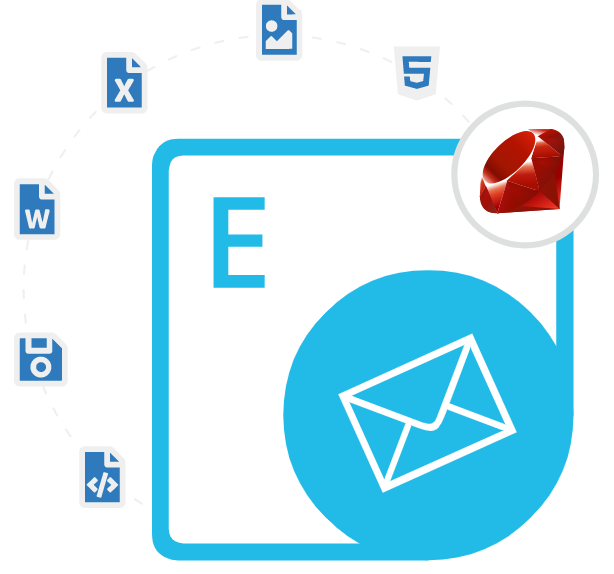
Aspose.Email Cloud SDK for Ruby
Ruby REST API to Process Microsoft Outlook Emails
A Powerful Outlook Email Processing REST API for composing, sending, receiving, manipulating & converting Email Messages in the Cloud.
Aspose.Email Cloud SDK for Ruby provides a comprehensive set of tools for working with Microsoft Outlooks email file formats in the cloud. It helps software developers to create powerful cloud applications for creating, sending, receiving, and manipulating email messages without using Microsoft Outlook or any other software. It provides secure data transfer using HTTPS and SSL encryption, ensuring that your data is safe and secure. The cloud SDK is platform-independent and can be used on any operating system or programming language that supports Ruby.
Aspose.Email Cloud SDK for Ruby is very features rich and provides a variety of features for programmatically working with email messages in the cloud, such as sending email messages using SMTP, using SMTP or Exchange or Google Mail servers, set various message properties (as subject, body, attachments, and recipients), receive email messages from POP3 and IMAP servers, extract various information from email messages (sender, recipient, subject, body, attachments, and headers), search email messages using various criteria and many more.
Aspose.Email Cloud SDK for Ruby provides an easy to use, simple, and intuitive API that provides several benefits for developers who want to work with email messages in their Ruby applications. The library supports working with standard email file formats such as Outlook MSG, EML, iCalendar files, VCard, and many more. Software developers can also convert email messages between various formats such as EML, MSG, MHTML, and HTML using the SDK. With its wide range of features and benefits, the SDK is an excellent choice for developers who want to work with email messages in their Ruby applications.
Getting Started with Aspose.Email Cloud SDK for Ruby
The recommend way to install Aspose.Email Cloud SDK for Ruby is using RubyGems package manager. Please use the following command for a smooth installation.
Install Aspose.Email Cloud SDK for Ruby via RubyGems
gem install aspose_email_cloud
You can also download it directly from Aspose product page.Send & Receive Email Messages via Ruby REST API
Aspose.Email Cloud SDK for Ruby gives software developers the capability to compose and send email messages inside their own Ruby applications. The SDK fully support sending email messages using SMTP, Exchange, or Google Mail servers. Software developers can easily set various message properties such as subject, body, attachments, and recipients. You can also append a new message to your email account with ease. It is also possible to receive email messages from POP3 and IMAP servers.
How to Append New Message to Your Email Account via Ruby API
email = EmailDto.new(
from: MailAddress.new(address: 'example@gmail.com'),
to: [MailAddress.new(address: 'to@aspose.com')],
subject: 'Some subject',
body: 'Some body'
)
appended_message_id = api.client.message.append(
ClientMessageAppendRequest.new(
account_location: imap_location,
folder: imap_folder_name,
message: MailMessageDto.new(value: email),
mark_as_sent: true))
Convert Email from One Format to Another via Ruby
Aspose.Email Cloud SDK for Ruby enables software developers to load email messages and convert them to from one format to another via Ruby REST API. It provides support for various file formats, such as EML, MSG, MHTM, HTML files can be converted to each other. Apart from email messages, software developers can also convert Calendar (iCalendar) and Contact Card (VCard) Files inside their own applications. The following example demonstrates how to convert email messages to other supported file formats.
Convert Email from One Format to Another via Ruby
mapi_file = api.email.convert(
EmailConvertRequest.new(
from_format: 'Eml',
to_format: 'Msg',
file: File.new('email.eml')))
converted = File.open(mapi_file, 'rb') do |f|
bin = f.read
# ...
end
Manage Email Attachments & Embedded Objects via Ruby API
Aspose.Email Cloud SDK for Ruby is a powerful tool that allows developers to work with email attachments and embedded objects in their Ruby applications. Using Ruby Cloud SDK, software developers can easily manage attachments and embedded objects, such as images, inside their email messages. Software developers can add attachments to email messages using the "Add Attachment" method by specifying the file path, name, and content type of the attachment. It is also possible to get or download and delete attachments.
Add Attachments to Email Messages via Ruby API
# Instantiate the EmailApi class
email_api = AsposeEmailCloud::EmailApi.new
# Upload attachment to cloud storage
file_name = "example.pdf"
path = "example_folder/#{file_name}"
file = File.new(file_name, 'rb')
email_api.upload_file(AsposeEmailCloud::UploadFileRequest.new(path, file))
# Add attachment to email message
message = AsposeEmailCloud::EmailDto::Message.new
message.to_address = AsposeEmailCloud::EmailDto::MailAddress.new('recipient@example.com')
message.from_address = AsposeEmailCloud::EmailDto::MailAddress.new('sender@example.com')
message.subject = 'Email with Attachment'
message.body = 'Please see attached file'
attachment = AsposeEmailCloud::EmailDto::Attachment.new
attachment.name = file_name
attachment.data_base64 = Base64.encode64(file.read)
attachment.content_type = 'application/pdf'
message.attachments = [attachment]
# Send email message
email_api.send(AsposeEmailCloud::SendEmailRequest.new(message))