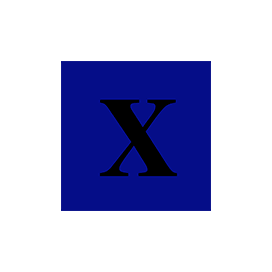
docxtemplater
צור Word DOCX מתבניות באמצעות JavaScript
ספריית JavaScript ליצירה, שינוי והמרה של קובצי Microsoft® Word DOCX.
מה זה docxtemplater?
docxtemplater היא ספריית JavaScript בקוד פתוח המסייעת ביצירה ועריכה של קובצי Word DOCX מתבנית. ספריית Word JavaScript מאפשרת למשתמשים להתאים אישית מסמכים שנוצרו באמצעות Word עצמו. הספרייה קלה לטיפול ואינה דורשת מיומנויות טכניות כלשהן כדי לערוך תבנית וורד. הספרייה סיפקה גם מספר מודולים לפונקציונליות ספציפית.
ספריית docxtemplater תומכת במספר תכונות הקשורות ליצירה וטיפול בקובץ DOCX כגון הוספת תמונות, הוספת טקסט מעוצב במסמך Word, הוספת כותרות עליונות/תחתונות, החלפת תמונה בכל מאפיינים קיימים, יצירת טבלאות, הוספת טקסט בסימן מים, עדכון שולי עמודים, הוספת הערות שוליים למסמך ועוד הרבה יותר.
כיצד להתקין docxtemplater?
הדרך המומלצת והקלה ביותר להתקין את docxtemplater היא באמצעות npm. אנא השתמש בפקודה הבאה להתקנה חלקה.
התקן את docxtemplater דרך npm
npm install docxtemplater pizzip
צור Word DOCX באמצעות JavaScript
ספריית docxtemplater מסייעת ביצירת מסמכי DOCX באפליקציית Node.js כמו גם בדפדפן בקלות. זה גם מאפשר לשנות את מסמכי ה-DOCX הקיימים כדי להוסיף טבלאות, תמונות, טקסט, פסקאות ועוד.
עדכון מספר DOCX באמצעות JavaScript
const PizZip = require("pizzip");
const Docxtemplater = require("docxtemplater");
const fs = require("fs");
const path = require("path");
// Load the docx file as binary content
const content = fs.readFileSync(
path.resolve(__dirname, "input.docx"),
"binary"
);
const zip = new PizZip(content);
const doc = new Docxtemplater(zip, {
paragraphLoop: true,
linebreaks: true,
});
// Render the document (Replace {first_name} by John, {last_name} by Doe, ...)
doc.render({
first_name: "John",
last_name: "Doe",
phone: "0652455478",
});
const buf = doc.getZip().generate({
type: "nodebuffer",
compression: "DEFLATE",
});
// buf is a nodejs Buffer, you can either write it to a
// file or res.send it with express for example.
fs.writeFileSync(path.resolve(__dirname, "output.docx"), buf);
הוסף ונהל טבלאות במסמכי Word
ספריית docxtemplater מאפשרת למפתחי תוכנה ליצור טבלה עם כמה שורות של קוד JavaScript. הספרייה כללה מספר שיטות ליצירה וניהול של טבלאות במסמך כמו יצירת טבלאות מאפס, יצירת טבלאות לולאה אנכית או על ידי העתקת תאים, מיזוג תאים של טבלה, הוספת שורות ועמודות, הגדרת רוחב לשורות ועמודות. וכן הלאה.
הוסף הערת שוליים למסמכי Word
ספריית docxtemplater החינמית כוללת תמיכה בהוספת הערות שוליים למסמך DOCX Word. הספרייה נותנת שליטה מלאה על התאמה אישית של הערות השוליים. ניתן להוסיף מספרים בכתב עילי ולהחיל סגנונות שונים על תוכן הערת השוליים בקלות.
הוספת הערות למסמכים באמצעות JavaScript
const imageOpts = {
getProps: function (img, tagValue, tagName) {
/*
* If you don't want to change the props
* for a given tagValue, you should write :
*
* return null;
*/
return {
rotation: 90,
// flipVertical: true,
// flipHorizontal: true,
};
},
getImage: function (tagValue, tagName) {
return fs.readFileSync(tagValue);
},
getSize: function (img, tagValue, tagName) {
return [150, 150];
},
};
const doc = new Docxtemplater(zip, {
modules: [new ImageModule(imageOpts)],
});
הוסף ושנה תמונות ב-DOCX
ספריית הקוד הפתוח docxtemplater מעניקה למתכנתי תוכנה את הכוח להכניס תמונות בתוך מסמך Word. הספרייה מאפשרת הגדרת רוחב וגובה התמונה, יישור תמונות, הוספת כיתוב לתמונות, שימוש בביטויים זוויתיים לקביעת גדלי תמונה וכדומה. אתה יכול גם לאחזר נתוני תמונה מכל מקור נתונים כגון נתוני base64, מערכת קבצים, כתובת URL ותמונה מאוחסנת של Amazon S3. תכונה נהדרת אחת של הספרייה היא שאתה יכול להימנע מתמונות גדולות מהמיכל שלהן.
תמונות רוטטות וזריקות דרך JavaScript
const imageOpts = {
getProps: function (img, tagValue, tagName) {
/*
* If you don't want to change the props
* for a given tagValue, you should write :
*
* return null;
*/
return {
rotation: 90,
// flipVertical: true,
// flipHorizontal: true,
};
},
getImage: function (tagValue, tagName) {
return fs.readFileSync(tagValue);
},
getSize: function (img, tagValue, tagName) {
return [150, 150];
},
};
const doc = new Docxtemplater(zip, {
modules: [new ImageModule(imageOpts)],
});