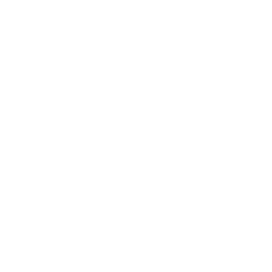
MSGReader
.NET Library for Outlook MSG Files Processing
Open Source C# .NET API to Read, Write, Modify, Manipulate, and Convert MS Outlook MSG and EML files.
What is MSGReader?
MSGReader is Open Source C# .NET library for reading Outlook MSG and EML files. It enables developers to read outlook MSG and EML files without using Microsoft Outlook. The most common outlook objects such as E-mail, Appointment, Task, Contact card & Sticky note are fully supported. All body types in MSG files, such as Text, HTML, HTML embedded into RTF and RTF are also supported.
There are a few options available for the manipulation of MSG files in MSGReader. It allows developers to remove attachments from email messages; they can also save the file to a new one.
There are a few options available for manipulation of MSG files in MSGReader. It allows developers to remove attachments from email message; they can also save the file to a new one.
Getting Started with MSGReader
The easiest way to install MSGReader is via NuGet. To use it from Visual Studio’s Package Manager Console, please enter the following command.
Here is the command
Install-Package MSGReader
Using MSGReader from a COM based language like VB script or VB6.
First, you need to download the latest version and then open the MSGReader project, set the Platform target to X86 & then build the code in release mode, Get "MsgReader.dll" file from BuildOutput folder & Copy the file to the desired location. After that Register the file for COM interop using the following command.
Read & Save Outlook MSG Message Attachment using .NET
MSGReader enables C# developers to access email messages & its attachment of Outlook MSG and EML files. It provides support for reading an Outlook MSG file and saving the message body and all its attachments to an output folder.
How to Read MSG data via C# .NET API?
// Read a email .msg file
Message message = new MsgReader.Outlook.Storage.Message("fileformat.msg");
// Read sender
Console.WriteLine("Sender:" + message.Sender);
// Read sent on
Console.WriteLine("SentOn:" + message.SentOn);
// Read recipient to
Console.WriteLine("recipientsTo:" + message.GetEmailRecipients(MsgReader.Outlook.RecipientType.To, false, false));
// Read recipient cc
Console.WriteLine("recipientsCc:" + message.GetEmailRecipients(MsgReader.Outlook.RecipientType.Cc, false, false));
// Read subject
Console.WriteLine("subject:" + message.Subject);
// Read body html
Console.WriteLine("htmlBody:" + message.BodyHtml);
Convert Outlook MSG as Text File using .NET API
MSGReader API provides the features for saving outlook MSG as a text file using .NET API. Developers can easily access the MSG file contents. Create an instance of the save file dialog box and save the message in TXT file format.
How to Save Outlook Email as a Text via C# API?
var fileName = Path.Combine(AppDomain.CurrentDomain.BaseDirectory,
"suggestion.msg");
using (var msg = new MsgReader.Outlook.Storage.Message(fileName))
{
var sb = new StringBuilder();
var from = msg.Sender;
var sentOn = msg.SentOn;
var recipientsTo = msg.GetEmailRecipients(
MsgReader.Outlook.Storage.Recipient.RecipientType.To, false, false);
var recipientsCc = msg.GetEmailRecipients(
MsgReader.Outlook.Storage.Recipient.RecipientType.Cc, false, false);
var recipientsBCC = msg.GetEmailRecipients(
MsgReader.Outlook.Storage.Recipient.RecipientType.Bcc, false, false);
var subject = msg.Subject;
sb.AppendLine($" From: {from.DisplayName} {from.Email}");
sb.AppendLine($" Sent: {sentOn.Value}");
sb.AppendLine($" To: {recipientsTo}");
sb.AppendLine($" CC: {recipientsCc}");
sb.AppendLine($" BCC: {recipientsBCC}");
sb.AppendLine($"Subject: {subject}");
sb.AppendLine($" Body:");
sb.AppendLine(msg.BodyText);
File.WriteAllText(Path.Combine(
AppDomain.CurrentDomain.BaseDirectory, "suggestion.txt"),
sb.ToString());
}