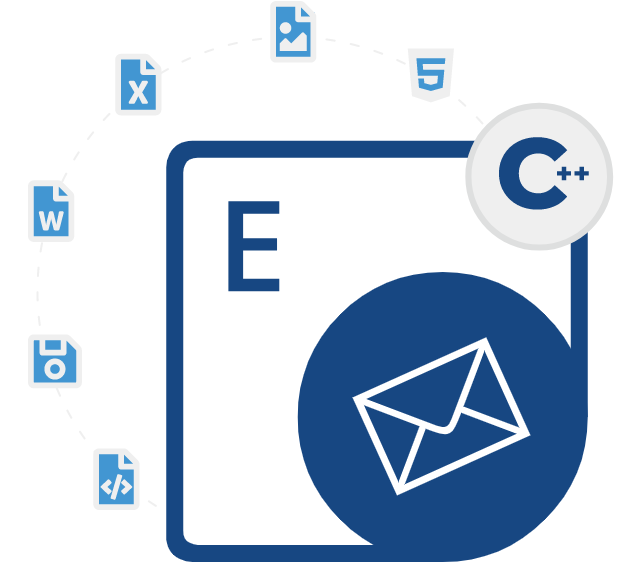
Aspose.Email for C++
C++ API for MS Outlook Email Processing
A Comprehensive C++ Outlook Email Generation, Reading, Editing and Management API. It supports MSG, MHT, EML, EMLX, and Other Email File Formats.
What is Aspose.Email for C++?
Aspose.Email for C++ is a comprehensive outlook Email management API that enables software developers to create their own applications for working with Microsoft Outlook Email file formats. It supports creating and sending email messages with different file formats such as Outlook MSG, MHT, EML, EMLX, and more. It supports parsing and manipulating email formats, making it easy to extract and manipulate email message data such as subject, body, recipient, sender, and other related information.
Aspose.Email for C++ provides complete support for working with popular email servers such as Exchange Server, IMAP, and POP3. With this support, developers can easily send and receive email messages, manage folders and messages on email servers, and more. Moreover, the library provides full support for the Exchange Web Services (EWS) API, making it ideal for use in Exchange-based applications. The library is written in the native C language and can be used on both Windows as well as Linux platforms.
Aspose.Email for C++ has provided simple and flexible methods for handling email attachments. It helps developers to add, access, and remove attachments from email messages. It supports a wide range of attachment file formats, including popular formats such as PDF, Excel, Word, and more. There are several other important features part of the library, such as generating emails via mail merge from different types of data sources, email addresses verification, embedding objects like images & sounds in email messages, handling Outlook media types such as messages, tasks, contacts, calendar and Journal items, parsing emails in MSG, MHT, and EML formats, Extract attachments from Outlook MSG and many more.
Getting Started with Aspose.Email for C++
The recommend way to install Aspose.Email for C++ is using NuGet. Please use the following command for a smooth installation.
Install Aspose.Email for C++ via NuGet
NuGet\Install-Package Aspose.Email.Cpp -Version 23.1.0
You can also download it directly from Aspose product page.Generate Email Messages via C++ API
Aspose.Email for C++ has included complete functionality for email message creation as well as sending it to different recipients inside C++ applications. The library supports all basic email properties like From, To, Subject and body for the newly created mail message. There are other important features also part of the library, such as associate a friendly name with an email address, add attachments, embed other objects, extracting contents from the MIME messages, and many more. The library supports creating and sending email messages in Outlook MSG, MHT, EML, EMLX and many mother file formats.
How to Generate Email Messages via C++ API?
// The path to the File directory.
System::String dataDir = RunExamples::GetDataDir_Email();
// Create a new instance of MailMessage class
System::SharedPtr message = System::MakeObject();
// Set subject of the message, Html body and sender information
message->set_Subject(L"New message created by Aspose.Email for C++");
message->set_HtmlBody(System::String(L"This line is in bold.
") + L"This line is in blue color");
message->set_From(System::MakeObject(L"from@domain.com", L"Sender Name", false));
// Add TO recipients and Add CC recipients
message->get_To()->Add(System::MakeObject(L"to1@domain.com", L"Recipient 1", false));
message->get_To()->Add(System::MakeObject(L"to2@domain.com", L"Recipient 2", false));
message->get_CC()->Add(System::MakeObject(L"cc1@domain.com", L"Recipient 3", false));
message->get_CC()->Add(System::MakeObject(L"cc2@domain.com", L"Recipient 4", false));
// Save message in EML, EMLX, MSG and MHTML formats
message->Save(dataDir + L"CreateNewMailMessage_out.eml", SaveOptions::get_DefaultEml());
message->Save(dataDir + L"CreateNewMailMessage_out.emlx", SaveOptions::CreateSaveOptions(MailMessageSaveType::get_EmlxFormat()));
message->Save(dataDir + L"CreateNewMailMessage_out.msg", SaveOptions::get_DefaultMsgUnicode());
message->Save(dataDir + L"CreateNewMailMessage_out.mhtml", SaveOptions::get_DefaultMhtml());
Convert Email Messages Format via C++ API
Aspose.Email for C++ enables software developers to load email messages of different format like EML, HTML, MHTML, MSG and Data etc. You can also load email messages with custom options and save it in various other file formats. The library has provided several functions for loading email messages from a disk and saves them back in other formats, such as saving email to EML format, saving EML to MSG saving as EML preserving TNEF attachments, EML to HTML and MHTML conversion, exporting email message to MHT and so on.
How to Convert EML Messages to MSG via C++ API?
// Create and initialize an instance of the Appointment class
Appointment appointment = new Appointment(
"Meeting Room 3 at Office Headquarters",// Location
"Monthly Meeting", // Summary
"Please confirm your availability.", // Description
new DateTime(2015, 2, 8, 13, 0, 0), // Start date
new DateTime(2015, 2, 8, 14, 0, 0), // End date
"from@domain.com", // Organizer
"attendees@domain.com"); // Attendees
// Save the appointment to disk in ICS format
appointment.Save(dstEmail, AppointmentSaveFormat.Ics);
Console.WriteLine("Appointment created and saved to disk successfully.");
Manage Email Attachments & Embeded Objects via C++ API
An Email attachment is a very useful and an easy way of sharing files, photos, videos and other computer generated file via email. Aspose.Email for C++ has included complete functionality for sending additional files as attachment with email messages. Please remember that the library allows sending any number of attachments but the size of the attachment is limited by the mail server. There are numerous features supported by the library for handling attachments, such as add attachments, removing attachments, display attachment file name, extract email attachment, read attachment’s Content-Description and so on. Same like attachment it is also possible to embed objects inside an email message using Aspose.Email library.
How to Add Attachments to an Email Message via C++ API?
// The path to the File directory.
System::String dataDir = RunExamples::GetDataDir_Email();
// Create an instance of MailMessage class
System::SharedPtr message = System::MakeObject();
message->set_From(L"sender@sender.com");
message->get_To()->Add(L"receiver@gmail.com");
// Load an attachment
System::SharedPtr attachment = System::MakeObject(dataDir + L"1.txt");
// Add Multiple Attachment in instance of MailMessage class and Save message to disk
message->get_Attachments()->Add(attachment);
message->AddAttachment(System::MakeObject(dataDir + L"1.jpg"));
message->AddAttachment(System::MakeObject(dataDir + L"1.doc"));
message->AddAttachment(System::MakeObject(dataDir + L"1.rar"));
message->AddAttachment(System::MakeObject(dataDir + L"1.pdf"));
message->Save(dataDir + L"outputAttachments_out.msg", SaveOptions::get_DefaultMsgUnicode());
Working with Outlook Calendar Items via C++ API
Aspose.Email for C++ has provided complete functionality for working with email messages as well as other Outlook elements, including the calendar items. It enables developers to programmatically create, read, modify, and save Outlook Calendar items in ICS format. The library also supports working with MapiCalendar and allows users to save the calendar item as MSG file format. There are numerous features part of the library, such as adding display reminder to a calendar, setting audio reminder to a calendar, adding and retrieving attachments from calendar files, checking status of recipients from a meeting request, setting a reminder by adding tags and many more.
How to Create & Save Calendar Item in ICS Format via C++ API?
// The path to the File directory.
System::String dataDir = RunExamples::GetDataDir_Outlook();
// Create the appointment
System::SharedPtr calendar = System::MakeObject(L"LAKE ARGYLE WA 6743", L"Appointment", L"This is a very important meeting :)", System::DateTime(2012, 10, 2, 13, 0, 0), System::DateTime(2012, 10, 2, 14, 0, 0));
calendar->Save(dataDir + L"CalendarItem_out.ics", Aspose::Email::Mail::AppointmentSaveFormat::Ics);