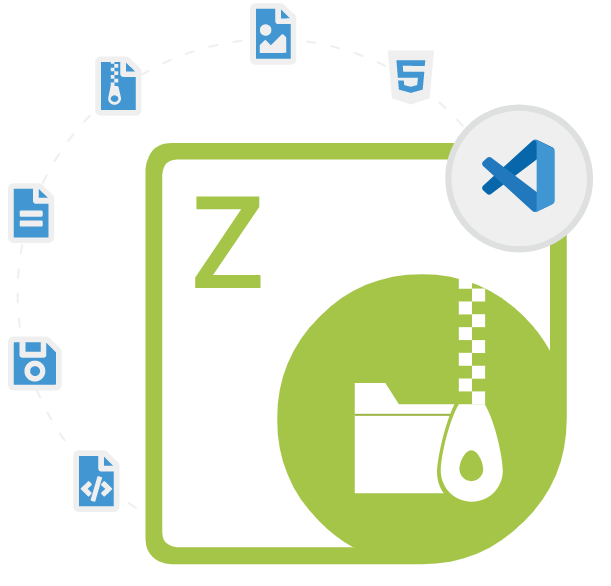
Aspose.ZIP for Python via .NET
Python API to Create & Manage ZIP, RAR & TAR Archives
Python Compression API to Create Archives or Decompress Archives of types ZIP, 7Zip, RAR, TAR, GZIP, BZ2, LZ, CPIO, XZ, Z, CAB in Python Apps.
Data compression plays a pivotal role in today's digital landscape, enabling efficient storage and transmission of files. Aspose.ZIP for Python via .NET Library is a versatile toolkit that empowers software developers to harness the power of data compression and archive management within Python applications through seamless integration with .NET. The library enables software developers to create, edit, manipulate, and manage various types of compressed archives such as ZIP, 7Zip, RAR, TAR, GZIP, BZ2, GZIP, LZ, CPIO, XZ, Z, CAB, and many more.
Its integration with Python through .NET bridges the gap between these two programming worlds, offering a powerful toolset to compress, decompress, and manage archive files within Python applications. This integration allows software developers to utilize the capabilities of the .NET framework while programming in Python. There are several important features part of the library, such as compressing multiple files, decompressing files or folders, extracting compressed files from these archives, applying encryption to compressed archives, applying password protection to archives, adding/deleting files to existing archives, tracking compression process, and many more.
Aspose.ZIP for Python via .NET supports various compression algorithms, allowing Software developers to choose the most suitable one for their specific use case. This flexibility ensures optimal compression ratios and performance. Its integration with Python through the .NET framework brings the power of this library to Python developers, offering a wide range of functionalities for working with compressed files. Its robust features, ease of integration, and extensive documentation make it a valuable tool for optimizing data storage and transmission. By incorporating this library, software developers can enhance their applications with efficient compression and extraction capabilities, ultimately delivering a better user experience.
Getting Started with Aspose.ZIP for Python via .NET
The recommend way to install Aspose.ZIP for Python via .NET is using pypi.org. Please use the following command for a smooth installation.
Install Aspose.Pdf via NuGet Command
pip install aspose-zip
You can download the library directly from Aspose.ZIP product page
Compression and Decompression via Python API
Aspose.ZIP for Python via .NET enables software engineers to compress as well as decompress various types to files inside their Python applications. The library allows software developers to compress multiple files and folders into various archive formats such as ZIP, 7Zip, RAR, TAR, GZIP, BZ2, GZIP, LZ, CPIO, XZ, and may more. Similarly, it provides the capability to extract compressed files from these archives. This feature is particularly useful when dealing with large sets of files that need to be shared or stored efficiently. The following example shows how software developers can create a compressed ZIP archive with just a couple of lines of Python code.
Create a Compressed ZIP Archive using Python
import clr
clr.AddReference("Aspose.Zip")
from Aspose.Zip import Archive, ArchiveUpdateAction, CompressionLevel
# Create an instance of Archive
archive = Archive()
# Add files and folders to the archive
archive.CreateEntry("file.txt")
archive.CreateEntry("folder/")
# Set compression level
archive.CompressionLevel = CompressionLevel.Normal
# Save the archive
archive.Save("example.zip")
Encryption and Password Protection Support
Security is paramount when dealing with sensitive data. Aspose.ZIP for Python via .NET makes it easy for software engineers to apply encryption or password protection while compressing or decompressing various types of files and folders inside their Python applications, ensuring that only authorized individuals can access the data within them. The library supports encrypting as well as decrypting whole archive or specific entries inside an archive. It supports various archive encryption technique such as ZipCrypto, AES encryption and various others.
Archive Manipulation Support via Python API
Aspose.ZIP for Python via .NET is a very useful API that allows software developers to create and manage various types of archives using Python commands. The library offers methods for adding, updating, and deleting files within existing archives without having to extract and re-compress the entire archive. This not only saves time but also reduces the risk of data loss.
Create Self-extracting Compressed Archives via Python
Aspose.ZIP for Python via .NET has provided a very useful features for creating and managing self-extracting compressed archives using Python. A self-extracting (SFX) archive is an executable file with an embedded archive that can be extracted automatically after launch. It creates a self-extracting ZIP archive with just a couple lines of code and supports features like adding files to it, setting password, running self-extracting archive and many more. The following code demonstrates how to use the Aspose.ZIP library to create a self-extracting archive with specific properties, such as the title and the default extraction location.
How to Create a Self-Extracting Archive via Python API?
import clr
clr.AddReference("Aspose.Zip")
from Aspose.Zip import ZipFile, CompressionLevel, SelfExtractorFlavor
def create_self_extracting_archive(source_directory, output_path):
with ZipFile() as zip:
# Add files from the source directory to the ZIP archive
zip.AddDirectory(source_directory)
# Set the SelfExtractorFlavor to create a self-extracting archive
zip.SelfExtractorFlavor = SelfExtractorFlavor.Exe
# Set optional self-extractor properties
zip.SelfExtractorExeOptions.Title = "Self-Extracting Archive"
zip.SelfExtractorExeOptions.DefaultExtractLocation = "Extracted_Files"
# Save the self-extracting archive
zip.SaveSelfExtractor(output_path, "password123") # Set a password if desired
# Usage
create_self_extracting_archive("source_directory", "self_extracting_archive.exe")