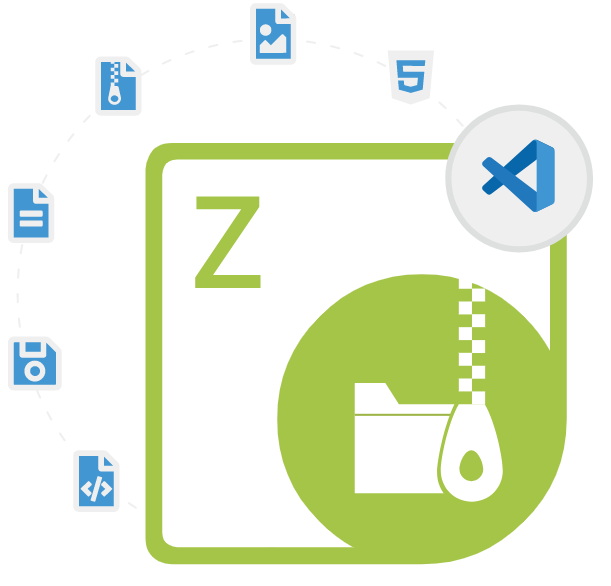
Aspose.ZIP for .NET
C# .NET API to Compress & Decompress ZIP, RAR Files
.NET Compression API allows to compress and decompress files, folders, directories and password protect the ZIP archives.
Aspose.ZIP for .NET is a very powerful document compression and archive management API that enables software developers to create a variety of applications for handling the compression/decompression of files and folders with lesser time and effort. The library makes it easy for developers to create, extract, modify, and manipulate ZIP archives in a variety of archive formats, such as ZIP, 7Zip, RAR, TAR, GZIP, BZ2, GZIP, LZ, CPIO, XZ, Z, CAB and many more.
The library is very feature rich and has included several important features for archiving and extracting files and folders, such as creating an archive from one or multiple files, inserting files into an existing archive, compressing directories to preserve its structure, deleting any unwanted entry from the archive, store files to archives without compression, decompress files or folders, encryption of 7z archives, apply Encryption to archives, encrypt specific entries with password in an archive, and many more. The library can be easily integrated with any kind of application, from ASP.NET web applications to forms applications within any OS.
Aspose.ZIP for .NET makes it easy for developers to create and manage encrypted archives. The library supports encryption algorithms such as AES 128, AES 192, and AES 256, and provides a number of options for controlling the encryption and decryption process. This makes it ideal for use in applications that require secure storage and transfer of sensitive data. The library also supports working with large archives using streams. This means that developers can easily read and write data from and to an archive without having to load the entire archive into memory.
Getting Started with Aspose.ZIP for .NET
The recommend way to install Aspose.ZIP for .NET is using NuGet. Please use the following command for a smooth installation.
Install Aspose.Pdf via NuGet Command
NuGet\Install-Package Aspose.Zip -Version 23.1.0
You can download the library directly from Aspose.ZIP product page
Compress & Extract Archive via .NET API
Aspose.ZIP for .NET gives software developers the capability to compress or extract archives inside their own .NET applications. The library has provided support for some commonly archive format such as ZIP, RAR, 7Zip, GZIP, BZ2 and numerous Linux format such as CPIO, TAR, Lzip, Bzip2, XZ and Z. The library has provided support for several basic and advanced features, such as ZIP files with password, extract a particular file from archive, Unzip Password Protected Zip, apply encryption and decryption, and so on.
Compressing Multiple Files using C#
using (FileStream zipFile = File.Open(dataDir + "CompressMultipleFiles_out.zip", FileMode.Create))
{
using (FileStream source1 = File.Open(dataDir + "alice29.txt", FileMode.Open, FileAccess.Read))
{
using (FileStream source2 = File.Open(dataDir + "asyoulik.txt", FileMode.Open, FileAccess.Read))
{
using (var archive = new Archive())
{
archive.CreateEntry("alice29.txt", source1);
archive.CreateEntry("asyoulik.txt", source2);
archive.Save(zipFile, new ArchiveSaveOptions() { Encoding = Encoding.ASCII, ArchiveComment = "There are two poems from Canterbury corpus" });
}
}
}
}
Create Password Protected Archive via C#
Aspose.ZIP for .NET enables software developers to compress and decompress files or folders with password protection inside their own C# .NET applications without worrying about the underlying file structure. The library has included several important features related to archive protection and encryption, such as encryption of files with AES192, encryption of files with AES256, password protect directory, encrypt multiple files with mixed encryption techniques, decompress AES encrypted archives, decompress AES encrypted stored archive, decompress encrypted folder to directory, decompress archive having single file, decompress archive having multiple files, extract stored archive without compression.
Encrypt Multiple Files with Mixed Encryption Techniques via C#.NET API?
/using (FileStream zipFile = File.Open(dataDir + "CompressWithTraditionalEncryption_out.zip", FileMode.Create))
{
using (FileStream source1 = File.Open(dataDir + "alice29.txt", FileMode.Open, FileAccess.Read))
{
var archive = new Archive(new ArchiveEntrySettings(null, new TraditionalEncryptionSettings("p@s$")));
archive.CreateEntry("alice29.txt", source1);
archive.Save(zipFile);
}
}
Create self-extracting (SFX) Archive via C#
Aspose.ZIP for .NET has provided software developers the capability for creating and managing a self-extracting (SFX) archive inside their own .NET applications. A self-extracting (SFX) archive is an executable file with an embedded archive that can be extracted automatically after launch. The library has included several important features for handling self-extracting archive such as creating a self-extracting archive instantiate, set password, running self-extracting archive (requires .NET Framework 2.0 or higher) and so on. The library also provided some command line options for self-extracting archive.
Compose a Self-extracting Archive via C# API
using (FileStream zipFile = File.Open("archive.exe", FileMode.Create))
{
using (var archive = new Archive())
{
archive.CreateEntry("entry.bin", "data.bin");
var sfxOptions = new SelfExtractorOptions()
{
ExtractorTitle = "Extractor",
CloseWindowOnExtraction = true,
TitleIcon = "C:\pictorgam.ico"
};
archive.Save(zipFile, new ArchiveSaveOptions() { SelfExtractorOptions = sfxOptions });
}
}
Convert ZIP Archive to Tar.gz, 7z & RAR via .NET API
Aspose.ZIP for .NET enables software developers to convert ZIP Archive to many other file formats with ease such as Tar.gz, 7z, RAR and so on. A tar.gz file is a combination of a .tar file and a .gz file. It is considered one of the most widespread compressed archive formats in the Linux world and on the other hand ZIP is the most popular for Windows. Aspose.ZIP for .NET has provided complete support for extract entries from the ZIP archive and directly put them to the tar.gz archive with just a couple of lines of code. Below examples shows how to achieve this.
Extract Entries from the ZIP Archive via .NET API
using (Archive source = new Archive("source.zip"))
{
using (TarArchive tar = new TarArchive())
{
foreach (ArchiveEntry entry in source.Entries)
{
if (!entry.IsDirectory)
{
MemoryStream mem = new MemoryStream();
entry.Open().CopyTo(mem);
tar.CreateEntry(entry.Name, mem);
}
}
tar.SaveGzipped("result.tar.gz");
}
}