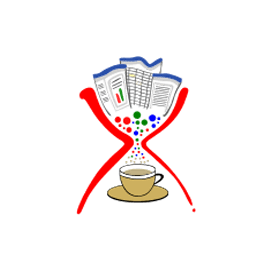
Apache POI HSMF
Process MSG Files via Open Source Java API
Read Microsoft Outlook MSG files to access Render Information, Subject & Body contents or Extract Attachments with Apache POI.
If you are a Java developer looking for an email processing library to process email messages, you may want to consider Apache POI-HSMF. It is the POI Project's pure Java implementation of the Outlook MSG format, providing low-level read access to MSG files along with a user-facing way to get at the common textual content of MSG files such as sender, subject, message body and more. Developers can get message headers information, save email messages, read fixed Size properties from MSG file, extract embedded message properties, Working with message encoding and much more.
Getting Started with Apache POI HSMF
First of all, you need to have the Java Development Kit (JDK) installed on your system. If you already have it then proceed to the Apache POI's download page to get the latest stable release in an archive. Extract the contents of the ZIP file in any directory from where the required libraries can be linked to your Java program. That is all!
Referencing Apache POI in your Maven-based Java project is even simpler. All you need is to add the following dependency in your pom.xml and let your IDE fetch and reference the Apache POI Jar files.
Apache POI Maven Dependency
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.0</version>
</dependency>
API to Access Outlook MSG Files
Apache POI-HSMF allows Java developers and programmers to access the contents of Outlook MSG files. Apache POI-HSMF is a port of the Microsoft Outlook message file format to pure Java. The API is at the very basic level as of now and, therefore, limited functionality is available for working with email messages. Developers can get
Extract Data from MSG - Java
// Open MSG file
MAPIMessage msg = new MAPIMessage("sample.msg");
// Read Content
System.out.println("From: " + msg.getDisplayFrom());
System.out.println("To: " + msg.getDisplayTo());
System.out.println("CC: " + msg.getDisplayCC());
System.out.println("BCC: " + msg.getDisplayBCC());
System.out.println("Subject: " + msg.getSubject());
Read & Extracts Attachments from Outlook MSG File
Apache POI-HSMF API enables Java developers to parse Outlook MSG files; extract and read the contents of the embedded document. Developers can access attachments of MAPI messages. It supports reading one or several Outlook MSG files and for each of them creates a text file from available chunks and a directory that contains attachments. It reads attachments from the Outlook MSG file and writes it to disk as an individual file.
Extract Attachments for MSG - Java
// Open MSG file
MAPIMessage msg = new MAPIMessage("sample.msg");
// Extract Attachment
AttachmentChunks[] attachments = msg.getAttachmentFiles();
if(attachments.length > 0) {
File d = new File("D:\\Attachments");
if(d.mkdir()) {
for(AttachmentChunks attachment : attachments) {
processAttachment(attachment, d);
}
}
}
Save Email Message Contents inside Java Apps
Java programmers can use Apache POI-HSMF API to extract and save email message content. The email body can be extracted to create a new file, and you can then write it to disc with the help of FileWrite.
Extract Email Body to Create a New File via Java
String filename = "message.msg";
MAPIMessage msg = new MAPIMessage(filename);
PrintWriter txtOut = new PrintWriter("ApacheMessage.txt");
txtOut.println("Email Body: " + msg.getTextBody());
txtOut.close();