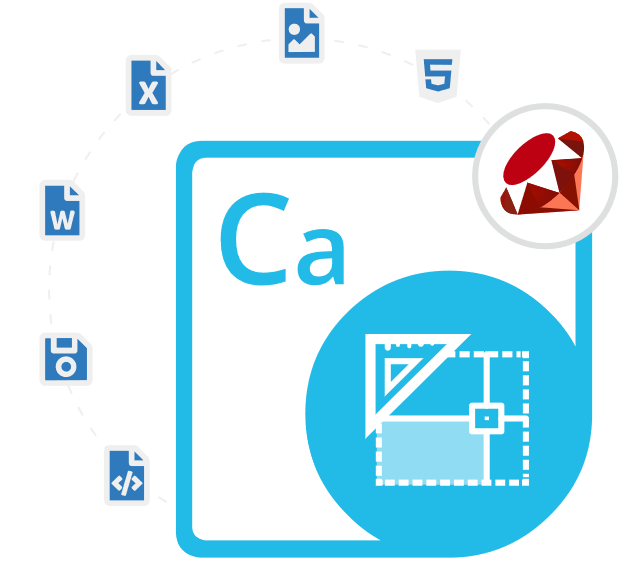
Aspose.CAD Cloud SDK for Ruby
Ruby REST API to Generate & Export CAD Drawings
Advanced Ruby SDK enables Software Professionals to Read, Write, Open, Update and Convert AutoCAD DWG, DWF drawings to PDF or Images in the Cloud.
What is Aspose.CAD Cloud SDK for Ruby?
Aspose.CAD Cloud SDK for Ruby is a fantastic tool for Ruby developers. It helps you handle different AutoCAD file types in the cloud. CAD files are commonly used in industries like architecture, engineering, and manufacturing. Dealing with CAD files can be tough because they are usually intricate and unique. Also, CAD software can be costly and demanding on your computer’s resources. With just a couple of lines of code you can edit existing CAD files, add or delete layers, adjust colors, change shapes, optimize CAD files for better performance, convert CAD drawings to raster images, and more.
Aspose.CAD Cloud SDK for Ruby offers an easy and handy method to handle CAD files in the cloud. This SDK allows developers to do various tasks on CAD files. You can convert them to PDF, DWG, DXF, DWF, and other formats. The library offers a straightforward API that software developers can use to handle various CAD file tasks in the cloud. The API is well explained and can be easily added to your current applications. It fully backs up major CAD file formats like DWG, STL, DWT, DGN, IGS, PLT, IFC, DXF, and more. Developers can also change CAD files to various formats like PDF, DWG, DXF, DWF, and others. Overall it’s a great choice for working with CAD files in the cloud. Using the Ruby Cloud SDK, software developers can easily convert CAD files to different formats, modify and manipulate CAD drawings, and perform various operations on CAD files.
Getting Started with Aspose.CAD Cloud SDK for Ruby
The recommend way to install Aspose.CAD Cloud SDK for Ruby is using RubyGem. To work with. Run the following command to add the Aspose.CAD Cloud SDK for Ruby to your project.
Install Aspose.CAD Cloud SDK for Ruby via RubyGem
gem install aspose_cad_cloud
You can also download it directly from Aspose product page.CAD Drawings Export to Raster Image via Ruby
Aspose.CAD Cloud SDK for Ruby has included various useful features for exporting CAD drawings to other support file formats using Ruby code. Software can also convert AutoCAD files to raster images with just a couple of lines of Ruby code. Raster images such as JPEG, PNG, TIFF, and BMP are some of the widely used file formats. The SDK makes it easy for software developers to export CAD Drawings to BMP, PNG, JPG, JPEG, JPEG2000, TIF, TIFF, PSD, GIF, WMF & many more. The following example shows how to convert a CAD file to a raster image using Ruby commands.
How to Convert a CAD File to a Raster Image via Ruby API?
require 'aspose_cad_cloud'
# create an instance of the CadApi class
cad_api = AsposeCadCloud::CadApi.new
# convert a CAD file to a raster image
input_file = 'input.dwg'
output_format = 'png'
output_file = 'output.png'
cad_api.convert_to_raster_image(input_file, output_format, output_file)
# convert a specific page of a CAD file to a raster image
page_number = 2
cad_api.convert_to_raster_image(
input_file,
output_format,
output_file,
{
dpi: 300, # set the DPI to 300
page_index: page_number # convert only the specified page
}
)
Get CAD Image Properties via Ruby API
Aspose.CAD Cloud SDK for Ruby provides the capability to get properties of an image and use it according to your needs inside Ruby applications. The library enables software developers to Retrieve CAD drawing properties such as image width, image height, color palette, image size, image bounds, get current unit type, image container, image title and many more. The following example demonstrates how software developers can get CAD image properties inside their own Ruby applications.
How to Get CAD Image Properties via Ruby Commands?
def test_get_properties_drawing_tests
filename = '910609.dxf'
remote_name = filename
dest_name = remote_test_out + remote_name
st_request = PutCreateRequest.new remote_test_folder + remote_name, File.open(local_test_folder + filename, "r").read
@storage_api.put_create st_request
request = GetDrawingPropertiesRequest.new remote_name, remote_test_folder
result = @Cad_api.get_drawing_properties_with_http_info request
assert_equal 200, result[1]
end
Resize, Flip or Rotate CAD Image via Ruby API
Aspose.CAD Cloud SDK for Ruby has included complete support for image manipulation and gives software developers the power to rotate or flip an existing AutoCAD drawing inside their own cloud applications. It supports Rotating images at 180FlipNone, Rotate180FlipX, Rotate180FlipXY, Rotate180FlipY, Rotate270FlipNone, Rotate90FlipX, Rotate90FlipXY, and several others. It is also possible to adjust the size of drawing images according to their own needs using Ruby commands. The following example demonstrates how to change image scale from the body using Ruby code.
How to Change CAD Image Size using Ruby API?
def test_post_drawing_scale
filename = '01.026.385.01.0.I SOPORTE ENFRIADOR.dwg'
remote_name = filename
output_format = 'pdf'
dest_name = remote_test_out + remote_name + '.' + output_format
st_request = PutCreateRequest.new remote_test_folder + remote_name, File.open(local_test_folder + filename, "r").read
@storage_api.put_create st_request
request = PostDrawingResizeRequest.new File.open(local_test_folder + filename, "r"), output_format, 320, 240, remote_test_folder
result = @Cad_api.post_drawing_resize_with_http_info request
assert_equal 200, result[1]
end