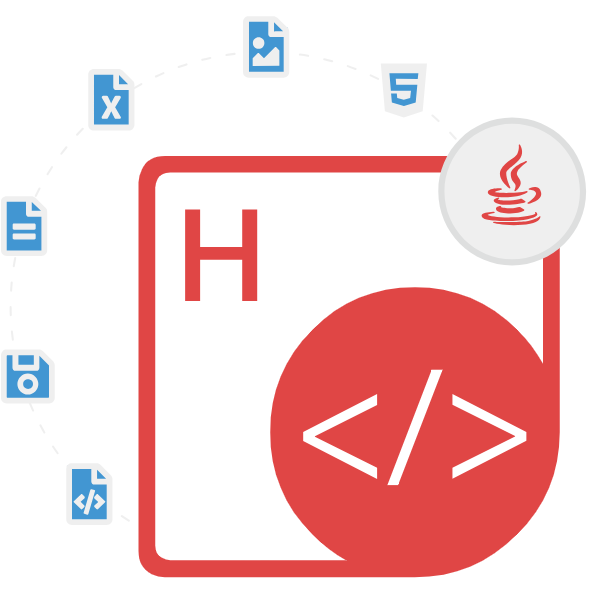
Aspose.HTML Cloud SDK for C++
C++ Cloud SDK to Generate, Edit & Render HTML Files
C++ REST HTML Library to Create, Edit, Parse, Render, remove & Convert HTML Files to EPUB, PDF & Raster Images (PNG, GIF, JPEG, BMP) Format in the Cloud.
Aspose.HTML Cloud SDK for C++ is a powerful tool that enables software developers to process HTML documents seamlessly in the cloud without any external dependencies. Aspose.HTML is a trusted name in the industry, known for its extensive range of APIs and SDKs that simplify document manipulation across various platforms. It allows programmers to effortlessly integrate HTML processing capabilities into their C++ applications, eliminating the need for complex manual coding and infrastructure management.
With the Aspose.HTML Cloud SDK for C++, software professionals can leverage the cloud-based functionalities of Aspose.HTML to efficiently create, convert, modify, and extract data from HTML files with ease. There are several other important features part of the library, such as HTML Parsing and Manipulation, HTML to PDF Conversion, HTML to Image Conversion, Extracting HTML Data, HTML Validation support, support for cloud storage, HTML fragment extraction, Populate HTML document template with data, and many more.
Aspose.HTML Cloud SDK for C++ is compatible with major platforms, including Windows, macOS, and Linux. The SDK interacts with Aspose.HTML Cloud using a RESTful API, providing a standardized and intuitive way to access HTML manipulation features. With a straightforward integration process and comprehensive documentation, developers can quickly incorporate the SDK into their C++ applications, saving valuable time and effort. With its cross-platform compatibility, RESTful API integration, and scalability advantages, the SDK offers a reliable solution for C++ developers seeking to incorporate HTML manipulation functionality into their applications.
Getting Started with Aspose.HTML Cloud SDK for C++
The recommend way to install Aspose.HTML Cloud SDK for C++ via NuGet. Please use the following command for a smooth installation.
Install Aspose.HTML Cloud SDK for C++ via NuGet
NuGet\Install-Package aspose.html.cloud.v143 -Version 22.12.1
You can download the library directly from Aspose.HTML Cloud SDK for C++ product page
Convert HTML to Image via C++ REST API
Aspose.HTML Cloud SDK for C++ has included very useful features for converting HTML documents to some popular images file formats. Software developers can convert HTML documents to various image formats such as JPEG, PNG, BMP, and TIFF with just a couple of lines C++ code. This functionality is valuable when generating thumbnail previews, capturing website screenshots, or any other scenario where HTML content needs to be rendered as an image.
HTML to PDF Conversion via RST API
Aspose.HTML Cloud SDK for C++ makes it easy for software developers to load and convert HTML documents to PDF format with ease. This feature is particularly useful when generating reports, invoices, or any other printable documents from HTML sources. The conversion process is highly customizable, enabling software developers to control various aspects such as page size, orientation, margins, headers, footers, and so on. The SDK handles the intricate process of converting complex HTML layouts into PDF documents while preserving the original formatting and styles.
Convert an HTML Document to PDF via C++ API
#include
#include
#include
int main()
{
// Create an instance of the API client
std::shared_ptr apiClient =
std::make_shared();
apiClient->setAppKey(L"APP_KEY");
apiClient->setAppSid(L"APP_SID");
apiClient->setBaseUrl(L"https://api.aspose.cloud");
// Create a conversion request
std::shared_ptr request =
std::make_shared();
request->setSourceUrl(L"https://example.com/input.html");
request->setFormat(L"pdf");
try
{
// Convert HTML to PDF
std::shared_ptr result =
apiClient->getHTMLApi()->postConvertDocument(request);
// Download the converted PDF file
apiClient->downloadFile(result->getPdf()->getHref(), L"output.pdf");
std::cout << "HTML to PDF conversion successful." << std::endl;
}
catch (std::exception& ex)
{
std::cout << "Error: " << ex.what() << std::endl;
}
return 0;
}
Extract HTML Fragment via C++ Cloud API
Aspose.HTML Cloud SDK for C++ has included complete support for extracting a particular sections or fragments of an HTML documents inside cloud-based C++ applications. This feature is very useful when dealing with large HTML files and needing to retrieve only a portion of the content for further processing or display. The SDK also supports extracting structured data from HTML documents using XPath queries or CSS selectors. This functionality proves valuable when working with web scraping, data mining, or content extraction tasks. The following code example demonstrates how to extract a specific sections or fragments from HTML documents using the C++ SDK.
How to Extract an HTML Fragment via C++ REST API?
#include
#include
#include
using namespace std;
using namespace aspose::html::cloud::api;
using namespace aspose::html::cloud::model;
int main() {
// Configure Aspose.HTML Cloud credentials
aspose::html::cloud::AsposeHtmlCloudConfig config;
config.set_client_id("your_client_id");
config.set_client_secret("your_client_secret");
// Create an instance of the HTMLApi
aspose::html::cloud::api::HTMLApi htmlApi(config);
// Set the source HTML file for extraction
std::string name = "sample.html";
std::string folder = "html_folder";
// Set the CSS selector for the HTML fragment to be extracted
std::string selector = "#myFragment";
try {
// Extract the HTML fragment
std::shared_ptr response = htmlApi.getHtmlFragmentByCSSSelector(name, folder, selector);
// Save the extracted HTML fragment to a file
std::ofstream outputFile("output.html");
outputFile << response->getFragmentContent();
outputFile.close();
std::cout << "HTML fragment extracted successfully." << std::endl;
} catch (const aspose::html::cloud::ApiClientException& ex) {
std::cout << "Error occurred: " << ex.get_message() << std::endl;
}
return 0;
}
EHTML Validation via C++ Cloud API
Aspose.HTML Cloud SDK for C++ supports HTML validation against standard HTML specifications, allowing software developers to ensure compliance and integrity of HTML documents. These HTML validation will be of great help for software developers by ensuring that their HTML documents comply with industry standards and best practices. It identifies and reports any errors or issues in the HTML code, helping maintain the quality of the processed documents.