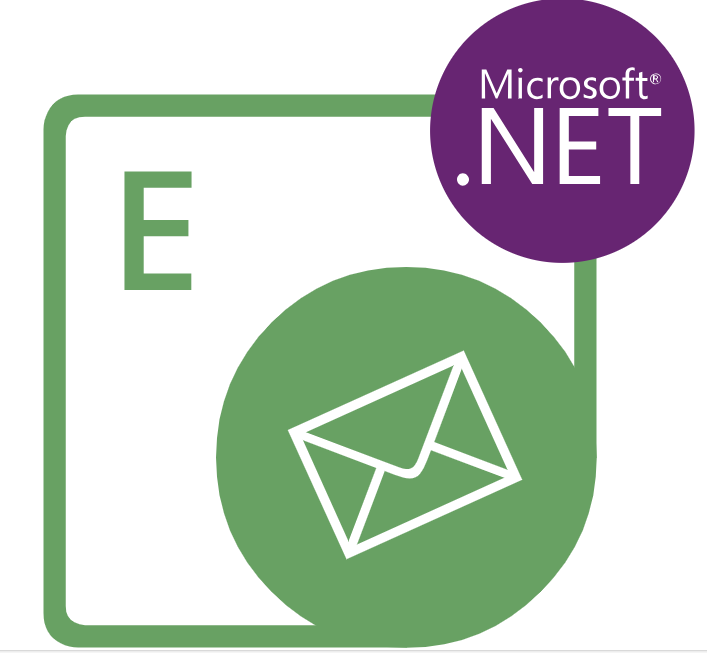
Aspose.Email for Node.js via .NET
Node.js API to Create & Manage Email Messages
Create, Edit, Read, Manipulate and Convert Outlook Email File Formats Like MSG, EML, PST, ICS, VCF and OST via Node.js API.
What is Aspose.Email for Node.js via .NET?
Aspose.Email for Node.js via .NET provides a robust tool for developers who want to work with email files in their Node.js applications. This API is flexible and tailored to help you build strong applications that deal with different email functions. It lets you create, read, convert, and work with email messages, manage attachments, and handle contact details efficiently. The API supports various email formats such as MSG, PST, OST, OFT, EML, EMLX, MBOX and many more.
Aspose.Email for Node.js via .NET API is like a powerful toolbox merging Aspose.Email for .NET with the flexibility of Node.js. This merging gives developers the best of both worlds. You can tap into the full range of Aspose.Email features right within the Node.js setup, making email management apps more efficient and powerful. The library packs key features create new email messages, reading existing ones, managing Outlook storage files, adding and extracting attachments, modifying attachments, working with calendar items, handling contact information, recognizing text from attachments and many more.
Aspose.Email for Node.js via .NET makes it easy for developers like you to handle emails using various protocols. You can send and receive emails through different mail servers effortlessly. This tool also helps convert email messages from one format to another, making sure they work well on different platforms. Additionally, it lets you connect with an OCR library or API to change attachments into text that you can edit and search. Software developers can transform email attachments into searchable formats, allowing users to conduct full-text searches on email archives. This is especially beneficial for legal, compliance, and auditing purposes. Aspose's extensive documentation and robust features make it easier to integrate these capabilities, allowing developers to focus on building unique solutions for their users.
Getting Started with Aspose.Email for Node.js via .NET
The recommend way to install Aspose.Email for Node.js via .NET is using npm. Please use the following command for a smooth installation.
Install Aspose.Email for Node.js via .NET via npm
npm install @aspose/email
You can download the library directly from Aspose.Email product page
Create and Send Email in Node.js Apps
Aspose.Email for Node.js via .NET has provided complete functionality for creating and sending email messages inside Node.js applications. There are several other important features part of the API, such as create new email message from the scratch, modify existing email messages, add attachments to emails, extract attachments, creating an email with an image attachment, managing calendar items and many more. Here’s a simple example that allows software developers to create an email with an image attachment inside Node.js applications.
How to Create a New Messages and Attach an Image to It via Node.js API
const aspose = require('aspose.email');
let email = new aspose.EmailMessage();
email.subject = "OCR Attachment Example";
email.body = "This email contains an attachment for OCR processing.";
email.to.add("recipient@example.com");
let attachment = aspose.Attachment.load("path/to/image.png");
email.attachments.add(attachment);
email.save("path/to/email.msg");
Manage Attachment to Email Messages in Node.js
Aspose.Email for Node.js via .NET API enables software developers to seamlessly add and manage attachments to email messages inside Node.js applications. The APIs has included various features for working with attachments inside email messages such as adding, extracting, deleting and modifying attachments within email messages, embedded messages as attachments, reading embedded message from attachment, replace existing attachment with a new one, save attachments from digitally signed message and so on. Here is an example that shows how you can save attachments to the local disk from an email message inside Node.js applications.
How to Save Attachments to Local Disk from an Email Message via Node.js API?
// Create an instance of MapiMessage from file
MapiMessage message = MapiMessage.FromFile(dataDir + fileName);
// Iterate through the attachments collection
foreach (MapiAttachment attachment in message.Attachments)
{
// Save the individual attachment
attachment.Save(dataDir + attachment.FileName);
}
Email Conversion to Other File Formats in Node.js
One of the standout features of Aspose.Email for Node.js via .NET is its ability to convert email messages between different formats, such as MSG, PST, OST, OFT, EML, EMLX, MBOX and many more. This features is very useful for applications that need to support multiple email standards and formats. Here is a useful example that shows how software developers can convert an Email to EML Format inside Node.js applications.
How to Convert an Email to EML Format inside Node.js Apps?
const aspose = require('aspose.email');
let email = aspose.EmailMessage.load("path/to/email.msg");
email.save("path/to/email.eml", aspose.SaveOptions.defaultEml);
Add & Manage Calendar Items in Emails in Node.js
Aspose.Email for Node.js via .NET supports calendar integration, allowing software developers to create and manage calendar items inside their own Node.js applications. Developers can work with calendar items, appointments, and tasks. This feature is particularly useful for applications that require scheduling and event management. It allows software developers to load, create, read, modify and store appointments in ICS file format with just a couple of lines of code. Here is an example that shows how software developers can create a Calendar event inside Node.js Apps.
How to Create a Calendar Event inside Node.js Apps?
Aspose.Email for Node.js via .NET API
const aspose = require('aspose.email');
let appointment = new aspose.Appointment(
"Conference Room",
"Project Meeting",
"Discuss project milestones and deliverables.",
new Date(2024, 7, 24, 10, 0),
new Date(2024, 7, 24, 11, 0),
"organizer@example.com",
["attendee1@example.com", "attendee2@example.com"]
);
let email = new aspose.EmailMessage();
email.addAlternateView(appointment.requestApointment());
email.save("project_meeting.ics");