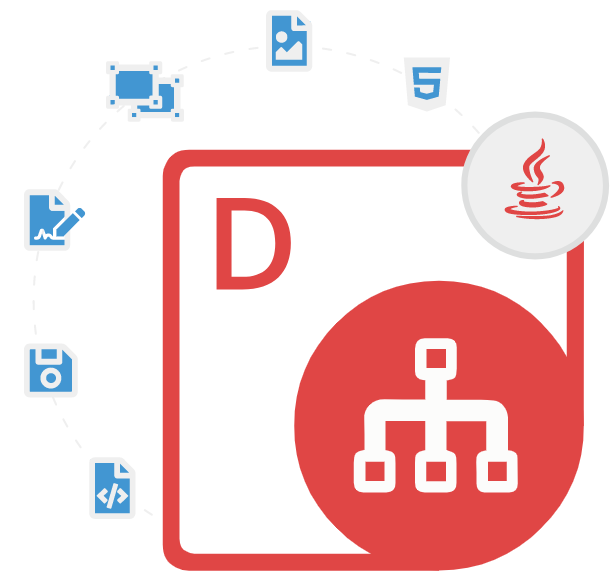
Aspose.Diagram for Java
Java API to Generate & Convert Visio Diagrams
Microsoft Visio document processing API to Generate, modify, manipulate, & convert Visio Diagrams to PDF, HTML, XPS & images file formats.
Aspose.Diagram for Java is a comprehensive diagramming library that gives software developers the capability to generate and manage Microsoft Visio Diagrams without installing Microsoft Office Visio dependencies. By using the Java diagramming library, developers can work with Visio diagrams in a programmatic way, making it easier to Print Visio diagrams with high fidelity, protect diagrams, access and read the properties, integrate with other systems, and manipulate Visio diagrams in a variety of ways.
Aspose.Diagram for Java enables software programmers to create new Visio diagrams from scratch or modify existing ones by adding or removing shapes, lines, text, and other elements. This helps developers in automating the important tasks of diagram creation, updating diagrams with new data, or changing the appearance of diagrams inside their own Java applications. There are some other important tasks also part of the library such as adding a hyperlink to a shape, grouping multiple shapes, inserting comments to drawings, parsing Visio diagrams, and many more.
Aspose.Diagram for Java is the ability to convert Visio diagrams to other formats. With Aspose.Diagram for Java, developers can convert Visio diagrams to PDF, XPS, HTML, JPEG, PNG, BMP, TIFF, SVG, EMF, XAML, and many other popular formats with just a couple of lines of Java code. The conversion process is very smooth and also makes it possible to share Visio diagrams with people who may not have access to MS Visio, or to integrate Visio diagrams into other systems. The library is designed to perform well on both the server as well as client side. Whether you need to create new diagrams, modify existing ones, or automate tasks, Aspose.Diagram for Java has you covered. With its powerful features and easy-to-use API, Aspose.Diagram for Java is the perfect tool for any Java developer who needs to work with Visio diagrams.
Getting Started with Aspose.Diagram for Java
The recommend way to install Aspose.Diagram for Java is via Maven repository. You can easily use Aspose.Diagram for Java API directly in your Maven Projects with simple configurations.
Maven repository for Aspose.Diagram for Java
//First you need to specify Aspose Repository configuration / location in your Maven pom.xml as follows:
<repositories>
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://releases.aspose.com/java/repo/</url>
</repository>
</repositories>
//Then define Aspose.Diagram for Java API dependency in your pom.xml as follows:
<dependencies>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-diagram</artifactId>
<version>19.9</version>
<classifier>jdk16</classifier>
</dependency>
</dependencies>
You can download the library directly from Aspose.Diagram product page
Visio Diagrams Generation via Java API
Aspose.Diagram for Java has included complete supports for generating Microsoft Visio diagrams inside Java applications without Microsoft Office Automation. To create a new diagram from the scratch you need to create a new Visio documents and add shapes as well as connectors to build up the diagram. The library supports various Layout approaches helping developers to quickly and smoothly create the diagrams. The library fully support working with VBA projects and allows developers to modify their VBA module code automatically with just a couple of lines of java code. Developers can also easily retrieve Visio connectors and font information. The library also gives users the control to stop conversion or loading of diagrams using InterruptMonitor when it is taking too long.
Create New Visio Drawing via Java API
string dataDir = RunExamples.GetDataDir_LoadSaveConvert();
// Initialize a Diagram class
Diagram diagram = new Diagram();
// Save diagram in the VSDX format
diagram.Save(dataDir + "CreateNewVisio_out.vsdx", SaveFileFormat.VSDX);
Visio Diagrams Conversion inside Java Apps
Aspose.Diagram for Java has included complete support for loading and converting Microsoft Visio diagrams to a wide range of file formats, including PDF, XPS HTML, EMF, SWF, XAML, JPEG, PNG, BMP, TIFF, SVG, EMF, and many more. The conversion process is simple and straightforward, and you can use the same code to convert multiple diagrams in batch mode. The library also supports the conversion of other file formats to Visio diagrams. You can easily control the appearance and behavior of the converted diagrams. The library supports setting options such as page size, margins, and more, to ensure that your converted diagrams look exactly the way you want them to.
Export Visio Drawing to PDF via Java Library
String dataDir = Utils.getDataDir(ExportToPDF.class);
// Call the diagram constructor to load diagram from a VSD file
Diagram diagram = new Diagram(dataDir + "ExportToPDF.vsd");
// Save as PDF file format
diagram.save(dataDir + "ExportToPDF_Out.pdf", SaveFileFormat.PDF);
Work with Pages in Visio Diagrams via Java API/h2>
Pages are the building blocks of a Visio diagram and are used to organize the shapes, lines, and other elements that make up a diagram. Aspose.Diagram for Java library enables software developers to easily create new pages, access existing pages, and manipulate the elements on a page. It allows accessing the shapes and elements on a page, and modifying their properties, such as size, position, and appearance. You can also add new shapes and elements to a page, and delete existing ones. It is also very easy to access and work with layers. The library allows users to easily create new layers, access existing layers, and modify the properties of layers, such as visibility and printing behavior.
How to Get a Page Object by Name from Visio Drawing via Java API
String dataDir = Utils.getDataDir(GetVisioPagebyName.class);
// Call the diagram constructor to load diagram from a VSDX file
Diagram diagram = new Diagram(dataDir + "Drawing1.vsdx");
// Set page name
String pageName = "Flow 2";
// Get page object by name
Page page2 = diagram.getPages().getPage(pageName);
Manage Masters in Visio Diagrams via Java API
Aspose.Diagram for Java makes it easy for software developers to work with masters and retrieve information like ID and names of masters inside Java applications. Masters are pre-designed shapes that can be reused multiple times in a Visio diagram. By using masters, you can ensure that your diagrams are consistent and standardized, which can make it easier to maintain and update your diagrams over time. The library allows creating new masters, modify existing ones, or delete masters that you no longer need. It is also possible to access the shapes and elements within a master, which makes it possible to manipulate the master's appearance and behavior. The library also allows controlling the behavior of the shapes in a master, such as how they respond to events such as resizing, rotating, and moving.
Get a Master from the Visio File via Java API
String dataDir = Utils.getDataDir(GetMasterbyID.class);
// Call the diagram constructor to load diagram from a VDX file
Diagram diagram = new Diagram(dataDir + "RetrieveMasterInfo.vdx");
// Set master id
int masterid = 2;
// Get master object by id
Master master = diagram.getMasters().getMaster(masterid);
System.out.println("Master ID : " + master.getID());
System.out.println("Master Name : " + master.getName());
System.out.println("Master Name : " + master.getUniqueID());