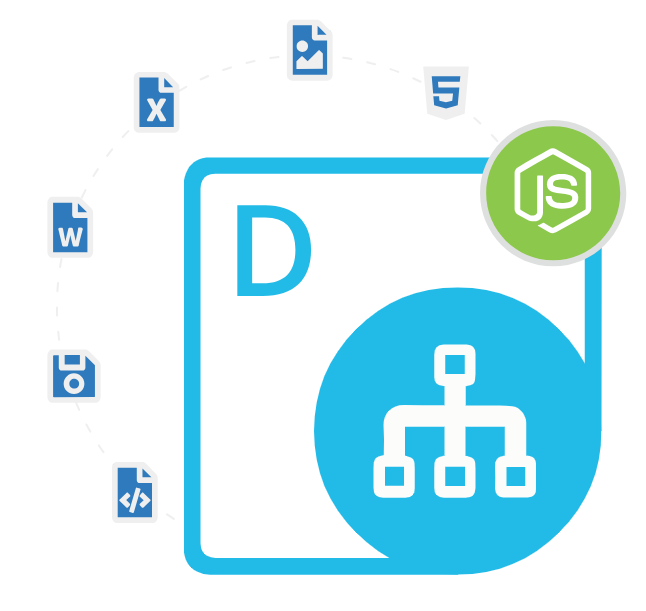
Aspose.Diagram Cloud SDK for Node.js
Node.js REST API to Generate & Convert Visio Diagrams
A Powerful Node.js Library That allows to Develop Cloud-based Applications for Creating, Editing, Manipulating and Converting Microsoft Visio VSDX, VDX & VSX Diagrams via JavaScript REST API.
What is Aspose.Diagram SDK for Node.js?
Lots of organizations use diagrams to show complicated data and processes. Microsoft Visio is a popular tool for making and sharing these diagrams. However, it’s been tough to work with these files programmatically, especially in the cloud. As the need for cloud-based solutions keeps rising, the Aspose.Diagram SDK for Node.js steps in to help software developers create apps focused on diagrams. . It simplifies working with Visio file formats like VSD, VSDX, and many more, allowing businesses to handle their diagram workflows efficiently. There are several important features part of the library, such as creating Visio diagrams from scratch, conversion of Visio files to various formats, modifying existing diagrams, extracting diagrams data, version control features support, customizing shapes and many more.
The Aspose.Diagram Cloud SDK for Node.js offers a complete tool for managing Microsoft Visio diagram files in the cloud. It enables developers to handle tasks like creating, reading, editing, and converting Visio diagrams without requiring Microsoft Visio on the server. This SDK streamlines the process of working with different diagram formats like VSDX, VDX, VSDM, VSSX, VSSM, VSTX, VSTM, VTX, VDX, and more, all within Node.js applications. Creating applications involves more than just making diagrams. It’s about setting the groundwork for building advanced and scalable applications. By automating diagram creation and integrating smoothly with various cloud services, developers can craft solutions that streamline processes, boost productivity, and enhance data representation. This software development kit (SDK) makes working with Visio diagrams easier by offering a user-friendly API, strong cloud support, and a wide array of diagram functions. It simplifies the process of working with Visio diagrams in any environment.
Getting Started with Aspose.Diagram Cloud SDK for Node.js
The recommend way to install Aspose.Diagram Cloud SDK for Node.js is using NPM. Please use the following command for a smooth installation.
Install Aspose.Diagram Cloud SDK for Node.js via npm
npm install aspose.diagram
You can download the library directly from Aspose.Diagram product page
Create Visio Diagrams in Node.js Programmatically
Aspose.Diagram Cloud SDK for Node.js has provided complete support for creating Visio diagrams from scratch inside Node.js applications. This capability is useful for applications that need to dynamically generate diagrams based on user input, data processing, or automation scripts. For example, imagine an application that creates organizational charts based on user data input. The SDK allows developers to programmatically add shapes, connectors, and define their properties such as position, size, and rotation. Here is an example that shows, how software developers can create a nee diagram inside Node.js applications.
How to Create a Visio Diagram inside Node.js Apps?
var aspose = aspose || {};
aspose.diagram = require("aspose.diagram");
var diagram = new aspose.diagram.Diagram();
diagram.save("output.vsdx", aspose.diagram.SaveFileFormat.VSDX);
Visio Diagram Conversion in Node.js
In many scenarios, diagrams need to be shared in different formats. The Aspose.Diagram Cloud SDK for Node.js supports conversion of Visio files to various other supported file formats such as PDF, SVG, PNG, GIF, JPEG and many more. This feature allows developers to build applications where users can upload a diagram and download it in a preferred format. The following example shows how easily software developers can convert an existing Visio diagram into PNG file formats inside Node.js.
How to Export Visio Diagram into PNG Image inside Node.js Apps?
const diagram = new Diagram('myDiagram.vsdx');
diagram.exportTo('myDiagram.png', 'png');
Visio Diagram Data Extracting in Node.js
In some cases, software developers may need to analyze diagram structures and extract detailed information from a diagram. Aspose.Diagram Cloud SDK for Node.js provides APIs to programmatically retrieve shape data, page information, layer details, and other elements from existing Visio diagrams inside Node.js application. This is useful for creating reports or integrating diagrams with other business systems. For example, an application could extract shapes and connectors from a workflow diagram to generate a textual summary of the process. The following example demonstrates how software developers can Extract Shape Data inside Node.js applications.
How to Extract Shape Data from Visio Diagram inside Node.js Environment?
const getShapeInfo = async () => {
try {
const shapeInfo = await diagramApi.getShape("diagram.vsdx", "Page-1", 1);
console.log("Shape info:", shapeInfo);
} catch (error) {
console.error("Error retrieving shape info:", error);
}
};
getShapeInfo();
Manipulate Visio Diagrams in Node.js Apps?
Aspose.Diagram Cloud SDK for Node.js allows software developers to load and manipulate existing diagrams with ease inside Node.js environment. You can add, remove, or edit shapes and their properties. Additionally, users can manipulate diagram pages, layers, connectors, and formatting properties directly through the SDK. Here is a simple example that shows how programmers can add a shape to a diagram inside Node.js applications.
How to Add a Shape to a Diagram via Node.js Library?
const addShape = async () => {
try {
const shapeData = {
shapeId: 1,
pageName: "Page-1",
x: 2,
y: 2,
width: 2,
height: 2
};
const response = await diagramApi.addShape("sample.vsdx", shapeData);
console.log(response);
} catch (error) {
console.error("Error adding shape:", error);
}
};
addShape();