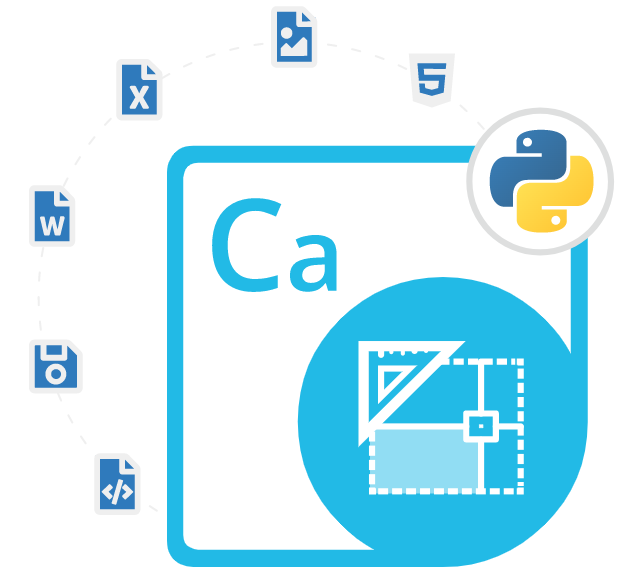
Aspose.CAD Cloud SDK for Python
Python REST API to Generate & Manipulate CAD Drawings
A Powerful Python API that fully support useful features for creating, editing, reading, manipulating and converting AutoCAD DWG, DXF DWF and BIM Files in the cloud.
What is Aspose.CAD Cloud Python SDK?
Aspose.CAD Cloud Python SDK is an extremely helpful software development kit (SDK) that enables programmers to design robust apps for working with AutoCAD and BIM drawings. With the help of the robust REST API, developers may convert CAD drawings to a number of file types, including PDF, SVG, BMP, PNG, JPEG, and more. This API is wrapped in the Aspose.CAD Cloud Python SDK, which makes it simple for programmers to include various features into their Python apps.
Software developers can incorporate CAD file conversion and manipulation features into their Python-based apps with the help of the dependable Aspose.CAD Cloud Python SDK. It enables software developers to transfer their CAD drawings from one format to another and offers incredibly strong conversion capabilities to convert their CAD drawings from one format to another such as DWG, DWF, and DXF to PDF and images formats such as BMP, PNG, JPG, JPEG, JPEG2000, TIF, TIFF, PSD, GIF and so on. Aspose.CAD Cloud Python SDK can be used on any platform that supports Python, including Windows, Linux, and macOS.
The Aspose.CAD Cloud Python SDK offers an affordable way to work with CAD files. It offers a scalable and adaptable cloud-based platform and does away with the need for pricey CAD software and gear. The SDK for working with CAD files has many key features, including the ability to edit CAD files programmatically, add or modify CAD entities, add or remove layers or layouts, render CAD files to different image formats (PNG, JPG, BMP, TIFF), support for CAD file validation, integration with cloud storage, and many more. All things considered, it is the ideal choice for programmers who must manage CAD files in their Python-based apps.
Getting Started with Aspose.CAD Cloud Python SDK
Aspose.CAD Cloud Python SDK can be installed using pip, the Python package manager. To install it, simply run the following command.
Install Aspose.CAD Cloud Python SDK via pip
pip install asposecadcloud
You can also download it directly from Aspose product page.Convert CAD Drawings to Image via Python API
Aspose.CAD Cloud SDK for Python has included complete support for converting CAD drawings to several other supported image file formats in the cloud. The library has included support for CAD Drawings conversion to BMP, PNG, JPG, JPEG, JPEG2000, TIF, TIFF, PSD, GIF and WMF file formats. The Software developers can also export a selected layers and layouts from the CAD drawings. The following example demonstrates how to export existing images to another format using Python REST API.
Export Part of the Image via Python API
Aspose.CAD Cloud SDK for Python enables software developers to export a part of a CAD image to other supported file formats using Python commands. To achieve the task first you need to load image and get information about the image using the get_drawing_properties method. After that you need to get the dimensions of the image area that you want to export. You will need to pass the file name and folder name of the image, as well as the coordinates of the top-left and bottom-right corners of the area. Now the image is ready to export, please pass the file name and folder name of the image, as well as the format that you want to export the image to. The following code is provided for exporting part of the image to other supported file formats.
How to Export Part of the Image via Python API?
import asposecadcloud
from asposecadcloud.apis.cad_api import CadApi
# Your Aspose Cloud credentials
client_id = 'your_client_id'
client_secret = 'your_client_secret'
base_url = 'https://api.aspose.cloud'
# Create an instance of the ApiClient class
configuration = asposecadcloud.Configuration()
configuration.api_key['ClientId'] = client_id
configuration.api_key['ClientSecret'] = client_secret
configuration.host = base_url
api_client = asposecadcloud.ApiClient(configuration)
# Create an instance of the CadApi class
cad_api = CadApi(api_client)
# Get the properties of the drawing
filename = 'sample.dwg'
folder_name = 'CAD'
drawing_properties = cad_api.get_drawing_properties(filename, folder=folder_name)
# Get the dimensions of the area to export
top_left_x = 0
top_left_y = 0
bottom_right_x = 100
bottom_right_y = 100
drawing_area = cad_api.get_drawing_area(filename, folder=folder_name, x=top_left_x, y=top_left_y, width=bottom_right_x-top_left_x, height=bottom_right_y-top_left_y)
# Export the area as a PNG image
export_format = 'png'
output_filename = 'output.png'
export_options = asposecadcloud.PngOptions()
export_options.area = drawing_area
cad_api.get_drawing_save_as(filename, export_format, folder=folder_name, out_path=output_filename, export_options=export_options)
Manage CAD Drawing Size via Python API
Aspose.CAD Cloud SDK for Python has included powerful support for handling CAD drawings and their sizes. The library provided support for Automatic size adjustment or Adjust size by using the UnitType enumeration. To resize an image first you need to load an existing image and get the current size of the drawing and after that create an instance of the new export options. After that set the new size of the drawing and convert the drawing to the new format with the new size. Now save it to the specified output file path. The following example shows how to achieve this.
How to Resize CAD Drawing via Python API?
import asposecadcloud
from asposecadcloud.apis.cad_api import CadApi
from asposecadcloud.models.cad_bmp_export_options import CadBmpExportOptions
# set up the API client
cad_api = CadApi(api_key, app_sid, api_base_url)
# specify the input and output file paths
input_file = "input.dwg"
output_file = "output.dwg"
# get the current size of the drawing
size = cad_api.get_drawing_properties(input_file).drawing_size
# create an instance of the BMP export options
bmp_export_options = CadBmpExportOptions()
# set the new size of the drawing
bmp_export_options.width = size.width * 2
bmp_export_options.height = size.height * 2
# convert the drawing to BMP format with the new size
cad_api.put_drawing_bmp(input_file, bmp_export_options, output_file)
Rotate and Flip CAD Images via Python API
Aspose.CAD Cloud SDK for Python has included some useful features for image manipulation and conversion to other support file format using Python REST API. To rotate and flip an existing image first you need to set the input and output file name and format. After that you need to set rotation and flip properties and then rotate or flip the drawing. Now you can download the rotated and flipped drawing and save it in the place of your choice.
How to Rotate and Flip CAD Images inside Python Applications?
import asposecadcloud
from asposecadcloud.apis.cad_api import CadApi
from asposecadcloud.models.requests import PutDrawingRotateFlipRequest
from asposecadcloud.models.rotate_flip_type import RotateFlipType
# Configure API key authorization
configuration = asposecadcloud.Configuration()
configuration.api_key['api_key'] = 'YOUR_APP_KEY'
configuration.api_key['app_sid'] = 'YOUR_APP_SID'
# Create a CAD API instance
cad_api = CadApi(asposecadcloud.ApiClient(configuration))
# Set input file name and format
filename = 'input.dwg'
format = 'dwg'
# Set output file name and format
output_filename = 'output.dwg'
output_format = 'dwg'
# Set rotation and flip properties
rotate_flip_type = RotateFlipType.FlipX
# Rotate and flip the drawing
request = PutDrawingRotateFlipRequest(filename, rotate_flip_type, output_format, folder=None, storage=None, output_path=output_filename)
response = cad_api.put_drawing_rotate_flip(request)
# Download the rotated and flipped drawing
download_request = asposecadcloud.DownloadFileRequest(output_filename)
download_response = cad_api.download_file(download_request)