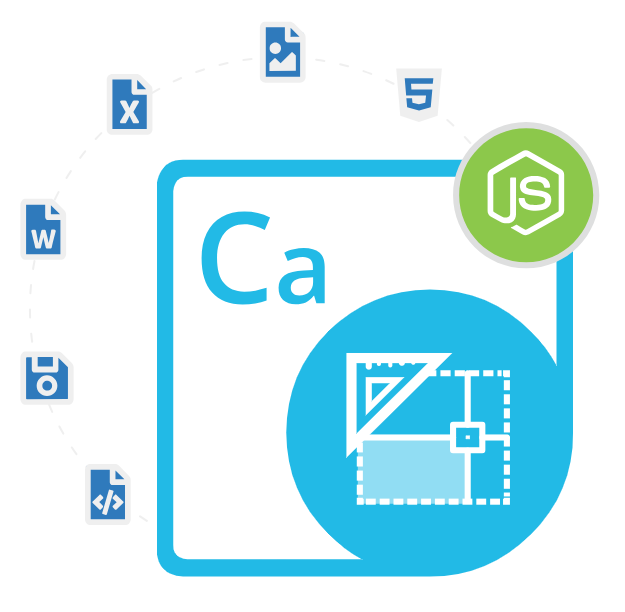
Aspose.CAD Cloud Node.js SDK
Free Node.js SDK to Create & Convert AutoCAD Drawings
Open Source Node.js API for Creating, Editing, Reading, and Exporting AutoCAD DWG, DXF, DWF, DXB & STL drawing to PDF & Raster Images (BMP, GIF, JPG & JPEG) inside Node.js Environment.
What is Aspose.CAD Cloud Node.js SDK?
Aspose.CAD Cloud Node.js SDK is designed for developers aiming to integrate CAD file processing features into their Node.js apps. If you work with intricate architectural blueprints, engineering designs, or technical drawings, this toolkit streamlines the management of diverse CAD formats. By handling all the complexities related to CAD file processing, this SDK provides a user-friendly API for performing various tasks such as converting, exporting or rendering files inside Node.js applications. Software developers that need a complete solution for building CAD and BIM file processing into their cross-platform applications find it even more an interesting choice.
The Aspose.CAD Cloud Node.js SDK is versatile. It can handle various standard CAD file types like DWG (AutoCAD Drawing), DXF (Drawing Exchange Format), DWF, IFC, STL, and even Elite CAD files used in reverse engineering. This broad support allows developers to work with popular CAD formats without needing multiple tools or libraries. In this library, we have covered key features, such as creating new diagrams from scratch, flip-rotate a CAD image, convert (save)CAD drawings to other file formats, get or set image properties of CAD drawing, modify scale of AutoCAD file, copy, moves or delete CAD files from cloud storage etc.
The Aspose.CAD Cloud Node.js SDK is a handy tool that makes handling CAD files in a Node.js setting a breeze. By tapping into cloud computing capabilities, it streamlines tasks, even those that require a lot of resources, so you can get things done smoothly. This also takes away the hassle of managing bulky local systems for processing CAD files. So, if you’re creating an online CAD viewer, an automated converter, or personalized reporting software, this SDK has got you covered with all the features you need.
Getting Started with Aspose.CAD Cloud Node.js SDK
The recommend way to install Aspose.CAD Cloud Node.js SDK is using NPM. Please use the following command for a smooth installation.
Install Aspose.CAD Cloud Node.js SDK via NPM
npm install aspose-cad
You can download the library directly from Aspose.CAD Cloud Node.js SDK product page
Create & Manipulate CAD Files inside Node.js
Aspose.CAD Cloud Node.js SDK makes it easy for software developers to programmatically create and manipulate CAD files inside their Node.js applications. The SDK supports a wide array of CAD file formats, ensuring that developers can create and work with the most commonly used types like DWG, DXF, and DWF, as well as lesser-known formats. Software developers can perform various manipulations on CAD files, including rotating, scaling, and flipping drawings. This feature enables the creation of customized views or adjustments without altering the original file. The following example shows, how software developer can load and modify an existing CAD file inside Node.js applications.
How to Load, Modify and Save an Existing CAD Drawing inside Node.js Apps?
const layoutOptions = new cadApi.LayoutOptions({
layoutName: "Model",
scaleFactor: 2.0 // Scale the layout by 2x
});
const layoutRequest = new cadApi.PostDrawingSaveAsRequest({
name: "basic.dxf",
format: "pdf",
outPath: "output/basic_scaled.pdf",
options: layoutOptions
});
// Modify and save the layout with scaling
cad.postDrawingSaveAs(layoutRequest).then(() => {
console.log("Layout modified and saved as PDF with scaling.");
});
Convert AutoCAD DWG to PDF in Node.js
One of the most powerful features of Aspose.CAD Cloud Node.js SDK is its ability to convert AutoCAD DWG, DWF, and DXF CAD drawings to PDF and image formats such as BMP, PNG, JPG, JPEG, TIF, TIFF, PSD, GIF, and several others formats. This feature is particularly useful for creating accessible versions of CAD drawings that can be easily viewed or shared. Here is an example that demonstrates, how software developers can convert AutoCAD DWG to PDF using Node.js library.
How to Convert AutoCAD DWG to PDF in Node.js Environment?
const cadApi = require("asposecadcloud");
// Initialize the CAD API
const cad = new cadApi.CadApi("Your Client ID", "Your Client Secret");
const conversionRequest = new cadApi.PostDrawingSaveAsRequest({
name: "sample.dwg",
format: "pdf",
outPath: "output/sample.pdf"
});
// Convert the DWG file to PDF
cad.postDrawingSaveAs(conversionRequest).then(() => {
console.log("DWG file converted to PDF successfully.");
});
CAD File Viewer Creation
One practical application of the Aspose.CAD Cloud Node.js SDK is creating a web-based CAD file viewer. By utilizing the rendering capabilities of the SDK, software developers can build a platform where users can upload CAD files and view them directly in the browser without needing specialized software. The viewer can include features like zooming, panning, and layer management, providing an intuitive experience for users.
Export Specific Layers of CAD Files in Node.js
Software developers often need to work with specific layers, layouts, or entities within a CAD file. Aspose.CAD Cloud Node.js SDK allows users to export these elements separately, making it easy to extract the exact information needed for further processing or presentation. Here is an example that shows how software developers can load and export specific layers of AutoCAD drawing into PNG image inside Node.js applications.
How to Export Specific Layer of CAD Drawing into PNG inside Node.js Apps?
const cadApi = require("asposecadcloud");
// Initialize the CAD API
const cad = new cadApi.CadApi("Your Client ID", "Your Client Secret");
const exportRequest = new cadApi.GetDrawingPropertiesRequest({
name: "sample.dwg",
folder: "input"
});
// Get CAD drawing properties to identify layers
cad.getDrawingProperties(exportRequest).then((properties) => {
const layerName = properties.layers[0].layerName;
// Export the first layer to PNG
const saveAsRequest = new cadApi.PostDrawingSaveAsRequest({
name: "sample.dwg",
format: "png",
outPath: `output/${layerName}.png`,
options: {
layerNames: [layerName]
}
});
cad.postDrawingSaveAs(saveAsRequest).then(() => {
console.log(`Layer '${layerName}' exported as PNG successfully.`);
});
});