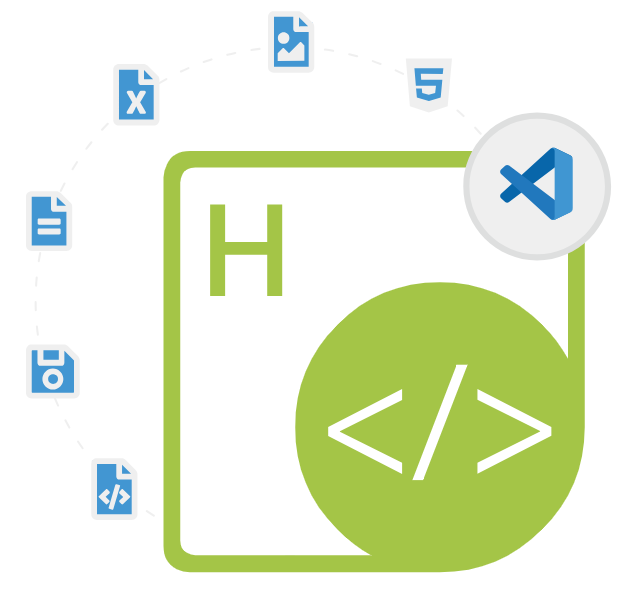
Aspose.HTML for .NET
C# .NET HTML API to Parse, Manipulate & Convert HTML Files
A Robust C# .NET HTML API that enables software developers to to load, analyze & edit web pages and convert to/from HTML, XHTML, MHTML, SVG, Markdown, PDF, DOCX, EPUB & more.
In today's digital landscape, HTML (Hypertext Markup Language) plays a crucial role in web development and web content creation. Being able to manipulate and process HTML documents programmatically is essential for many leading applications as well as software professionals. Aspose.HTML for .NET is a robust library that provides software developers with a comprehensive set of tools for handling various tasks related to HTML files without any external dependencies. The API is very easy to handle and empowers software developers to manipulate, parse, and render HTML documents using .NET languages such as C#, VB.NET, and ASP.NET.
Aspose.HTML for .NET is a feature-rich library developed by Aspose team that enables computer programmers to load, parse, manipulate, modify, and convert HTML files inside their .NET applications. There are several important features part of the library, such as generating HTML documents programmatically. HTML Parsing, updating a specific HTML element, creating new HTML elements dynamically, HTML conversion to other supported file formats, extracting specific data from HTML pages, generating printable reports, archiving web content, and many more.
Aspose.HTML for .NET offers a very powerful conversion feature that enables software developers to convert HTML files from/to some leading file formats such as HTML, XHTML, MHTML, SVG, Markdown, PDF, XPS, DOCX, EPUB, PNG, TIFF, JPEG, BMP, and many more. This can be particularly useful for generating reports, invoices, or any document that requires precise layout and styling. With support for HTML parsing, extraction, manipulation, rendering, and conversion, the library provides a comprehensive solution for handling HTML-related tasks. Whether you need to generate PDF reports, transform HTML content, or extract data from HTML documents, Aspose.HTML for .NET is a valuable tool to have in your development toolkit.
Getting Started with Aspose.HTML for .NET
The recommend way to install Aspose.HTML for .NET is using NuGet. Please use the following command for a smooth installation.
Install Aspose.HTML for .NET via NuGet
NuGet\Install-Package Aspose.Font.Cpp -Version 23.5.0
You can download the library directly from Aspose.HTML for .NET product page
Create & Manipulate HTML File via .NET
Aspose.HTML for .NET makes it easy for software professional to generate a HTML document programmatically from scratch inside their C# applications. Software developers can perform various operations on HTML documents such as add or modify HTML elements, work with attributes, manage content, load HTML file via URL, Load HTML from a file and many more. The library provides a rich set of methods and properties to handle text formatting, tables, images, hyperlinks, CSS styles, and more. The following example shows how to create a new HTML document inside .NET applications.
Create a New HTML Document using C# Code
using System.IO;
using Aspose.Html;
...
// Prepare an output path for a document saving
string documentPath = Path.Combine(OutputDir, "create-new-document.html");
// Initialize an empty HTML document
using (var document = new HTMLDocument())
{
// Create a text element and add it to the document
var text = document.CreateTextNode("Hello World!");
document.Body.AppendChild(text);
// Save the document to a disk
document.Save(documentPath);
}
HTML to PDF Conversion via C# API
Aspose.HTML for .NET has included a very powerful converter that allows software developers to convert HTML documents to PDF and other supported file formats with ease. The library allows seamless conversion of HTML documents to PDF format without any dependencies. The library handles the conversion process accurately, preserving the original layout, formatting, and styling of the HTML content. This feature is particularly useful for generating PDF reports, invoices, and other documents from HTML templates. The following example shows how to convert an HTML document to PDF files using .NET code.
How to Convert HTML to PDF using C# .NET API?
using System.IO;
using Aspose.Html.Converters;
using Aspose.Html.Saving;
...
// Invoke the ConvertHTML() method to convert the HTML code to PDF
Converter.ConvertHTML(@"Hello, World!
", ".", new PdfSaveOptions(), Path.Combine(OutputDir, "convert-with-single-line.pdf"));
Render HTML Documents via C# API
The Aspose.HTML for .NET library has included built-in functionality to render HTML documents directly to the screen or an image, allowing software developers to visualize the HTML content within their applications. It helps users in generating website screenshots, creating visual representations of web pages, or generating previews of HTML content. This feature is especially valuable in scenarios where real-time HTML rendering is required, such as web browsers, email clients, or content management systems. The following example shows how to render SVG files to other file formats with ease.
How to Render SVG File to PDF using C# API?
using System.IO;
using Aspose.Html;
using Aspose.Html.Rendering;
using Aspose.Html.Rendering.Pdf;
using Aspose.Html.Rendering.Pdf.Encryption;
...
// Initialize an SVG document from the file
using var document = new SVGDocument(Path.Combine(DataDir, "shapes.svg"));
// Create an instance of SVG Renderer
using var renderer = new SvgRenderer();
// Prepare a path to save the converted file
string savePath = Path.Combine(OutputDir, "merge-svg.pdf");
// Create the instance of the PdfRenderingOptions class and set a custom page size
var options = new PdfRenderingOptions();
options.PageSetup.AnyPage = new Page(new Size(600, 500));
// Create an instance of PdfDevice class
using var device = new PdfDevice(options, savePath);
// Render SVG to PDF
renderer.Render(device, document);
HTML Parsing and Manipulation
Aspose.HTML for .NET offers efficient HTML parsing capabilities, allowing software developers to load HTML documents and navigate the document structure using the Document Object Model (DOM). The DOM enables easy access to HTML elements, attributes, and content, facilitating seamless manipulation and extraction of data from HTML files. The library, helps users to extract specific elements, modify their attributes and content, and create new HTML elements dynamically with ease.
HTML Validation and Cleaning
The Aspose.HTML for .NET library has provided a very useful feature for validation and cleaning that help ensure the integrity and compliance of HTML documents. Software Developers can validate HTML files against various standards, such as HTML5, XHTML, or custom schemas inside their C# applications. They can also clean and sanitize HTML documents by removing unwanted or potentially malicious elements, ensuring a safe and reliable processing environment.