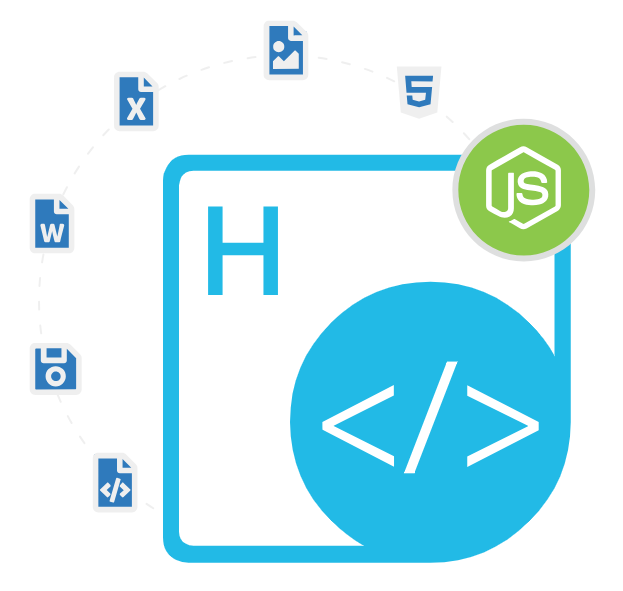
Aspose.HTML Cloud SDK for Node.js
Node.js HTML API to Generate & Convert HTML Files
A Powerful Node.js HTML Library to Create, Edit, Parse, Load, Manipulate & Convert HTML Files to XPS, PDF & Raster Images (PNG, GIF, JPEG, BMP) Format via Node.js API.
Aspose.HTML Cloud SDK for Node.js is a robust, cloud-based platform that enables software developers to load and process HTML documents programmatically. It is part of Aspose's comprehensive cloud platform, designed to offer a range of document processing capabilities with ease of use, scalability, and efficiency in mind. Whether it’s converting, parsing, or rendering HTML files, the SDK provides an efficient and flexible solution for managing HTML in web applications. The SDK is designed for Node.js developers, offering seamless integration with cloud APIs for various document-processing needs.
Aspose.HTML Cloud SDK operates on a secure cloud infrastructure, which ensures data security and scalability. The SDK is very easy to use and supports multiple HTML-related functions, such as creating HTML files from the scratch, loading and manipulating HTML pages, converting HTML files to various formats (PDF, DOCX, PNG, SVG, and more), extracting text and images from web pages and many more. These capabilities enable software developers to integrate rich document processing features into their applications without worrying about the underlying complexities. The SDK is built on REST APIs, making it easy to integrate into existing Node.js applications.
Aspose.HTML Cloud SDK for Node.js is cloud-based, meaning software developers can build applications that work across multiple platforms. Users can access the HTML-related features from any device, be it a desktop or mobile, using a web interface powered by the Node.js backend. Instead of writing complex code to handle HTML processing, developers can use the SDK’s predefined methods to perform actions such as document read and conversion, HTML manipulation, content extraction and more. By integrating the SDK into reporting tools, developers can automate the conversion of HTML reports into print-ready formats, making the document generation process more efficient. With these rich features and easy integration, it is a great choice to build robust applications that handle complex document processing tasks effortlessly.
Getting Started with Aspose.HTML Cloud SDK for Node.js
The recommended way to install Aspose.HTML Cloud SDK for Node.js is using NPM. Please use the following command for a smooth installation.
Install Aspose.HTML Cloud SDK for Node.js via NPM
npm install @asposecloud/aspose-html-cloud --save
You can download the library directly from Aspose.HTML Cloud SDK for Node.js product page
Converting HTML to PDF in Node.js Apps
One of the most common tasks in web development is converting HTML document content to PDF file. Aspose.HTML Cloud SDK for Node.js provides a straightforward API to handle this conversion process, which is useful in creating PDFs from dynamically generated HTML web pages. The following example demonstrates how to convert an HTML file into a PDF inside Node.js applications. In this example, the convertHtmlToPdf method takes an HTML file, converts it to PDF, and saves it to the specified output location.
How to Convert an HTML file into a PDF via Node.js API?
const convertHtmlToPdf = async () => {
try {
const htmlFilePath = 'path/to/sample.html';
const outputPdfPath = 'output/sample.pdf';
const result = await htmlApi.convertHtmlToPdf({
file: htmlFilePath,
output: outputPdfPath
});
console.log('HTML successfully converted to PDF:', result);
} catch (error) {
console.error('Error converting HTML to PDF:', error);
}
};
convertHtmlToPdf();
Extract Text from HTML via Node.js SDK
Web scraping or data extraction from HTML documents is a common requirement in many applications. Aspose.HTML Cloud SDK for Node.js can help software developers in extracting a specific data from HTML files with just a couple of lines of code, such as text content, images, hyperlinks, metadata and so on. The following example shows how to extracts text from an HTML file inside Node.js environment. This feature is particularly useful when building content analysis tools, search engines, or even web crawlers that need to process large amounts of HTML content.
How to Extract Text from an HTML File inside Node.js Apps?
const extractTextFromHtml = async () => {
try {
const inputFile = 'path/to/sample.html';
// Extract text from HTML document
const result = await htmlApi.extractText({
file: inputFile
});
console.log('Extracted text:', result.text);
} catch (error) {
console.error('Error during text extraction:', error);
}
};
extractTextFromHtml();
HTML to Image Conversion in Node.js
Another crucial feature of the Aspose.HTML Cloud SDK for Node.js is converting HTML files to image formats, such as PNG, JPEG, BMP, GIF and more inside Node.js environment. This functionality is useful when generating visual snapshots of web content or when working with dynamic content like diagrams, charts, and other visual elements. This method allows developers to convert HTML content into high-quality images, which is ideal for capturing snapshots of dynamically generated web content. Here’s is an example the shows how to convert an HTML file to PNG inside Node.js applications.
How to Convert HTML File to PNG Images inside Node.js Apps?
const convertHtmlToImage = async () => {
try {
const htmlFilePath = 'path/to/sample.html';
const outputImagePath = 'output/sample.png';
const result = await htmlApi.convertHtmlToImage({
file: htmlFilePath,
output: outputImagePath,
format: 'png'
});
console.log('HTML successfully converted to PNG:', result);
} catch (error) {
console.error('Error converting HTML to image:', error);
}
};
Generating Reports from HTML in Node.js
For software developers building reporting systems, Aspose.HTML Cloud SDK for Node.js can convert HTML reports into various formats such as PDF, DOCX, or XPS inside Node.js applications. This enables users to export their reports in the desired format with minimal effort. By integrating the SDK into reporting tools, developers can automate the conversion of HTML reports into print-ready formats, making the document generation process more efficient.