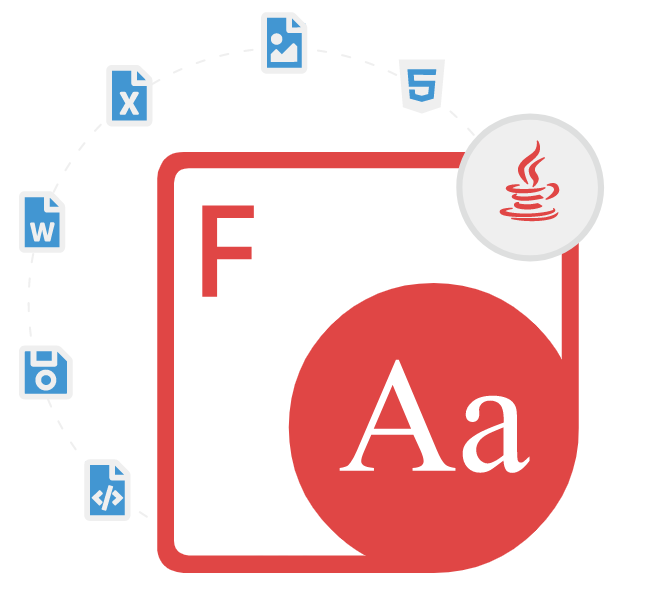
Aspose.Font for Java
Java Font API to Load, Create & Convert Fonts
A Powerful Java Library that allows to Load, save, draw, convert and render font file format like TrueType, WOFF, CFF, EOT, OpenType, and Type1 with ease.
Aspose.Font for Java is an advanced Font management library that enables Java developers to work with various types of fonts inside their own Java applications. The library has incorporated a wide range of features that allows software developers to load, render, create, read, manipulate and convert font files with ease. The library supports extracting information about fonts, and even convert fonts from one format to another without any external dependencies.
Aspose.Font for Java supports multiple front formats such as TrueType (with TrueType collections), WOFF, CFF, EOT, OpenType, and Type. It provides an easy-to-use API for loading and parsing fonts in various formats and with just a couple of lines of code, programmers can load a font file and access its various properties, such as the font name, style, size, weight, variants, font family and many more. Using the API, software developers can subset fonts to reduce their size and improve their performance. This is especially useful when working with web fonts or embedding fonts in PDF documents.
Aspose.Font for Java is very easy to use and provides full support for creating custom fonts from scratch. This can be useful when you need to create a unique font for your application or project. It is also possible to embed fonts in PDF documents, which ensures that the fonts are displayed correctly on all devices. With just a couple of lines code developers can convert font between various other supported file format such as TrueType, OpenType, WOFF, WOFF2, CFF, and SVG formats. With its robust & advanced set of features and comprehensive documentation, Aspose.Font is an excellent choice for developers who need to work with fonts in their Java applications.
Getting Started with Aspose.Font for Java
The recommend way to install Aspose.Font for Java is using Maven. Please use the following command for a smooth installation.
Maven Dependency for Aspose.Font for Java
// First you need to specify Aspose Repository configuration / location in your Maven pom.xml as follows:
<repositories>
<repository>
<id>snapshots</id>
<name>repo;/name>
<url>http://repository.aspose.com/repo/</url>
</repository>
</repositories>
//Then define Aspose.Font for Java API dependency in your pom.xml as follows:
<dependencies>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-font;/artifactId>
<version>20.10</version>
</dependency>
</dependencies>
You can download the library directly from Aspose.Font for Java product page
Fonts Conversion to Other Formats via Java API
Aspose.Font for Java has included support for loading and converting Fonts to other supported file formats inside their Java applications. The library has included support for several important font conversion options, including conversion between TrueType, OpenType, WOFF, WOFF2, CFF, and SVG formats. This allows you to convert fonts to the format that best suits your needs, whether it's for web development, desktop publishing, or other applications. The following code snippet demonstrates how to convert a font file from Type 1 to Woff file format using Java code.
Convert font formats from Type 1 to Woff using Java
// Open Type 1 font
byte[] fontMemoryData = Files.readAllBytes(Paths.get(getDataDir(), "Courier.pfb"));
FontDefinition fontDefinition = new FontDefinition(FontType.Type1, new FontFileDefinition("pfb", new ByteContentStreamSource(fontMemoryData)));
Font font = Font.open(fontDefinition);
// Woff output settings
String outPath = Paths.get(getOutputDir(), "Type1ToWoff_out3.ttf").toString();
FileOutputStream outStream = new FileOutputStream(outPath);
// Convert type1 to woff
font.saveToFormat(outStream, FontSavingFormats.WOFF);
Work with TrueType Font via Java API
Aspose.Font for Java is a very useful Java API allows software developers to work with various types of Fonts such as TrueType and open type fonts inside their Java applications. The library allows to load and read TrueType Font types from files stored in your digital storage. It also supports opening TTF font files and read the Font Metrics information. It is also possible to render text using Java API. The following example shows how to Load and Save TrueType Font from Disc using Java commands.
How to Load & Save TrueType Font from Disc via Java?
String fileName = Utils.getDataDir() + "Montserrat-Regular.ttf"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new FileSystemStreamSource(fileName)));
TtfFont font = (TtfFont) Font.open(fd);
byte[] fontMemoryData = Utils.getInputFileBytes("Montserrat-Regular.ttf"); //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new ByteContentStreamSource(fontMemoryData)));
TtfFont font = (TtfFont) Font.open(fd);
//Work with data from just loaded TtfFont object
//Save TtfFont to disk
//Output Font file name with full path
String outputFile = Utils.getDataDir() + "Montserrat-Regular_out.ttf";
font.save(outputFile);
Fonts Loading inside Java Apps
Aspose.Font provides an easy-to-use API that provides complete support for loading and parsing fonts in various file formats, including TrueType, OpenType, WOFF, WOFF2, CFF and many more. With just a few lines of code, developers can load a font file and access its various properties, such as the font name, style, size, weight, family, variant, character set and so on. The following code snippet demonstrates how to easily can software developers load a font file and retrieve its name inside their Java applications.
How to Load a Font File & Retrieve Its Name via Java API?
// Load the font file
FontDefinition fontDefinition = new FontDefinition(FileUtils.readFileToByteArray(new File("myfont.ttf")));
// Retrieve the font name
String fontName = fontDefinition.getFontName();