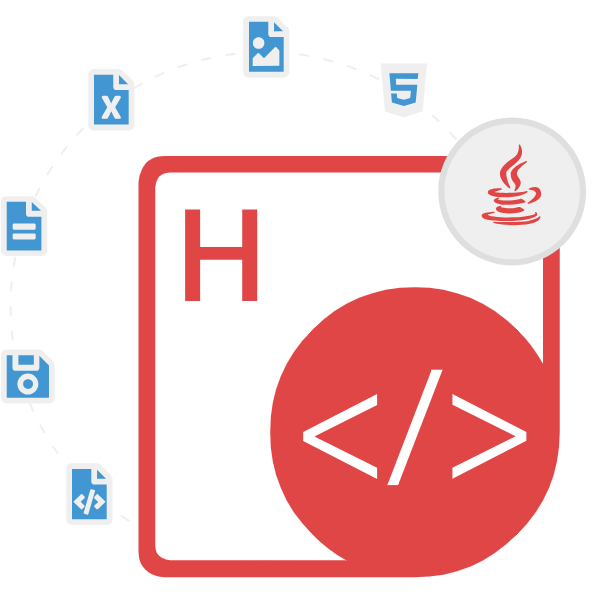
Aspose.HTML for Java
Java HTML API to Create, Edit & Convert HTML Files
Open Source Java HTML Library to Create, Edit, Parse, Load, Delete & Convert HTML Files to XPS, PDF & Raster Images (PNG, GIF, JPEG, BMP) Format using Java API.
In the world of software development, efficient and reliable libraries play a crucial role in simplifying complex tasks. When it comes to handling HTML files and performing various operations on them, Aspose.HTML for Java emerges as a versatile and powerful library that provides an extensive set of features, enabling software developers to create, modify, load, parse, manipulate, and convert HTML documents effortlessly. An active and supportive community of developers and extensive documentation provide valuable resources for getting started, troubleshooting issues, and discovering best practices.
Aspose.HTML for Java is very easy to handle and built with performance and reliability in mind. The library is optimized to handle large HTML files efficiently, ensuring smooth processing even with complex documents with just a couple of lines of Java code. It also offers a variety of performance tuning options, such as enabling or disabling JavaScript execution during rendering, to cater to different use cases. Furthermore, the library undergoes rigorous testing and continuous improvement, ensuring high-quality output and minimizing the risk of errors or inconsistencies.
Aspose.HTML for Java seamlessly integrates with other popular Java libraries and frameworks, making it an ideal choice for various development scenarios. Whether software developers are working with Spring, JavaFX, Apache POI, or any other Java ecosystem, Aspose.HTML for Java provides smooth integration, allowing them to leverage its HTML manipulation capabilities within their existing projects. With its powerful parsing, DOM manipulation, HTML-to-PDF conversion, rendering, CSS styling, and integration capabilities, the library can be a great choice for software developers to efficiently work with HTML content inside their Java applications.
Getting Started with Aspose.HTML for Java
The recommend way to install Aspose.HTML for Java via Maven repository. You can easily use the API directly in your Maven Projects with simple configurations
Add Aspose.HTML for Java Maven Dependency
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-html</artifactId>
<version>20.9.1</version>
<version>jdk16</version>
</dependency>
You can download the library directly from Aspose.HTML for Java product page
Export HTML to PDF using Java
One of the notable features of Aspose.HTML for Java is its ability to convert HTML documents to PDF format with just a couple of lines of Java code. This feature is particularly useful when generating reports or sharing web content in a standardized format. The library provides fine-grained control over the conversion process, allowing developers to customize page settings, apply CSS styles, embed fonts, and even convert specific sections of the HTML document. The following code shows how software developers can convert HTML file to PDF using Java code.
Convert HTML file to PDF using Java API
// Load HTML document
HTMLDocument document = new HTMLDocument("template.html");
// Convert HTML to PDF
Converter.convertHTML(document, new PdfSaveOptions(), "output.pdf");
Create & Manipulate HTML Files via Java
Aspose.HTML for Java has included the ability to create new HTML documents from the scratch inside Java applications. Software developers programmatically read, parse, modify, and remove HTML in the document. The library provides a rich set of methods and properties to modify the HTML structure, add or remove elements, update attributes, and manipulate CSS styles. With this flexibility, developers can automate tedious tasks, dynamically generate HTML content, or enhance existing documents with ease. Additionally, the library supports HTML sanitization, ensuring the output conforms to specified standards and security requirements.
Create an Empty HTML Document via Java API
// Initialize an empty HTML Document
com.aspose.html.HTMLDocument document = new com.aspose.html.HTMLDocument();
try {
// Save the HTML document to a disk
document.save("create-empty-document.html");
} finally {
if (document != null) {
document.dispose();
}
}
HTML Rendering and Extraction
Aspose.HTML for Java enables Software developers to render HTML documents to various output formats, such as images, PDF, DOCX, XPS, SVG and many more. This functionality proves valuable when creating thumbnail previews, generating images from HTML templates, or converting HTML to other presentation formats. Additionally, the library allows selective extraction of specific HTML elements, such as tables or images, which can be useful for data extraction or content analysis purposes. The following example shows how to render and extract HTML using Java API.
HTML Rendering and Extraction using Java API
import com.aspose.html.dom.Document;
import com.aspose.html.rendering.HtmlRenderer;
import com.aspose.html.rendering.pdf.PdfDevice;
import com.aspose.html.rendering.pdf.PdfRenderingOptions;
public class HtmlRenderingExample {
public static void main(String[] args) {
// Load the HTML document
Document document = new Document("input.html");
// Render HTML to PDF
PdfRenderingOptions options = new PdfRenderingOptions();
options.getPageSetup().setAnyPage(new com.aspose.html.drawing.Page(new com.aspose.html.drawing.Size(800, 600)));
PdfDevice device = new PdfDevice("output.pdf");
HtmlRenderer renderer = new HtmlRenderer();
renderer.render(device, document, options);
System.out.println("HTML rendered to PDF successfully!");
// Extract HTML content
String htmlContent = document.getBody().getInnerHTML();
System.out.println("Extracted HTML content:\n" + htmlContent);
}
}
Efficient HTML Parsing via Java API
Parsing HTML documents can be a challenging task, especially when dealing with complex structures or poorly formatted code. Aspose.HTML for Java provides robust HTML parsing capabilities, allowing developers to extract data, navigate the document tree, and manipulate elements effortlessly. The library ensures accurate parsing, even with malformed HTML, by employing advanced algorithms that handle common parsing challenges.
Perform HTML Document Parsing using Java API
import com.aspose.html.HTMLDocument;
import com.aspose.html.IHTMLDocument;
import com.aspose.html.IHTMLHtmlElement;
public class HTMLParsingExample {
public static void main(String[] args) {
// Load the HTML document
HTMLDocument document = new HTMLDocument("input.html");
// Access the root HTML element
IHTMLHtmlElement rootElement = document.getRootElement();
// Print the inner text of the body element
System.out.println("Body content:");
System.out.println(rootElement.getBody().getTextContent());
// Access and print the values of specific elements
System.out.println("Links:");
document.querySelectorAll("a").forEach(element -> {
System.out.println("URL: " + element.getAttribute("href"));
System.out.println("Text: " + element.getTextContent());
});
// Manipulate the document structure
IHTMLHtmlElement newElement = document.createElement("div");
newElement.setTextContent("This is a new element");
rootElement.appendChild(newElement);
// Save the modified document
document.save("output.html");
}
}