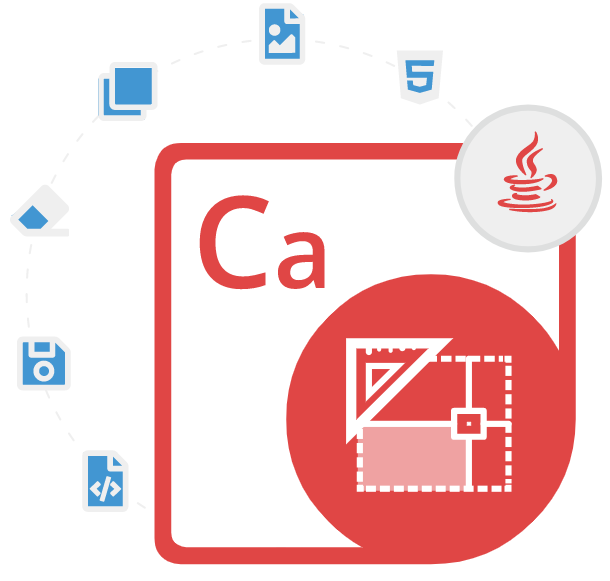
Aspose.CAD for Java
Java API to Create, Edit & Convert CAD Drawings
It enables Software Developers to Create, Edit & Convert AutoCAD DWG, DXF & STL Documents to PDF & Raster Images (BMP, GIF, JPG & JPEG) without any Dependencies.
What is Aspose.CAD for Java?
Aspose.CAD for Java is a versatile Java library that empowers software developers like you to work with AutoCAD DWG, STL, DWF, DWT, and DXF Documents without needing AutoCAD or any other CAD software. This library allows you to open, read, render, manipulate, and convert these files to PDF and Raster Images. With an intuitive API, you can easily handle CAD files in your Java applications. Plus, it’s thread-safe, so you can use it in multiple threads hassle-free.
Aspose.CAD for Java comes with a handy feature that lets you convert various CAD drawing formats like DXF, DWG, DWT, DGN, IFC, DWF, DWFX, STL, IGES(IGS), CF2, Collada(DAE), PLT, OBJ, SVG, DXB, FBX, U3D, 3DS, STP files to PDF, along with supported raster image formats like PNG, BMP, TIFF, JPEG & GIF using just a few lines of Java code. It’s user-friendly and built for speed and efficiency, consuming minimal memory and delivering optimized performance. It uses advanced algorithms to ensure that operations on CAD files are fast and responsive.
Aspose.CAD for Java offers advanced functions like displaying and printing CAD files in Java apps. A standout feature is its capability to handle and transform CAD files. With this tool, developers can make, adjust, and remove CAD elements like lines, circles, arcs, and text. You can also change characteristics like color, layer, and line type of objects. Aspose.CAD for Java fully backs 3D models, letting you handle them just like 2D models. In essence, it’s a user-friendly Java tool with top-notch capabilities and great customer assistance, making it perfect for Java developers dealing with CAD files in their apps.
Getting Started with Aspose.CAD for Java
The recommend way to install Aspose.CAD for Java is via Maven repository. You can easily use Aspose.CAD for Java API directly in your Maven Projects with simple configurations.
Maven repository for Aspose.CAD for Java
//First, you need to specify the Aspose Maven Repository configuration/location in your Maven pom.xml as follows:
<repositories>
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://releases.aspose.com/java/repo/</url>
</repository>
</repositories>
//Define Aspose.PDF for Java API Dependency
<dependencies>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-cad;/artifactId>
<version>22.12</version>
<classifier>20.12</classifier>
</dependency>
</dependencies>
You can download the library directly from Aspose.CAD product page
Export CAD Files via Java API
Aspose.CAD for Java enables software developers to convert various AutoCAD formats to other support file formats with just a couple of lines of Java code. The library allows loading various CAD file formats such as DXF & DWG and converts it to PDF and raster images without needing AutoCAD or any other application. The library has provided several important functions for CAD files conversion, such as export 3D AutoCAD images to PDF, export CAD layouts to PDF, set pen properties options while exporting diagram, decompose cad objects and process separate entities inside insert, ACAD Proxy Entities, read and export IGES format, implement and count mesh models like edges, vertices and faces, set custom point of view for Model layout and many more.
How to Export 3D AutoCAD Images to PDF via Java API?
String dataDir = Utils.getDataDir(Export3DAutoCADImagesToPDF.class) + "ExportingCAD/";
String srcFile = dataDir + "conic_pyramid.dxf";
Image cadImage = Image.load(srcFile);
CadRasterizationOptions rasterizationOptions = new CadRasterizationOptions();
rasterizationOptions.setPageWidth(500);
rasterizationOptions.setPageHeight(500);
rasterizationOptions.setTypeOfEntities(TypeOfEntities.Entities3D);
rasterizationOptions.setLayouts(new String[] {"Model"});
PdfOptions pdfOptions = new PdfOptions();
pdfOptions.setVectorRasterizationOptions(rasterizationOptions);
cadImage.save(dataDir + "Export3DImagestoPDF_out_.pdf", pdfOptions);
Render CAD & BIM File Formats via Java API
Aspose.CAD for Java gives software developers the ability to programmatically render CAD and BIM file formats inside their own Java applications. The library allows loading various CAD file formats such as AutoCAD DWG, DWF, DWT or DXF files and convert it to JPEG, PNG, PSD, BMP, DICOM, WebP, EMF, WMF, SVG, PDF and many more with just a couple of lines of Java code. The below example demonstrates how to load DWG file, set the page width and height of the output file and save DWG files to PDF inside Java applications.
How to Render DWG files via Java API?
// Load the CAD file
Image image = Image.load("input.dwg");
// Create an instance of CadRasterizationOptions
CadRasterizationOptions rasterizationOptions = new CadRasterizationOptions();
rasterizationOptions.setPageWidth(1000);
rasterizationOptions.setPageHeight(1000);
// Set the render options
PdfOptions pdfOptions = new PdfOptions();
pdfOptions.setVectorRasterizationOptions(rasterizationOptions);
// Save the output file
image.save("output.pdf", pdfOptions);
Export AutoCAD DGN to PDF via Java API
Aspose.CAD for Java is a powerful API that allows software developers to work with AutoCAD DGN files inside their own Java applications. The library supports AutoCAD DGN files conversion to PDF as well as raster Image file formats such as PNG, BMP, TIFF, JPEG and GIF with ease. To achieve the goal first you need to load an existing DGN file as DgnImage and set different properties for it, after that Call the save method of the DgnImage class object to save the output file in the format of your choice.
How to Convert AutoCAD DGN Format to PDF via Java API?
// load an existing DGN file as DgnImage.
DgnImage dgnImage = (DgnImage)Image.load(dataDir + "Nikon_D90_Camera.dgn");
// Create an object of CadRasterizationOptions class and define/set different properties
PdfOptions options = new PdfOptions();
CadRasterizationOptions vectorOptions = new CadRasterizationOptions();
vectorOptions.setPageWidth(1500);
vectorOptions.setPageHeight(1500);
vectorOptions.setNoScaling(true);
vectorOptions.setAutomaticLayoutsScaling(false);
options.setVectorRasterizationOptions(vectorOptions);
OutputStream outStream = new FileOutputStream(dataDir + "ExportDGNToPdf_Out.pdf");
// Call the save method of the DgnImage class object.
dgnImage.save(outStream, options);