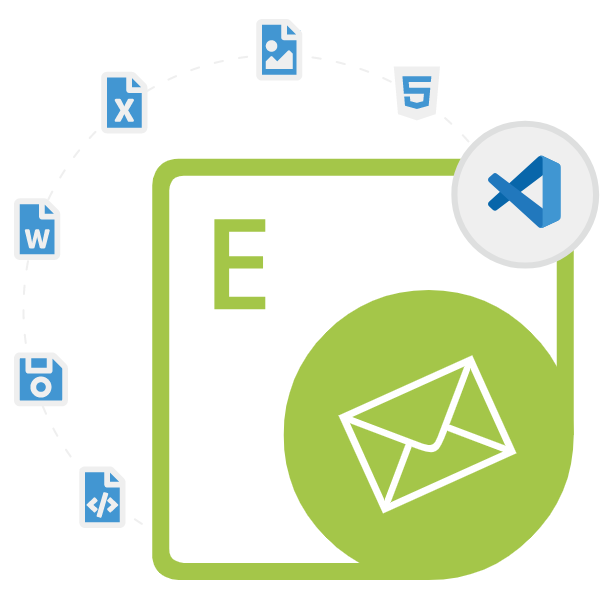
Aspose.Email for .NET
C# .NET API to Process Microsoft Outlook Emails
A Powerful Email Processing API that allows to Create, Manipulate, Analyze, Convert and Transmit Email Messages Without using Microsoft Outlook.
What is Aspose.Email for .NET?
Aspose.Email for .NET is a powerful and easy to use tool for managing emails. It’s like a Swiss Army knife for software developers, letting you handle, read, craft, and transform email messages in different formats like Microsoft Exchange Server, Microsoft Outlook, and IMAP. This library makes it easy and affordable for developers to build all sorts of email apps. Plus, it works seamlessly with both asynchronous and synchronous programming styles.
Aspose.Email for .NET comes packed with handy features to help you load and save email messages in many file formats like MSG, PST, OST, OFT, EML, EMLX, MBOX, ICS, VCF, HTML, MHTML, and more. This makes it simple for you to switch between various email file types and make sure your messages work well with many email programs. The tool also offers security elements such as cram-MD5 authentication, digest-MD5 authentication, AUTH LOGIN authentication, and more to keep your emails safe. The library supports a number of network protocols, such as SMTP, MIME, POP3 and IMAP.
The Aspose.Email for .NET library is a valuable tool for developers working with email messages. It offers various features like creating plain text or HTML emails, adding or removing attachments, embedding objects, working with HTML content, importing and exporting emails, sending emails in batches, saving multiple email messages, template-based mail merging, creating email templates from files, adding iCalendar events to email messages, customize email headers, setting message priority, date or time, and so on. This library can be a great asset for your email-related tasks.
Getting Started with Aspose.Email for .NET
The recommend way to install Aspose.Email for .NET is using NuGet. Please use the following command for a smooth installation.
Install Aspose.Email for .NET via NuGet
NuGet\Install-Package Aspose.Email -Version 22.12.0
You can also download it directly from Aspose product page.Create & Send Email Messages via .NET API
Aspose.Email for .NET has included support for creating and manipulating email message inside C# .NET applications. The library allows creating simple email message with properties like From, To, Subject and body etc. Another great feature that library offers, is that changing email addresses to human-friendly names in an email message. Developers can easily construct email messages that can be transmitted to an SMTP server for delivery with ease. You can easily specify the email body encoding in ANSI, ASCII, Unicode, BigEndianUnicode,UTF-7, UTF-8 and send email message to single or multiple recipients.
How to Create New Email via .NET API?
string dataDir = RunExamples.GetDataDir_Email();
// Create a new instance of MailMessage class
MailMessage message = new MailMessage();
// Set subject of the message, Html body and sender information
message.Subject = "New message created by Aspose.Email for .NET";
message.HtmlBody = "This line is in bold.
" + "This line is in blue color";
message.From = new MailAddress("from@domain.com", "Sender Name", false);
// Add TO recipients and Add CC recipients
message.To.Add(new MailAddress("to1@domain.com", "Recipient 1", false));
message.To.Add(new MailAddress("to2@domain.com", "Recipient 2", false));
message.CC.Add(new MailAddress("cc1@domain.com", "Recipient 3", false));
message.CC.Add(new MailAddress("cc2@domain.com", "Recipient 4", false));
// Save message in EML, EMLX, MSG and MHTML formats
message.Save(dataDir + "CreateNewMailMessage_out.eml", SaveOptions.DefaultEml);
message.Save(dataDir + "CreateNewMailMessage_out.emlx", SaveOptions.CreateSaveOptions(MailMessageSaveType.EmlxFormat));
message.Save(dataDir + "CreateNewMailMessage_out.msg", SaveOptions.DefaultMsgUnicode);
message.Save(dataDir + "CreateNewMailMessage_out.mhtml", SaveOptions.DefaultMhtml);
Create & Manage Appointment via .NET Email API
Aspose.Email for .NET has provided complete support for working with appointments inside various .NET(C#, VB, ASP, J# etc.) applications. It allows software developers to load, create, read, modify and store appointments in ICS file format inside their own .NET applications. You can add the following information while creating an appointment location, summary, description, start date, end date, organizer and attendees. The appointment file can be opened in Microsoft Outlook or any software that can load an ICS file. You can easily add and read multiple events from ICS file with just couple of lines of C# code. It is also possible to create an appointment request in Draft mode. The library also supports setting the status of appointment attendees while formulating a reply message.
How to Create & Save Appointment to Disk in ICS Format via C# API?
// Create and initialize an instance of the Appointment class
Appointment appointment = new Appointment(
"Meeting Room 3 at Office Headquarters",// Location
"Monthly Meeting", // Summary
"Please confirm your availability.", // Description
new DateTime(2015, 2, 8, 13, 0, 0), // Start date
new DateTime(2015, 2, 8, 14, 0, 0), // End date
"from@domain.com", // Organizer
"attendees@domain.com"); // Attendees
// Save the appointment to disk in ICS format
appointment.Save(dstEmail, AppointmentSaveFormat.Ics);
Console.WriteLine("Appointment created and saved to disk successfully.");
Working with Outlook MSG Messages via C# API
Aspose.Email for .NET has included very powerful features that enables software developers to create and manipulate Outlook message (MSG) files inside their own C# applications. The library supports creating MSG messages, add attachments to messages, MSG message generation with an RTF body, save message as a draft, body compression support and so on. It is also very easy to modify the properties of an MSG file, such as To, From, Subject, Body, Attachments, etc. Another great feature of the library is loading, viewing and parsing the MSG file to display its contents.
How to Convert Outlook Message File (MSG) to TIFF Image via C# API?
string dataDir = RunExamples.GetDataDir_KnowledgeBase();
MailMessage msg = MailMessage.Load(dataDir + "message3.msg", new MsgLoadOptions());
// Convert MSG to MHTML and save to stream
MemoryStream msgStream = new MemoryStream();
msg.Save(msgStream, SaveOptions.DefaultMhtml);
msgStream.Position = 0;
// Load the MHTML stream using Aspose.Words for .NET and Save the document as TIFF image
Document msgDocument = new Document(msgStream);
msgDocument.Save(dataDir + "Outlook-Aspose_out.tif", SaveFormat.Tiff);
Add & Manage Outlook Attachment via C# API
Aspose.Email for .NET makes it easy for software developers to create MSG messages with attachments using C#.NET API. The library has provided several important features for handling attachments inside outlook email messages, such as save attachment from outlook message, embedded messages as attachments, remove MSG attachments, reading embedded message from attachment, replace existing attachment with a new one, save attachments from digitally signed message and so on.
How to Add Attachments to Emails via .NET API?
// Create an instance of MailMessage class
var eml = new MailMessage
{
From = "sender@from.com",
To = "receiver@to.com",
Subject = "This is message",
Body = "This is body"
};
// Load an attachment
var attachment = new Attachment("1.txt");
// Add Multiple Attachment in instance of MailMessage class and Save message to disk
eml.Attachments.Add(attachment);
eml.AddAttachment(new Attachment("1.jpg"));
eml.AddAttachment(new Attachment("1.doc"));
eml.AddAttachment(new Attachment("1.rar"));
eml.AddAttachment(new Attachment("1.pdf"));
eml.Save("AddAttachments.eml");