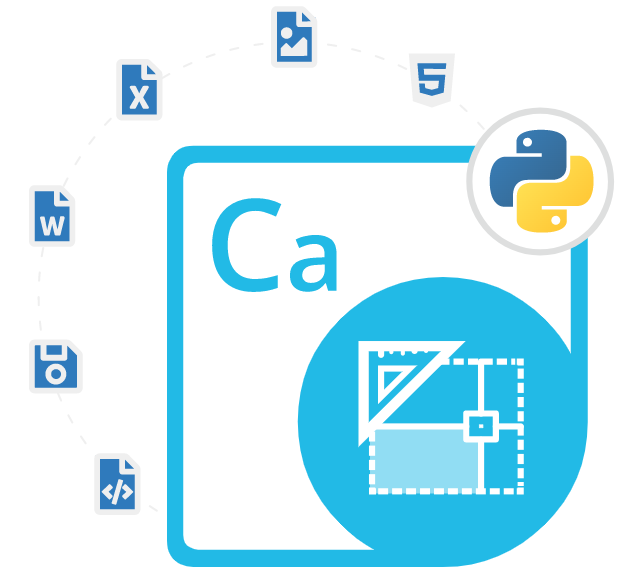
Aspose.CAD for Python via .NET
Python API for CAD Drawings Generation & Conversion
A Powerful Python AutoCAD API that Fully Support Advanced Features for Generating, Editing, Reading, Manipulating and Converting CAD File Formats.
In today's fast-paced world, graphical content is at the forefront of communication, whether it's engineering drawings, architectural designs, or intricate technical diagrams. To efficiently handle and manipulate such graphical data in your Python projects, you can rely on the powerful and versatile tool called Aspose.CAD for Python via .NET . It provides support for various CAD file formats and allows for efficient conversion, rendering, editing, and manipulation of CAD files. It is commonly associated with the .NET platform, it can also be integrated into Python applications through .NET.
Aspose.CAD for Python via .NET an advanced, robust and flexible API that empowers software professionals to create, modify, read, and convert Computer-Aided Design (CAD) drawings in various supported file formats. It supports popular CAD file formats such as DWG, DXF, DWF, and DGN, allowing software developers to work with CAD data efficiently. Whether you need to extract information from CAD drawings or generate CAD files programmatically, Aspose.CAD provides the tools and features you need to get the job done. Make sure you have Python and IronPython installed on your system. By combining Python, IronPython, and .NET, you can build cross-platform applications that can run on various operating systems.
Aspose.CAD for Python via .NET is optimized for high-performance processing, making it suitable for large and complex CAD files. It has an active community of developers and provides extensive documentation, tutorials, and customer support. This ensures that you can get help when you need it and access a wealth of resources to aid your development. Whether you're building CAD viewers, converters, or editors, Aspose.CAD provides the flexibility and features you need to succeed in your projects. So, go ahead and explore the potential of the API to streamline your CAD-related development tasks.
Getting Started with Aspose.CAD for Python via .NET
Aspose.CAD for Python via .NET can be installed using pip, the Python package manager. To install it, simply run the following command
Install Aspose.CAD for Python via .NET via pip
pip install aspose-cad
You can also download it directly from Aspose product page.Convert CAD Drawings to Image via Python API
Aspose.CAD Cloud SDK for Python has included complete support for converting CAD drawings to several other supported image file formats in the cloud. The library has included support for CAD Drawings conversion to BMP, PNG, JPG, JPEG, JPEG2000, TIF, TIFF, PSD, GIF and WMF file formats. The Software developers can also export a selected layers and layouts from the CAD drawings. The following example demonstrates how to export existing images to another format using Python REST API.
Generate Visio Diagrams via Python API
Aspose.CAD for Python via .NET has included complete support for creating and manipulating Visio diagrams programmatically without using Microsoft Visio. The API enables software developers to manage CAD diagram’s shapes and their properties with ease. It supports layout shapes feature to automatically position shapes faster as compare to the manual way. Using Aspose.Diagram, you can create new Visio diagrams from scratch or load existing ones, add and manipulate shapes, text, and other elements, and save the modified diagrams to disk or stream. Here's a simplified example of creating a Visio diagram using Aspose.CAD and IronPython.
How to Create Visio Diagram inside Python Applications?
import clr
clr.AddReference("Microsoft.Office.Interop.Visio")
from Microsoft.Office.Interop.Visio import *
# Create a new instance of Visio application
visio_app = ApplicationClass()
# Create a new document
doc = visio_app.Documents.Add("")
# Add shapes to the document
page = doc.Pages.Add()
shape1 = page.DrawRectangle(1, 1, 3, 2)
shape2 = page.DrawRectangle(5, 5, 7, 6)
# Connect the shapes
connector = page.Drop(page.Application.ConnectorToolDataObject, shape1, shape2)
# Save the document
doc.SaveAs("MyDiagram.vsdx")
# Close the document
doc.Close()
AutoCAD Drawings Conversion via Python API
Aspose.CAD for Python via .NET makes it easy for software developers to load and convert existing CAD drawing to various other supported file formats inside Python applications. It supports a wide range of CAD file formats, making it a versatile solution for developers. You can convert between various formats such as DXF, DWG, DWT, DGN, IFC, DWF, DWFX, STL, IGES, CF2, Collada(DAE), PLT, OBJ, SVG, DXB, FBX, U3D, 3DS, STP and so on. Developers can extract specific elements, and even export CAD data to image formats with just a couple of lines of code. The following code example shows how software developers can convert AutoCAD DWG format to PDF file inside Python applications.
Convert AutoCAD DWG Drawing to PDF via Python API
import aspose.cad as cad;
cadImage = cad.Image.load("file.dwg");
rasterizationOptions = cad.imageoptions.CadRasterizationOptions()
rasterizationOptions.page_width = 1200
rasterizationOptions.page_height = 1200
pdfOptions = cad.imageoptions.PdfOptions()
pdfOptions.vector_rasterization_options = rasterizationOptions
cadImage.save("result.pdf", pdfOptions)
CAD Drawings Rendering via Python API
Aspose.CAD for Python via .NET provides the ability to load CAD drawings in various formats like DWG, DXF, DWF, or DGN and render it into images. Software developers can render CAD drawings to images, PDFs, or other formats. This is beneficial for generating high-quality previews and reports. In the following example, Aspose.CAD is used to render a CAD drawing (in this case, a DWG file) into a PNG image. You can customize the rendering options, such as page size, resolution, and image format, according to your requirements.
How to Render CAD DWG Drawing to PNG Image using Python Code?
import asposecad
from asposecad.image import Image
from asposecad.imageoptions import CadRasterizationOptions, PdfDocumentOptions, PdfCompliance
# Set your Aspose.CAD license
asposecad.license.set_license("path/to/your/license.lic")
# Load the CAD file
cad_image = Image.load("path/to/your/cadfile.dwg")
# Specify rendering options
rasterization_options = CadRasterizationOptions()
rasterization_options.page_width = 800 # Set the width of the output image
rasterization_options.page_height = 600 # Set the height of the output image
# Render the CAD drawing
cad_image.save("path/to/output/rendered_image.png", rasterization_options)
# You can also render to PDF if desired
pdf_options = PdfDocumentOptions()
pdf_options.compliance = PdfCompliance.PdfA1b
cad_image.save("path/to/output/rendered_image.pdf", pdf_options)
Extract Information from CAD Drawings via Python
Extracting metadata and other information from CAD files is an important activity while working with AutoCAD drawing. Aspose.CAD for Python via .NET has provided complete support for load an existing CAD drawing and extract information from it using Python applications. You can access various features and functions to achieve your specific goals. It supports getting details about layers, entities, and properties.