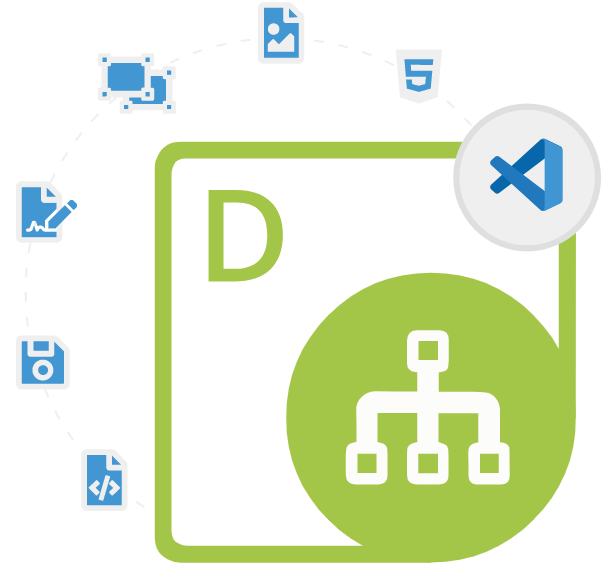
Aspose.Diagram for .NET
C# .NET API to Create, Edit & Convert Visio Diagrams
A Powerful Visio Diagraming API to create, edit, manipulate, & convert Visio files to XPS, HTML, SVG, SWF, XAML, images or PDF formats.
Aspose.Diagram for .NET is a very useful Visio Diagramming library that gives software developers the ability to create, modify, manipulate, and convert Microsoft Visio Diagrams inside C# applications. The library provides a rich set of functionalities for working with shapes and pages within Microsoft Visio diagrams. It allows adding, deleting, and modifying shapes and shapes’ properties, such as size, position, and formatting. The library is designed to perform well on both a server and a client.
Aspose.Diagram for .NET provides very useful features for handling page properties such as page size, orientation, and margins, and manages the visibility and order of layers and sections within a Visio diagram. It also supports retrieving Visio connectors and Font Information, Merging different diagrams, Inserting or copying a new page, formatting Visio Page, managing page size, extracting Images, adding a hyperlink to a Visio shape, adding a watermark to an Image, raw data processing, replacing a picture shape, create fields, add or retrieve or copy Visio Shape Data and many more.
There are various useful benefits part of Aspose.Diagram for .NET such as its ability to read, write, and convert Microsoft Visio diagrams in a variety of formats such VSDX, VSX, VTX, VDX, VSSX, VSTX, VSDM, VSSM, VSD, VSS, VST and VDW. This enables developers to easily work with Visio diagrams in a format that best suits their needs, whether it be for collaboration, analysis, or presentation. In addition to its core functionality, the library also provides a range of advanced features, such as support for macros and plugins, and the ability to perform calculations using built-in functions. This allows developers to create complex and sophisticated Visio diagrams and perform data analysis as well as generate reports with ease.
Getting Started with Aspose.Diagram for .NET
The recommend way to install Aspose.Diagram for .NET is using NuGet. Please use the following command for a smooth installation.
Install Aspose.Diagram for .NET via NuGet
Install-Package Aspose.Pdf
You can also download it directly from Aspose product page.Create Visio Diagrams via C# .NET API
Aspose.Diagram for .NET has included complete support for creating and manipulating Visio diagrams programmatically without using Microsoft Visio. The library allows users to handle diagram’s shapes and their properties with ease. It supports layout shapes feature to automatically position shapes faster as compare to the manual way. The library also supports working with VBA module code automatically and allows users to extract and modify VBA module code. Using Aspose.Diagram, you can create new Visio diagrams from scratch or load existing ones, add and manipulate shapes, text, and other elements, and save the modified diagrams to disk or stream.
Create New Diagram from the Scratch via .NET API
string dataDir = RunExamples.GetDataDir_LoadSaveConvert();
// Initialize a Diagram class
Diagram diagram = new Diagram();
// Save diagram in the VSDX format
diagram.Save(dataDir + "CreateNewVisio_out.vsdx", SaveFileFormat.VSDX);
Convert Visio Diagrams to Other File Formats via C# API
Aspose.Diagram for .NET is convenient and efficient solution enables software developers to open and convert Microsoft Visio diagrams to various file formats inside their own .NET applications. The library supports Visio diagrams conversion to some popular file formats such as PDF, XPS HTML, EMF, SWF, XAML, JPEG, PNG, BMP, TIFF, SVG, EMF, and many more. The conversion process is fast and efficient, and the output file is accurate and of high quality. The library supports some important features, such as splitting pages, converting Visio drawing with selective shapes, modifying existing shapes, and so on.
Export Microsoft Visio Drawing to PDF via C# API
string dataDir = RunExamples.GetDataDir_LoadSaveConvert();
// Call the diagram constructor to load a VSD diagram
Diagram diagram = new Diagram(dataDir + "ExportToPDF.vsd");
MemoryStream pdfStream = new MemoryStream();
// Save diagram
diagram.Save(pdfStream, SaveFileFormat.PDF);
// Create a PDF file
FileStream pdfFileStream = new FileStream(dataDir + "ExportToPDF_out.pdf", FileMode.Create, FileAccess.Write);
pdfStream.WriteTo(pdfFileStream);
pdfFileStream.Close();
pdfStream.Close();
// Display Status.
System.Console.WriteLine("Conversion from vsd to pdf performed successfully.");
Add & Manage Shapes in Visio Files via .NETAPI
Aspose.Diagram for .NET allows software developers to insert shapes into Visio diagrams and manage its properties with ease. The library supports several important features for handling Visio shapes, such as add new shape, retrieve and modify existing shape, convert Visio shape to HTML or image, copy existing shape, connect shapes with each other, manage Shapes Paragraph, Shapes Gluing support, Visio TimeLine Shapes, calculate Pin Values, setting Size of a shape, apply theme to shape, group, convert and verify shapes and many more.
Retrieve Shape Information from Visio Diagram via C# API
string dataDir = RunExamples.GetDataDir_Shapes();
// Load diagram
Diagram vsdDiagram = new Diagram(dataDir + "RetrieveShapeInfo.vsd");
foreach (Aspose.Diagram.Shape shape in vsdDiagram.Pages[0].Shapes)
{
// Display information about the shapes
Console.WriteLine("\nShape ID : " + shape.ID);
Console.WriteLine("Name : " + shape.Name);
Console.WriteLine("Master Shape : " + shape.Master.Name);
}
Insert & Edit Text in Visio Diagrams via .NET API
Aspose.Diagram for .NET allows software developers to working with Visio in different ways inside Visio Diagrams using .NET library. The C# library has include different features for handling text in shapes, such as inserting text shape, customize text shape in the Visio diagram, update the shape’s text, find and replace the shape’s text, apply Built-in or custom style-sheet to text, apply different style on the each text value of a shape, extract plain Text from the Visio diagram page and many more.
Adds Text Shape in the Visio Diagram via .NET API
string dataDir = RunExamples.GetDataDir_ShapeText();
// Create a new diagram
Diagram diagram = new Diagram();
// Set parameters and add text to a Visio page
double PinX = 1, PinY = 1, Width = 1, Height = 1;
diagram.Pages[0].AddText(PinX, PinY, Width, Height, "Test text");
// Save diagram
diagram.Save(dataDir + "InsertTextShape_out.vsdx", SaveFileFormat.VSDX);