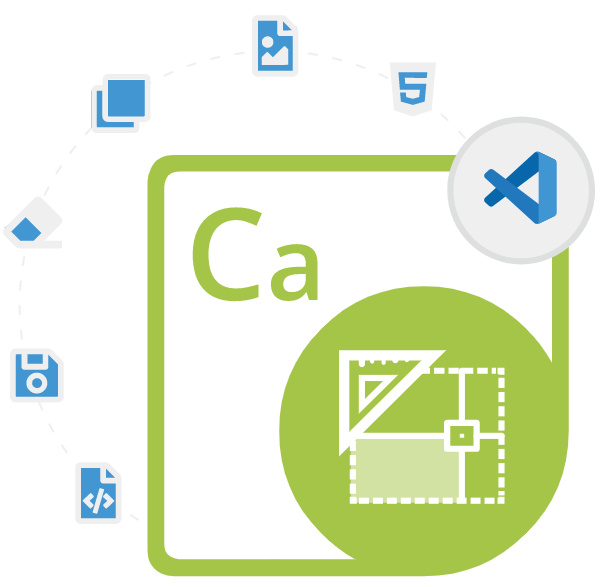
Aspose.CAD for .NET
C# .NET API to Create, Edit & Convert CAD Drawings
A Powerful CAD Drawings Processing API that enabels software developers to load, read, process, & convert CAD & BIM Files.
Aspose.CAD for .NET is a standalone very powerful CAD and BIM documents processing API for reading, manipulating, processing, and converting numerous CAD and BIM file formats. The library enables developers to create applications that can handle complex and sophisticated CAD drawings. The library has included support for loading (inputting) various file formats, such as DWG, DXF, DWT, DGN, DWF, DWFX, IFC, STL, IGES, PLT, CF2, OBJ, HPGL, and IGS. With just a couple of lines of .NET code, it is possible to convert these support file formats to PDF, WMF, SVG, EMF, BMP, GIF, JPG, JPEG, DICOM, WEBP, JP2, JPEG2000, PNG, TIFF, and PSD.
Aspose.CAD for .NET is designed to be highly productive and scalable making it easy for software developers to incorporate CAD functionality into their applications. It's advanced caching and memory management capabilities ensure that even the largest and most complex CAD drawings can be processed quickly and efficiently. This makes the library an ideal solution for applications that need to work with large amounts of CAD data.
Aspose.CAD for .NET has included complete support for both 2D and 3D drawings making it a versatile tool for working with a wide range of CAD formats. There are several important features part of the library, such as adjusting CAD drawing size, exporting DWG/DXF drawings and layouts into specified sizes, SHX fonts export, setting a timeout on save, and many more. Overall, it is a versatile library that provides developers with a wide range of tools for working with CAD drawings. Whether you're building a desktop application, a web application, or a mobile app, Aspose.CAD can help you take your CAD functionality to the next level.
Getting Started with Aspose.CAD for .NET
The recommend way to install Aspose.CAD for .NET is using NuGet. Please use the following command for a smooth installation.
Install Aspose.CAD for .NET via NuGet
NuGet\Install-Package Aspose.CAD -Version 23.1.0
You can also download it directly from Aspose product page.AutoCAD Drawings Conversion to PDF via .NET
Aspose.CAD for .NET has included a very useful feature for converting AutoCAD DXF as well as DWG drawings to PDF file format without any external dependencies. The library allows developers to customize the CAD conversion process and has provided many optional features that upon setting can override the rendering process according to the application needs. Developers can easily set the canvas size, customize background & drawing colors, set auto layout scaling, tracking of CAD rendering process, substitute the required fonts with the available fonts, export CAD layouts to PDF, add pen options in the export properties and many more.
AutoCAD 3D File Export to PDF via .NET API
// The path to the documents directory.
string MyDir = RunExamples.GetDataDir_ConvertingCAD();
string sourceFilePath = MyDir + "conic_pyramid.dxf";
using (Aspose.CAD.Image cadImage = Aspose.CAD.Image.Load(sourceFilePath))
{
Aspose.CAD.ImageOptions.CadRasterizationOptions rasterizationOptions = new Aspose.CAD.ImageOptions.CadRasterizationOptions();
rasterizationOptions.PageWidth = 500;
rasterizationOptions.PageHeight = 500;
// rasterizationOptions.TypeOfEntities = TypeOfEntities.Entities3D;
rasterizationOptions.Layouts = new string[] { "Model" };
PdfOptions pdfOptions = new PdfOptions();
pdfOptions.VectorRasterizationOptions = rasterizationOptions;
MyDir = MyDir + "Export3DImagestoPDF_out.pdf";
cadImage.Save(MyDir, pdfOptions);
}
Export AutoCAD DWG & DXF to Raster Image via C#
Aspose.CAD for .NET has included very useful functionality for exporting AutoCAD DWG & DXF drawings to raster image formats with just a couple of lines of C# code. The library allows conversion to PNG, BMP, TIFF, JP2, PSD, DICOM, WEBP, JPEG and GIF file formats with ease. The library has included some useful features for working with AutoCAD drawing export, such as exporting AutoCAD DXF or DWG layouts, tracking CAD rendering process, CAD Layers conversion to raster image file formats, all CAD layers conversion to separate Images and many more.
Convert AutoCAD DWG or DXF to Images Formats via C# API
// The path to the documents directory.
string MyDir = RunExamples.GetDataDir_ConvertingCAD();
string sourceFilePath = MyDir + "conic_pyramid.dxf";
using (Aspose.CAD.Image image = Aspose.CAD.Image.Load(sourceFilePath))
{
// Create an instance of CadRasterizationOptions
Aspose.CAD.ImageOptions.CadRasterizationOptions rasterizationOptions = new Aspose.CAD.ImageOptions.CadRasterizationOptions();
// Set page width & height
rasterizationOptions.PageWidth = 1200;
rasterizationOptions.PageHeight = 1200;
// Create an instance of PngOptions for the resultant image
ImageOptionsBase options = new Aspose.CAD.ImageOptions.PngOptions();
// Set rasterization options
options.VectorRasterizationOptions = rasterizationOptions;
MyDir = MyDir + "conic_pyramid_raster_image_out.png";
// Save resultant image
image.Save(MyDir, options);
}
Add Text & Manage CAD Size via C# API
Aspose.CAD for .NET has included complete support for handling text and images inside CAD drawings with ease. The library has included several important features for handling text entities, such as add new text entities, modify existing text, setting up quality options for the text, inserting MTEXT entities to the right of existing ones, and many more. The library also provides complete support for programmatically adjusting CAD drawing size. It has included two important options for handling CAD drawing size. The first one is Automatic size adjustment and the 2nd one is adjust size by using the UnitType enumeration. The developers don’t need to provide the width and height properties for the automatic option. The following example shows how to achieve it.
Atomic Scaling of CAD Drawings via C# API
string MyDir = RunExamples.GetDataDir_ConvertingCAD();
string sourceFilePath = MyDir + "sample.dwg";
// Load a CAD drawing in an instance of Image
using (var image = Aspose.CAD.Image.Load(sourceFilePath))
{
// Create an instance of BmpOptions class
Aspose.CAD.ImageOptions.BmpOptions bmpOptions = new Aspose.CAD.ImageOptions.BmpOptions();
// Create an instance of CadRasterizationOptions and set its various properties
Aspose.CAD.ImageOptions.CadRasterizationOptions cadRasterizationOptions = new Aspose.CAD.ImageOptions.CadRasterizationOptions();
bmpOptions.VectorRasterizationOptions = cadRasterizationOptions;
cadRasterizationOptions.CenterDrawing = true;
// Set the UnitType property
cadRasterizationOptions.UnitType = Aspose.CAD.ImageOptions.UnitType.Centimenter;
// Set the layouts property
cadRasterizationOptions.Layouts = new string[] { "Model" };
// Export layout to BMP format
string outPath = sourceFilePath + ".bmp";
image.Save(outPath, bmpOptions);