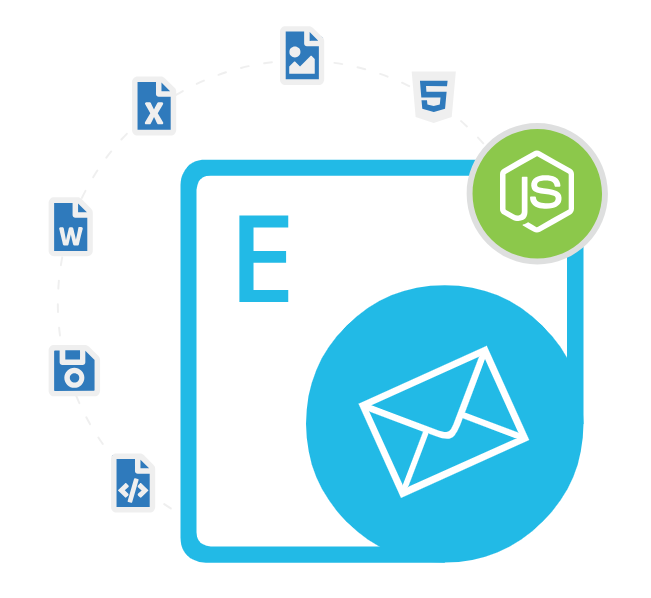
Aspose.Email Cloud SDK for Node.js
Node.js API to Create & Manage Email Messages
Create, Edit, Read, Manipulate and Convert Outlook Email File Formats Like MSG, EML, PST, ICS, VCF and OST via Node.js API.
What is Aspose.Email Cloud SDK for Node.js?
Managing emails efficiently is a critical component of many modern applications, whether for automating email workflows, integrating with CRM systems, or processing email attachments. Aspose.Email Cloud SDK for Node.js provides a powerful cloud-based API that enables software developers to work with email file formats effortlessly. This SDK supports creating, editing, converting, sending, and receiving emails, making it an essential tool for Node.js developers. There are various other important features also part of the SDK such as adding and managing email attachments, email storage handling in cloud, processing email headers and metadata, working with embedded objects and linked resources, manipulating Email signature, calendar event processing, extracting metadata for compliance reporting and so on.
The Aspose.Email Cloud SDK for Node.js provides developers with a powerful yet straightforward way to integrate email processing capabilities into their applications. It allows developers to create, manipulate, convert, and process email messages in various formats, such as EML, PST, MSG, OFT, EMLX, MBOX and many more. It simplifies the development process by abstracting away the complexities of email protocols and formats, allowing developers to focus on application logic. Node.js's cross-platform nature, combined with the cloud-based SDK, enables development on any operating system. It ensures email data protection with secure authentication mechanisms. Overall it is an excellent choice for developers looking to incorporate email functionality into their applications with ease.
Getting Started with Aspose.Email Cloud SDK for Node.js
The recommend way to install Aspose.Email Cloud SDK for Node.js is using npm. Please use the following command for a smooth installation.
Install Aspose.Email Cloud SDK for Node.js via npm
npm install @asposecloud/aspose-email-cloud --save
You can download the library directly from Aspose.Email product page
Create & Send Emails Programmatically
Aspose.Email Cloud SDK for Node.js makes it easy for software developers to programmatically create and manipulate email messages inside Node.js applications. with just a couple of lines of code Node.js developers can generate emails from scratch, set subject, body, recipients, and attachments, and even send them via SMTP. The following example demonstrates how software developers can create and send a simple email messages inside Node.js applications.
How to Create and Send a Simple Email Messages Inside Node.js Apps?
const asposeEmailCloud = require("aspose-email-cloud");
const config = {
clientId: "YOUR_CLIENT_ID",
clientSecret: "YOUR_CLIENT_SECRET",
};
const apiInstance = new asposeEmailCloud.EmailApi(config);
const message = {
from: "sender@example.com",
to: "recipient@example.com",
subject: "Test Email from Aspose.Email Cloud",
body: "This is a test email sent using Aspose.Email Cloud SDK for Node.js.",
};
const request = new asposeEmailCloud.SendEmailRequest({ message });
apiInstance.sendEmail(request)
.then((response) => {
console.log("Email sent successfully!");
})
.catch((error) => {
console.error("Error sending email:", error);
});
Email Format Conversion inside Node.js Apps
Aspose.Email Cloud SDK for Node.js has provided complete support for handling email file formats and conversion between these formats inside Node.js applications. The cloud SDK supports emails conversion between different formats without loss of data, such as MSG, PST, OST, OFT, EML, EMLX, MBOX and many more. Here is a very useful example that demonstrates how software developers can convert MSG file to EML format inside Node.js applications.
How to Convert MSG to EML via Node.js REST API?
const { EmailCloud, EmailConvertRequest } = require('aspose-email-cloud');
const emailApi = new EmailCloud({
appSid: 'YOUR_APP_SID',
appKey: 'YOUR_APP_KEY'
});
async function convertMsgToEml() {
try {
const request = new EmailConvertRequest({
fromFormat: 'msg',
toFormat: 'eml',
file: 'input.msg' // Can be a file path or Buffer
});
const result = await emailApi.email.convert(request);
console.log('Converted successfully:', result);
} catch (error) {
console.error('Conversion failed:', error);
}
}
convertMsgToEml();
Add & Manage Email Attachments via Node.js API
Aspose.Email Cloud SDK for Node.js has provided complete support for effortlessly adding, extracting, or removing attachments from email messages dynamically. With a couple of lines of code software developers can add, extract, and manage attachments within email messages. This is crucial for applications that require file sharing or document processing. The following example demonstrates how Node.js developers can extract attachments from an email messages inside Node.js environment.
How to Extract & Download Attachments from Email Messages via Node.js REST API?
const { EmailCloud, AttachmentDownloadRequest } = require('aspose-email-cloud');
const emailApi = new EmailCloud({
appSid: 'YOUR_APP_SID',
appKey: 'YOUR_APP_KEY'
});
async function downloadAttachment() {
try {
const attachment = await emailApi.email.attachment.download(
new AttachmentDownloadRequest({
fileName: 'email.eml',
attachmentName: 'document.pdf'
})
);
console.log('Attachment downloaded:', attachment);
} catch (error) {
console.error('Error downloading attachment:', error);
}
}
downloadAttachment();
Parse & Extract Email Data in Node.js
The Aspose.Email Cloud SDK for Node.js allows for parsing of emails, and extracting information from emails. This is very useful for data extraction, and data analysis. The SDK makes it easy for Node.js developers to read email files in different formats and extract subject, body, sender, recipients, and attachments with just a couple of lines of code. The following example demonstrates how software developers can extract email metadata inside Node.js environment.
How to Extract Email Metadata inside Node.js Apps?
const { EmailCloud, EmailGetRequest } = require('aspose-email-cloud');
const emailApi = new EmailCloud({
appSid: 'YOUR_APP_SID',
appKey: 'YOUR_APP_KEY'
});
async function parseEmail() {
try {
const email = await emailApi.email.get(
new EmailGetRequest({ filePath: 'email.eml' })
);
console.log('Subject:', email.subject);
console.log('From:', email.from.address);
console.log('Body:', email.body);
console.log('Attachments:', email.attachments?.length);
} catch (error) {
console.error('Error parsing email:', error);
}
}
parseEmail();