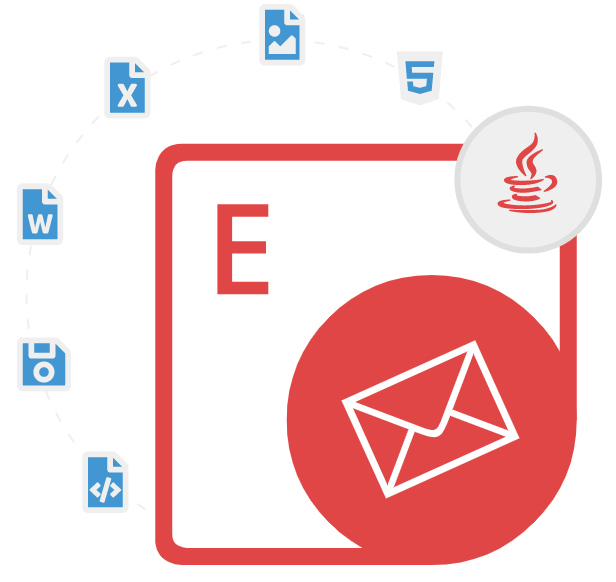
Aspose.Email for Java
Java API to Create & Send Outlook Emails
A Powerful Email Processing API that allows to Create, Manipulate, Analyze, Convert and Transmit Email Messages Without using Microsoft Outlook.
What is Aspose.Email for Java?
Aspose.Email for Java is a handy tool for Java developers. It helps you generate and handle Outlook email messages in your Java applications. With this library, you can manage email file formats like MSG, PST, OST, OFT, EML, EMLX, MBOX, and VCF. This feature comes in handy if you need to switch email data from one format to another or deal with emails in archives.
Creating and editing emails in Java is a breeze with Aspose.Email for Java. You can craft a new message or tweak an existing one, changing details like the sender, recipients, subject, and attachments with just a few lines of Java code. This library also shines when it comes to managing email attachments. It allows developers to seamlessly add, edit, or extract attachments from email messages. This flexibility opens up a world of possibilities for working with emails and their attachments in different ways, such as storing attachments in a database or extracting attachments for processing.
Aspose.Email for Java comes packed with some key features to manage Outlook MSG files effectively. These features include crafting new MSG files from the ground up, modifying existing MSG files, checking MSG content, extracting attachments from MSG files, saving attachments to your computer, and more. Additionally, the software enables you to open and read PST files effortlessly and convert them to MSG format seamlessly. The library provides detailed documentation and examples, making it easy for developers to understand how to use the library and how to integrate it into their projects.
Getting Started with Aspose.Email for Java
The recommend way to install Aspose.Email for Java is via Maven repository. You can easily use Aspose.PDF for Java API directly in your Maven Projects with simple configurations.
Maven repository for Aspose.Pdf for Java
//First, you need to specify the Aspose Maven Repository configuration/location in your Maven pom.xml as follows:
<repositories>
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://releases.aspose.com/java/repo/</url>
</repository>
</repositories>
// For a successful installation of Aspose.Email for Java, First, you need to specify the Aspose Maven Repository configuration/location in your Maven pom.xml as follows:
<dependencies>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-email</artifactId>
<version>22.12</version>
<classifier>jdk16</classifier>
</dependency>
</dependencies>
You can download the library directly from Aspose.Email product page
Email Messages Creation via Java API
Aspose.Email for Java makes it easy for software developers to programmatically create email messages and send it to multiple recipients inside their own Java applications. The library supports adding attachments, and setting the subject, body, sender, and recipient details with ease. You can also specify message date, message priority, message sensitivity and option for delivery notifications. It is also possible to customize email headers, create a signature for the end of the email, and create as well as send a batch of similar email messages using the mail merge feature. With just a little effort you can extract email message contents as well as email headers.
Create New Email Message & Set Properties via Java API
MailMessage message = new MailMessage();
message.setFrom(new MailAddress("sender@gmail.com"));
message.getTo().add("receiver@gmail.com");
message.setSubject("Using MailMessage Features");
// Specify message date
message.setDate(new Date());
// Specify message priority
message.setPriority(MailPriority.High);
// Specify message sensitivity
message.setSensitivity(MailSensitivity.Normal);
// Specify options for delivery notifications
message.setDeliveryNotificationOptions(DeliveryNotificationOptions.OnSuccess);
Outlook Email Message Conversion inside Java Apps
Aspose.Email for Java has included very powerful support for converting Outlook email messages to various formats with ease. The library allows conversion of Outlook email messages to HTML, MHTML, ICS, VCF, TXT, EML, MSG, and more. The MailMessage class is used to load the Outlook message file, and the save method is used to save the message in the desired format with just a few lines of code. The library also supports for detecting file formats, load & save EML messages, saving as EML preserving TNEF attachments, preserving embedded message format, EML to MSG conversion, save MSG with preserved dates, saving MailMessage as MHTML, Rendering Calendar Events, saving Message as Outlook template (.oft) file and many more.
Email Message Conversion to HTML via Java API
MailMessage msg = MailMessage.load(dataDir + "Message.msg");
msg.save(dataDir + "SavingMessageAsHTML_out1.html", SaveOptions.getDefaultHtml());
//or
MailMessage eml = MailMessage.load(dataDir + "test.eml");
HtmlSaveOptions options = SaveOptions.getDefaultHtml();
options.setEmbedResources(false);
options.setHtmlFormatOptions(HtmlFormatOptions.WriteHeader | HtmlFormatOptions.WriteCompleteEmailAddress);
eml.save(dataDir + "SavingMessageAsHTML_out2.html", options);
How to Manage Outlook Storage Files via Java API?
Aspose.Email for Java has provided several functions for working with Outlook Storage Files using Java commands. The library can be used to create a new Outlook PST file and add a subfolder to it with just a couple of lines of code. Another great feature of the library is that developers can read and convert Outlook OST files to PST and vice versa. It is also possible to read to PST file and get information about folders and subfolders. There are several other important features part of the library, such as handling messages, large PST handling, contacts, calendar items, MapiTask, MapiJournal, MapiNote and many more.
Create PST File & Add Folder to It via Java API
// Create new PST
try (PersonalStorage pst = PersonalStorage.create(path, FileFormatVersion.Unicode)) {
// Add new folder "Test"
pst.getRootFolder().addSubFolder("Inbox");
}
Work with Outlook Contacts via Java API
Aspose.Email for Java has included complete supports for working with Outlook contacts (VCards) inside Java applications. The library allows software developers to create, read, update and save contacts to disk with just a couple of lines of code. The library allows supports rendering contact information to MHTML. To achieve this you need to load VCard into MapiContact and then converted to MHTML with the help of MailMessage API.
Load VCard into MapiContact and Convert It to MHTML via Java API
String dataDir = Utils.getSharedDataDir(RenderingContactInformationToMhtml.class) + "outlook/";
//Load VCF Contact and convert to MailMessage for rendering to MHTML
MapiContact contact = MapiContact.fromVCard(dataDir + "ContactsSaqib Razzaq.vcf");
ByteArrayOutputStream os = new ByteArrayOutputStream();
contact.save(os, ContactSaveFormat.Msg);
MapiMessage msg = MapiMessage.fromStream(new ByteArrayInputStream(os.toByteArray()));
MailConversionOptions op = new MailConversionOptions();
MailMessage eml = msg.toMailMessage(op);
//Prepare the MHT format options
MhtSaveOptions mhtSaveOptions = new MhtSaveOptions();
mhtSaveOptions.setCheckBodyContentEncoding(true);
mhtSaveOptions.setPreserveOriginalBoundaries(true);
mhtSaveOptions.setMhtFormatOptions(MhtFormatOptions.RenderVCardInfo | MhtFormatOptions.WriteHeader);
mhtSaveOptions.setRenderedContactFields(ContactFieldsSet.NameInfo | ContactFieldsSet.PersonalInfo | ContactFieldsSet.Telephones | ContactFieldsSet.Events);
eml.save(dataDir + "ContactsSaqib Razzaq_out.mhtml", mhtSaveOptions);
System.out.println("Execution Completed.");