easy to create engaging and interactive 3D applications and games. The library also provides a wide range of tools and features for manipulating 3D models. This includes the ability to import and export 3D models, create and edit 3D models, and perform various operations on 3D models, such as scaling, rotation, and translation.
Aspose.3D for Python via .NET is built on top of the .NET framework and can be used with Python using IronPython, which is a .NET implementation of the Python programming language. With its support for a wide range of 3D file formats and its rich set of features for manipulating and animating 3D models, it is an excellent choice for developers who need to work with 3D content in their applications.
Getting Started with Aspose.3D for Python via .NET
The easiest way to install Aspose.3D for Python via .NET stable release is using pip. Please use the following command for a smooth installation.
Install Aspose.3D for Python via .NET via pip
pip install aspose-3d
You can also download it directly from Aspose product page.Generate & Load 3D Scene via Python API
Aspose.3D for Python via .NET has included complete support for creating new 3D scenes from the scratch and then saving it in any supported file format using Python commands. The library also provided support for reading existing 3D Scenes, making changes to existing 3D, saving a 3D scene in the PDF, opening existing scenes from a password-protected PDF, detecting a file format, extracting 3D contents from a PDF file, setting load options before loading a 3D Obj file and many more.
How to Create a 3D Scene Document via Python API?
import aspose.threed as a3d
# For complete examples and data files, please go to https://github.com/aspose-3d/Aspose.3D-for-.NET
# The path to the documents directory.
# Create an object of the Scene class
scene = a3d.Scene()
# Save 3D scene document
scene.Save("document.fbx", a3d.FileFormat.FBX7500ASCII)
3D Scene Rendering via Python API
Aspose.3D for Python via .NET enables software developers to load and render 3D scenes inside their own Python applications. The library supports high-quality rendering of 3D scenes, including anti-aliasing, depth of field, motion blur, and more. It also supports importing and exporting various 3D file formats, including FBX, OBJ, STL, and 3DS. This means you can easily import existing 3D models into your scene or export your 3D scene to a compatible format. It supports features like cast and receiving shadows on 3D geometries, hardware-based rendering of 3D geometry, rendering 3D view in image format from the camera, rendering a Panorama view of the 3D scene, and so on. The following code example shows how software developers can render a Panorama view of a 3D scene and save in the image format.
How to Render Panorama View of 3D Scene & Save It in Image Format via Python?
from aspose.pydrawing.imaging import ImageFormat
from aspose.pydrawing import Color
import aspose.threed as a3d
# load the scene
scene = Scene.from_file("vc.glb")
# create a camera for capturing the cube map
cam = a3d.entities.Camera(a3d.entities.ProjectionType.PERSPECTIVE)
cam.near_plane = 0.1
cam.far_plane = 200
cam.rotation_mode = a3d.entities.RotationMode.FIXED_DIRECTION
scene.root_node.create_child_node(cam).transform.set_translation(5, 6, 0);
# create two lights to illuminate the scene
scene.root_node.create_child_node(a3d.entities.Light("", a3d.entities.LightType.POINT).transform.set_translation(-10, 7, -10)
light = a3d.entities.Light()
light.color = Color.cadet_blue
scene.root_node.create_child_node(light).transform.set_translation(49, 0, 49)
# create a renderer
# Create a cube map render target with depth texture, depth is required when rendering a scene.
rt = renderer.render_factory.create_cube_render_texture(a3d.render.RenderParameters(False), 512, 512)
# create a 2D texture render target with no depth texture used for image processing
final = renderer.render_factory.CreateRenderTexture(a3d.render.RenderParameters(False, 32, 0, 0), 1024 * 3 , 1024)
# a viewport is required on the render target
rt.create_viewport(cam, a3d.utilities.RelativeRectangle.from_scale(0, 0, 1, 1))
renderer.render(rt)
# execute the equirectangular projection post-processing with the previous rendered cube map as input
equirectangular = renderer.get_post_processing("equirectangular")
# Specify the cube map rendered from the scene as this post processing's input
equirectangular.input = rt.targets[0]
# Execute the post processing effect and save the result to render target final
renderer.execute(equirectangular, final)
# save the texture into disk
final.targets[0].save("panorama.png", ImageFormat.PNG)
Work with Animation in 3D Document
Aspose.3D for Python via .NET provides allows software developers to create and work with animations inside 3D documents using the Python library. The library has included numerous features for working with animations, such as rendering the animated scene, setup the target camera in a 3D file, creating skeletal animations, working with morph targets, and blending multiple animations. The following example demonstrates how to create a simple animation using Python commands.
How to Create a Simple Animation using Python API?
import clr
clr.AddReference("Aspose.3D")
from Aspose.ThreeD import *
# create a new scene
scene = Scene()
# create a new camera
camera = Camera()
camera.Name = "Camera"
scene.RootNode.CreateChildNode(camera)
# create a new mesh
mesh = Mesh()
mesh.Name = "Mesh"
vertices = Vector3[]
vertices.append(Vector3(0, 0, 0))
vertices.append(Vector3(0, 1, 0))
vertices.append(Vector3(1, 1, 0))
vertices.append(Vector3(1, 0, 0))
mesh.Vertices = vertices
mesh.Triangles = [0, 1, 2, 0, 2, 3]
# create a new node for the mesh
meshNode = Node()
meshNode.Name = "MeshNode"
meshNode.Translation = Vector3(0, 0, 0)
meshNode.CreateChildNode(mesh)
# add the mesh node to the scene
scene.RootNode.CreateChildNode(meshNode)
# create a new animation track for the mesh node
track = AnimationTrack(meshNode, AnimationTargetType.Translation)
# create a new keyframe at time 0
keyframe1 = Keyframe()
keyframe1.Time = 0
keyframe1.Value = Vector3(0, 0, 0)
track.Keyframes.Add(keyframe1)
# create a new keyframe at time 1
keyframe2 = Keyframe()
keyframe2.Time = 1
keyframe2.Value = Vector3(0, 0, 5)
track.Keyframes.Add(keyframe2)
# create a new animation clip
clip = AnimationClip("Clip")
clip.Duration = 1
clip.Tracks.Add(track)
# add the animation clip to the scene
scene.Animations.Add(clip)
# save the scene to a file
scene.Save("animation.fbx", FileFormat.FBX7400ASCII)
Work with Polygons using Python API
Aspose.3D for Python via .NET enables software developers to add and manage polygon meshes in their 3D models inside Python applications. The library has included support for various features related to polygon mesh management, such as loading a polygon mesh from a file, creating a polygon mesh, converting all polygons to triangles in a 3D model, exporting the polygon mesh to a file, and many more. The following example shows how users can create a polygon mesh by creating a Mesh object and adding vertices and faces to it.
How to Create a Polygon Mesh using Python API?
# Create a mesh
mesh = Mesh()
# Add vertices
mesh.Vertices.Add(Vector3(0, 0, 0))
mesh.Vertices.Add(Vector3(0, 0, 1))
mesh.Vertices.Add(Vector3(0, 1, 0))
mesh.Vertices.Add(Vector3(1, 0, 0))
# Add faces
mesh.Faces.AddPolygonFace(0, 1, 2)
mesh.Faces.AddPolygonFace(0, 1, 3)
mesh.Faces.AddPolygonFace(0, 2, 3)
mesh.Faces.AddPolygonFace(1, 2, 3)
# Export the polygon mesh to a file
# Create a scene and add the mesh to it
scene = Scene()
scene.RootNode.CreateChildNode(mesh)
# Save the scene to a file
scene.Save("mesh.obj", FileFormat.WavefrontOBJ)