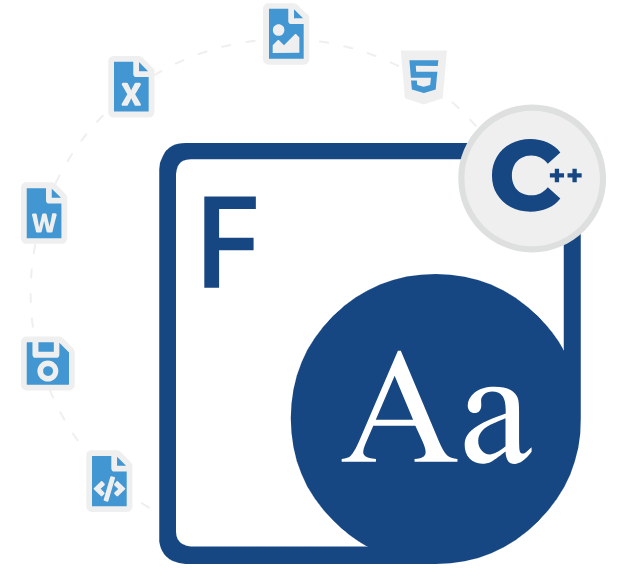
Aspose.Font for C++
C++ Font API to Read, Save & Convert Fonts
C++ Library allows to Load, Read, Save and Convert different font formats such as TrueType, WOFF, EOT, OpenType, CFF, and Type1 within C++ based applications.
Aspose.Font for C++ is a very powerful tool that simplifies font handling and offers an extensive set of features for software developers and programmers. It empowers C++ developers to work with various font formats and perform a wide range of font-related tasks. It enables software developers to load, generate, edit, and convert font files in various formats, such as TrueType (TTF), OpenType (OTF), PostScript Type 1 (PFB/PFA), WOFF, EOT, and many others. The API can elevate your font-related tasks to the next level without any external dependencies.
Aspose.Font for C++ is a feature-rich library that provides comprehensive font manipulation capabilities for C++ developers. The library supports a wide range of font operations, including extracting font metadata, Load font files from disc or stream, accessing glyph information, converting fonts to different formats, reading font information, updating font files & saving it to the disc, reading metrics information from Font files, rendering text using font glyphs, merging TrueType fonts and much more. It supports 32-bit as well as 64-bit operating systems.
Aspose.Font for C++ offers advanced typographic capabilities, including handling ligatures, contextual and discretionary glyph substitutions, and advanced text layout options. These features are particularly useful when working with complex scripts or languages that require sophisticated text shaping and rendering. The API is cross-platform and allows users to use it seamlessly on different operating systems, including Windows, Linux, and macOS. If you're looking to enhance font manipulation capabilities in your C++ applications, Aspose.Font is undoubtedly worth considering.
Getting Started with Aspose.Font for C++
The recommend way to install Aspose.Font for C++ is using NuGet. Please use the following command for a smooth installation.
Install Aspose.Font for C++ via NuGet Command
NuGet\Install-Package Aspose.Font.Cpp -Version 23.4.0
You can download the library directly from Aspose.Font for C++ product page
Font Conversion to Supported File Formats via C++ API
One of the notable features of Aspose.Font for C++ is its ability to convert fonts between different supported file formats. This becomes very convenient when software developers need to convert fonts to a specific format compatible with your target environment or platform. The library supports seamless conversion between TrueType, OpenType, CFF, PostScript Type 1 fonts, and more allowing developers to cater to a wide range of scenarios. The following example shows how to load font from any of the supported formats like TTF and convert it to WOFF2 using C++ commands.
Convert TTF to WOFF2 using C++ ApI
using namespace System;
using namespace Aspose::Font::Sources;
using namespace Aspose::Font::Ttf;
using namespace Aspose::Font;
// Open TTF font
SharedPtr fontFileDefinition = MakeObject(MakeObject(u"Montserrat-Regular.ttf"));
SharedPtr fontDefinition = MakeObject(FontType::TTF, fontFileDefinition);
SharedPtr font = Font::Open(fontDefinition);
// WOFF2 output settings
SharedPtr outStream = IO::File::Create(u"Montserrat-Regular.woff2");
// Convert TTF to WOFF2
font->SaveToFormat(outStream, FontSavingFormats::WOFF2);
Load and Extract Type1 Fonts via C++ API
Aspose.Font for C++ allows software developers to load Type1 as well as other supported font formats from different sources, such as local files, streams, or URLs. Software developers can load fonts individually or in bulk, making it easy to process multiple fonts simultaneously. Once loaded, developers can update properties of the fonts and save them back to the desired format or export them to other formats with ease. The following example shows how to load and extract data from type1 fonts inside C++ applications.
How to Load and Extract Data from Type1 Fonts using C++ API?
System::String fileName = dataDir + u"courier.pfb";
//Font file name with full path
System::SharedPtr fd = System::MakeObject(Aspose::Font::FontType::Type1, System::MakeObject(u"pfb", System::MakeObject(fileName)));
System::SharedPtr font = System::DynamicCast_noexcept(Aspose::Font::Font::Open(fd));
Font Metrics and Glyphs Support
Aspose.Font for C++ is a very feature-rich library that provides access to a wealth of font metrics, including ascent, descent, line gap, and various other essential measurements. Software developers can retrieve glyph information such as Unicode values, bounding boxes, advance widths, and more. These features enable users to accurately position and render text within your applications. The following example shows how software developers can render text with the glyphs using C++ commands.
How to Render Text with the Glyphs in C++ Applications?
using Aspose::Font::Glyphs;
using Aspose::Font::Rendering;
using Aspose::Font::RenderingPath;
class GlyphOutlinePainter: IGlyphOutlinePainter
RenderingText::GlyphOutlinePainter::GlyphOutlinePainter(System::SharedPtr path)
{
_path = path;
}
void RenderingText::GlyphOutlinePainter::MoveTo(System::SharedPtr moveTo)
{
_path->CloseFigure();
_currentPoint.set_X((float)moveTo->get_X());
_currentPoint.set_Y((float)moveTo->get_Y());
}
void RenderingText::GlyphOutlinePainter::LineTo(System::SharedPtr lineTo)
{
float x = (float)lineTo->get_X();
float y = (float)lineTo->get_Y();
_path->AddLine(_currentPoint.get_X(), _currentPoint.get_Y(), x, y);
_currentPoint.set_X(x);
_currentPoint.set_Y(y);
}
void RenderingText::GlyphOutlinePainter::CurveTo(System::SharedPtr curveTo)
{
float x3 = (float)curveTo->get_X3();
float y3 = (float)curveTo->get_Y3();
_path->AddBezier(_currentPoint.get_X(), _currentPoint.get_Y(), (float)curveTo->get_X1(), (float)curveTo->get_Y1(), (float)curveTo->get_X2(), (float)curveTo->get_Y2(), x3, y3);
_currentPoint.set_X(x3);
_currentPoint.set_Y(y3);
}
void RenderingText::GlyphOutlinePainter::ClosePath()
{
_path->CloseFigure();
}
System::Object::shared_members_type Aspose::Font::Examples::WorkingWithTrueTypeAndOpenTypeFonts::RenderingText::GlyphOutlinePainter::GetSharedMembers()
{
auto result = System::Object::GetSharedMembers();
result.Add("Aspose::Font::Examples::WorkingWithTrueTypeAndOpenTypeFonts::RenderingText::GlyphOutlinePainter::_path", this->_path); result.Add("Aspose::Font::Examples::WorkingWithTrueTypeAndOpenTypeFonts::RenderingText::GlyphOutlinePainter::_currentPoint", this->_currentPoint);
return result;
}
Font Manipulation & Editing via C++ API
Aspose.Font for C++ allows software developers to load Type1 as well as other supported font formats from different sources, such as local files, streams, or URLs. Software developers can load fonts individually or in bulk, making it easy to process multiple fonts simultaneously. Once loaded, developers can update properties of the fonts and save them back to the desired format or export them to other formats with ease. The following example shows how to load and extract data from type1 fonts inside C++ applications.
Read Font Metrics information from Type1 Fonts inside C++ Apps
System::String fileName = dataDir + u"courier.pfb";
//Font file name with full path
System::SharedPtr fd = System::MakeObject(Aspose::Font::FontType::Type1, System::MakeObject(u"pfb", System::MakeObject(fileName)));
System::SharedPtr font = System::DynamicCast_noexcept(Aspose::Font::Font::Open(fd));
System::String name = font->get_FontName();
System::Console::WriteLine(System::String(u"Font name: ") + name);
System::Console::WriteLine(System::String(u"Glyph count: ") + font->get_NumGlyphs());
System::String metrics = System::String::Format(u"Font metrics: ascender - {0}, descender - {1}, typo ascender = {2}, typo descender = {3}, UnitsPerEm = {4}", font->get_Metrics()->get_Ascender(), font->get_Metrics()->get_Descender(), font->get_Metrics()->get_TypoAscender(), font->get_Metrics()->get_TypoDescender(), font->get_Metrics()->get_UnitsPerEM());
System::Console::WriteLine(metrics);