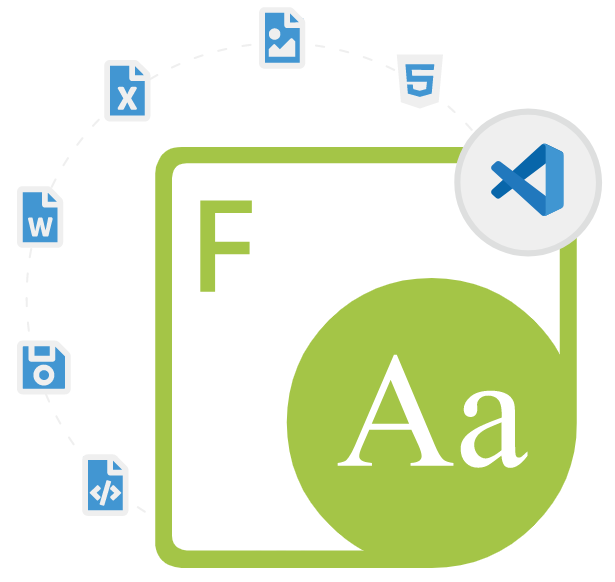
Aspose.Font for .NET
.NET Font API to Load, Save & Convert Fonts
C# .NET API enables software developers to Load, Encode, Save & Convert TrueType, CFF, OpenType, and Type1 within .NET Apps.
Fonts play an essential role in creating & managing visually appealing documents, presentations, brochures, and designs. However, managing fonts can be a very challenging task, especially when dealing with multiple fonts across different platforms and devices. Aspose.Font for .NET offers a comprehensive and advanced solution that enables software developers to Load, Encode, Save & Convert various types of font inside their own .NET applications. The library provides a simple and intuitive interface to load fonts from a variety of sources, such as local files, streams, URLs, system directories as well as use custom font search paths.
Aspose.Font for .NET is a very easy-to-handle and feature-rich font management API that allows software developers to work with different font families such as TrueType, OpenType, and Type1 fonts inside their own .NET applications. The library provides a unified interface to work with fonts, regardless of the underlying format or platform. Using Aspose.Font API, software developers can perform a wide range of font-related tasks, such as loading, installing, and embedding fonts, as well as converting fonts to different formats without any external dependencies.
Aspose.Font for .NET ensures compatibility across different platforms and devices by allowing developers to embed fonts in their documents or convert fonts to different formats. This is a very useful feature, especially when working with legacy fonts or when dealing with complex typography. It also supports font subsetting features, which is very helpful in reducing file sizes and improving rendering performance. With its easy deployment and self-contained library, Aspose.Font for .NET is the ultimate solution for font management in .NET applications.
Getting Started with Aspose.Font for .NET
The recommend way to install Aspose.Font for .NET is using NuGet. Please use the following command for a smooth installation.
Install Aspose.Font for .NET via NuGet Command
NuGet\Install-Package Aspose.Font -Version 23.3.0
You can download the library directly from Aspose.Font for .NET product page
Convert Fonts to Other Formats via C# API
Aspose.Font for .NET has included completely functionality that allows software developers to cprogrammatically using C# .NET code. Using the Aspose.Font API Software developers can convert various types of fonts such as OpenType, Web Open Font Format (WOFF and WOFF2), Type1, TrueType, Compact Font Format (CFF) and many more. The following example shows how easily can software developers converts TTF fonts to WOFF2 inside C# applications.
Convert TTF Fonts to WOFF2 using C#?
// Open ttf font
string fontPath = Path.Combine(DataDir, "Montserrat-Regular.ttf");
FontDefinition fontDefinition = new FontDefinition(FontType.TTF, new FontFileDefinition(new FileSystemStreamSource(fontPath)));
Font font = Font.Open(fontDefinition);
// Woff2 output settings
string outPath = Path.Combine(OutputDir, "Montserrat-Regular.woff2");
FileStream outStream = File.Create(outPath);
// Convert ttf to woff2
font.SaveToFormat(outStream, FontSavingFormats.WOFF2);
Work with True Type Fonts via C# API
Aspose.Font for .NET has included very powerful features for working with various types of fonts. It lets Software developers to read TrueType Font types from files stored in their digital storage. Software developers can load fonts from different sources, including local files, streams, URLs and so on. After loading the TrueType Font files, software developers can work with the font file, make changes to some parameters and would like to save the file to disc. The following example shows how to load True Type fonts and save it to disk via C# API.
How to Load True Type Fonts, Update It & Save it using C# .NET API?
string fileName= dataDir + "Montserrat-Regular.ttf"; //Font file name with full path
FontDefinition fd = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new FileSystemStreamSource(fileName)));
TtfFont ttfFont = Aspose.Font.Font.Open(fd) as TtfFont;
//byte array to load Font from
string dataDir = RunExamples.GetDataDir_Data();
byte[] fontMemoryData = File.ReadAllBytes(dataDir + "Montserrat-Regular.ttf");
FontDefinition fd = new FontDefinition(FontType.TTF, new FontFileDefinition("ttf", new ByteContentStreamSource(fontMemoryData)));
TtfFont ttfFont = Aspose.Font.Font.Open(fd) as TtfFont;
//Work with data from just loaded TtfFont object
//Save TtfFont to disk
//Output Font file name with full path
string outputFile = RunExamples.GetDataDir_Data() + "Montserrat-Regular_out.ttf";
ttfFont.Save(outputFile);
Rendering Text inside via C# API
Aspose.Font for .NET has included very useful features enabling software developers to render text inside their own .NET applications with ease. It provides a flexible and powerful API for rendering text in various ways. The simplest way to render text using the API is to draw it on a Graphics object and then by using the TextRenderingOptions class developers can render the text as an image. Software developers can also render Text as a Vector Graphic by creating a Font object and after that create a Graphics object and call the DrawPath method to draw the glyph path using a black pen and finally, we save the resulting vector graphic to an SVG file. The following example shows how to draw text on a graphics object and render text as an image using C# code.
Render Graphics Object to Text via .NET API
// Draw Text on an Object
using (var font = new Aspose.Font.Font("Arial.ttf"))
using (var graphics = new Aspose.Imaging.Graphics())
{
graphics.DrawString("Hello, World!", font, Aspose.Imaging.Brushes.Black, new Aspose.Imaging.PointF(10, 10));
graphics.Save("output.png", new Aspose.Imaging.ImageOptions.PngOptions());
}
//Rendering Text as an Image
using (var font = new Aspose.Font.Font("Arial.ttf"))
{
var options = new Aspose.Font.TextRenderingOptions("Hello, World!", font, 24, Aspose.Font.Color.Black);
var image = options.ToImage();
image.Save("output.png", new Aspose.Imaging.ImageOptions.PngOptions());
}