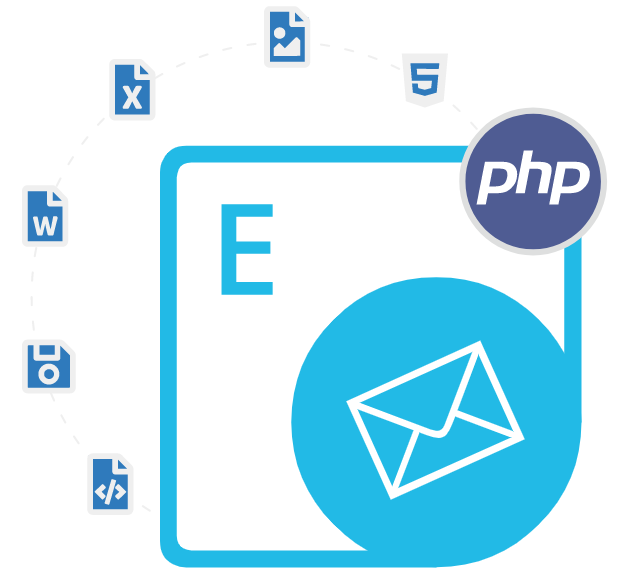
Aspose.Email Cloud SDK for PHP
PHP API to Process Microsoft Outlook Emails
PHP Email Processing Cloud SDK that allows to Compose, Send, Receive, Edit & Convert Outlook MSG, MHT, EML, EMLX, and other email file formats.
Aspose.Email Cloud SDK for PHP uses a very useful and reliable API that enables software developers to work with email messages and other related tasks inside their PHP-based applications. It is built on the top of a very popular Aspose.Email Cloud API that makes developers life easy by providing them a rich set of features for working with email messages, attachments, calendars, and other related tasks. It is built on top of a powerful and robust cloud infrastructure, which ensures that it can handle high volumes of traffic and data without any issues.
Aspose.Email Cloud SDK for PHP is very easy to use and includes a wide range of useful features for handling email messages inside their own PHP applications. It includes features like create and send simple or MIME email messages, manage email message attachments, add CC and BCC recipients, email messages encoding and decoding, email document headers, set delivery notification options, work with calendars and schedule appointments, manage email contacts, mark special emails via setting flag, fetch email properties and many more. Moreover, it is very easy to convert email messages between various formats such as EML, MSG, MHTML, and HTML using the SDK.
Aspose.Email Cloud SDK for PHP is very flexible and is designed to work with a wide range of email messaging protocols, including SMTP, POP3, and IMAP. It allows software developers to use the SDK to integrate email messaging capabilities into a wide range of applications, including web-based applications, mobile applications, and desktop applications. Overall, Aspose.Email Cloud SDK for PHP is an ideal choice for developers who need to build robust and scalable email messaging applications that can handle a large number of users and messages.
Getting Started with Aspose.Email Cloud SDK for PHP
The recommend way to install Aspose.Email Cloud SDK for PHP is using composer. Please use the following command for a smooth installation.
Install Aspose.Email Cloud SDK for PHP via Composer
composer require aspose/aspose-email-cloud
You can also download it directly from Aspose product page.Create and Manage Email Messages
Aspose.Email Cloud SDK for PHP is an excellent choice for developers who want to create, send, receive, append, flag, and convert cloud emails inside their own PHP applications. The API supports working with various email file formats such as EML, MSG, MHTML, and HTML format. There are several important features part of the library for working with email messages, such as creating email messages from the scratch, send an email with attachments, inserting customize title for email messages, setting email date, Adding CC and BCC recipients, and many more.
How to Create Email File via PHP API
$email = (new EmailDto())
->setFrom(new MailAddress("Organizer Name", "organizer@aspose.com"))
->setTo(array(new MailAddress("Attendee Name", "attendee@aspose.com")))
->setSubject("Some subject")
->setBody("Some body");
$storage = "First storage";
$folder = "folder/on/storage";
$emailFile = "email.eml";
$format = "Eml";
$api->email()->save(new EmailSaveRequest(
new StorageFileLocation($storage, $folder, $emailFile),
$email, $format));
Create & Manage Appointments via PHP REST API
An ICS file format is used to for iCalendar which is a standardized format for storing and transmitting calendar data. Aspose.Email Cloud SDK for PHP enables software developers to create and read iCalendar (.ics) files inside their own PHP applications. The SDK supports several important features for handling appointments using PHP commands, such as creating a new appointment, Getting appointments from a specific folder, getting details of a specific appointment, updating an existing appointment, Deleting an appointment, and so on. The following example shows how software developers can Create a new appointment inside the PHP application.
Create a New Appointment inside PHP Applications
$api = new \Aspose\Email\Api\AppointmentApi(null, $config);
$request = new \Aspose\Email\Model\CreateAppointmentRequest();
$request->setMailAccountId("Your email account ID");
$request->setFolder("Calendar");
$request->setAppointment($appointment); // replace $appointment with your appointment object
$response = $api->createAppointment($request);
//Get details of a specific appointment:
$request = new \Aspose\Email\Model\GetAppointmentRequest();
$request->setMailAccountId("Your email account ID");
$request->setFolder("Calendar");
$request->setMessageId("Appointment ID");
$response = $api->getAppointment($request);
$appointment = $response->getAppointment();
Convert Email Message & Contact Files via PHP API
Aspose.Email Cloud SDK for PHP has included complete support for converting email messages and contact files to other supported file formats inside their own PHP applications. The library has provided support for email messages conversion to EML, MSG, MHTM, HTML and many more. It also provides support for converting iCalendar to Microsoft Outlook MSG, creating iCalendar to MAPI or ICS files, converting MSG file to an iCalendar file, Convert Calendar File to ICS, Convert VCard Files, and many more. The following example demonstrates how to convert email message file formats using PHP commands.
MSG Email Message Conversion to EML via PHP API
$emailDto = (new EmailDto())
->setFrom(new MailAddress(null, 'from@aspose.com'))
->setTo(array(new MailAddress(null, 'to@aspose.com')))
->setSubject('Some subject')
->setBody('Some body')
->setDate(new DateTime());
$mapi = $api->email()->asFile(
new EmailAsFileRequest('Msg', $emailDto));
$eml = $api->email()->convert(
new EmailConvertRequest('Msg', 'Eml', $mapi));
$fileContent = $eml->fread($eml->getSize());
$this->assertRegExp(
"/" . $emailDto->getFrom()->getAddress() . "/",
$fileContent);
$dto = $api->email()->fromFile(
new EmailFromFileRequest('Eml', $eml));
$this->assertEquals(
$emailDto->getFrom()->getAddress(),
$dto->getFrom()->getAddress());