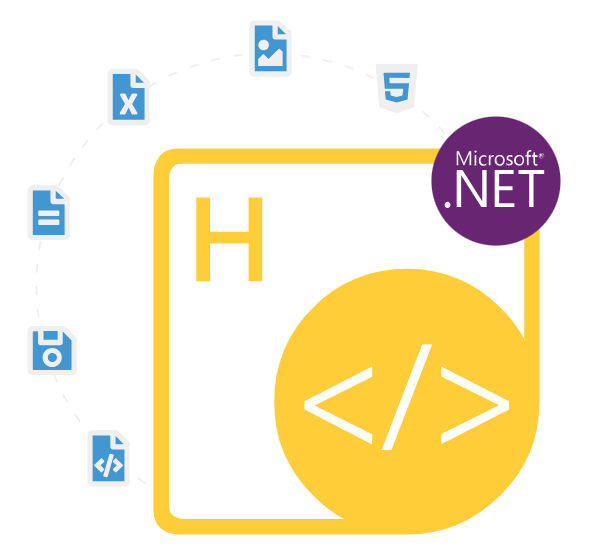
Aspose.HTML for Python via .NET
Python HTML API to Create & Convert HTML to PDF, XPS, DOCX
Top Python HTML Library Enables Developers to Load, Parse, Create, Render, Edit, and Convert HTML Files to PDF, XPS, DOCX, PNG & JPEG. It supports CSS, JavaScript & Modern HTML Standards
Aspose.HTML for Python via .NET is a Python API powered by .NET, designed specifically to let developers load, parse, render, edit, and convert HTML documents with precision. It provides extensive support for CSS, JavaScript, and modern HTML standards, making it ideal for building applications that generate, transform, or display HTML content. There are several important features part of the library, such as creating HTML documents from scratch, loading HTML from files as well as stream, loading HTML directly from a URL, parsing HTML files and modifying the Document Object Model (DOM) dynamically, HTML to PDF conversion, rendering HTML to popular image formats such as JPEG, PNG, BMP, and TIFF, converting HTML to EPUB, and many more.
Aspose.HTML for Python via .NET acts as a "headless browser," providing an extensive API that allows developers to manage HTML and related formats (XHTML, MHTML, SVG, Markdown, EPUB) without needing any external software or UI. This makes it an ideal choice for a wide array of applications, from web scraping and content generation to document conversion and report building. The API design is intuitive, especially for those familiar with web standards and DOM manipulation. Aspose.HTML offers rich configuration options for rendering, such as setting page size, margins, DPI, and more. This is especially useful for document publishing or archiving systems. Whether you're automating conversions, generating reports, archiving content, or building a full-fledged editor, this API gives you the tools to do it cleanly and efficiently.
Getting Started with Aspose.HTML for Python via .NET
The recommend way to install Aspose.HTML for Python via .NET via PyPi. You can easily use the API directly in your Maven Projects with simple configurations.
Install Aspose.HTML Cloud SDK for PHP via PyPi
pip install aspose-html-net
You can download the library directly from Aspose.HTML for Python via .NET product page
Load & Parse HTML from Various Sources
Aspose.HTML for Python via .NET has provided complete support for loading and parsing HTML documents programmatically inside Python applications. The API lets Python developers to load HTML documents from files, URLs, or streams, making it highly versatile in web content processing. The following code snippets loads an HTML page from the internet and prints its title—ideal for web scraping or content analysis.
How to Loads an HTML Page from the Internet and Prints its Title via Python API?
from aspose.html import HTMLDocument
# Load HTML document from a web URL
document = HTMLDocument("https://example.com")
print(document.title)
Convert HTML to PDF, XPS, DOCX via Python API
One of the flagship features Aspose.HTML for Python via .NET is its advanced document conversion engine. The API has provided complete support for reading and converting HTML to PDF, XPS, DOCX, images & various other file format using Python API. Here is a powerful example that allows software developers to load an HTML file and converts it into a high-quality PDF using the built-in rendering engine.
How to Load and Convert HTML File to high-quality PDF via Python API?
from aspose.html import HTMLDocument
from aspose.html.saving import PdfSaveOptions
from aspose.html.rendering.pdf import PdfDevice
document = HTMLDocument("input.html")
options = PdfSaveOptions()
device = PdfDevice("output.pdf")
device.render(document)
Dynamic Report Generation Systems
Need to generate dynamic reports from data? Aspose.HTML for Python via .NET allows you to take HTML templates, bind them with data, and convert them to print-ready PDFs or images inside Python applications. Software developers can create a templating engine where placeholders in the HTML are replaced by real data, rendered to PDF, and emailed to clients—all in Python.
Edit and Manipulate HTML Elements via Python
Aspose.HTML provides a complete DOM (Document Object Model) API, allowing you to edit HTML nodes, modify attributes, or create new elements programmatically. The library fully supports external and inline CSS styling and executes embedded JavaScript, ensuring that the rendered output stays faithful to how it would appear in a browser. You can build dynamic web documents or automate changes across multiple files using these methods. The following example shows how developers can apply CSS styles dynamically to HTML elements or extract existing style inside Python apps.
How Apply CSS Styles Dynamically to HTML Elements or Extract Existing Styles via Python API?
import aspose.html as html
import aspose.html.dom as dom
# Create a new HTML document
document = html.HTMLDocument()
# Create a paragraph element
paragraph = document.create_element("p")
paragraph.text_content = "Styled with Aspose.HTML!"
# Apply CSS style
paragraph.style.color = "blue"
paragraph.style.font_size = "20px"
# Append to the document body
document.body.append_child(paragraph)
# Save the document
document.save("styled.html")