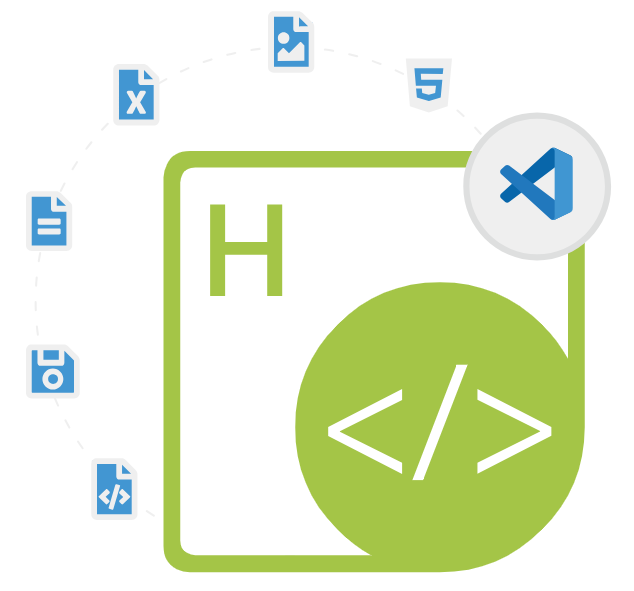
Aspose.HTML Cloud SDK for Python
Python HTML SDK to Create, Edit & Convert HTML Documents
A Python HTML SDK that enables Programmers to Develop and Manage cloud-based HTML Generation, Parsing, Manipulation and Conversion applications via Python REST API.
In the fast-paced world of software development, leveraging powerful tools and libraries can significantly streamline the process of creating, editing, and manipulating various types of documents. Aspose.HTML Cloud SDK for Python is one such tool that empowers software developers and engineers to work with HTML documents effortlessly, enabling them to focus on creating exceptional applications rather than dealing with intricate HTML-related intricacies.
Aspose.HTML Cloud SDK for Python is a comprehensive set of tools and libraries that enable software developers to integrate HTML processing capabilities into their Python applications. This SDK provides a wide array of features, such as parsing, rendering, editing, printing, and converting HTML documents to various formats, making it an essential asset for projects that require precise handling of HTML content. It offers an intuitive interface that abstracts the complexities of HTML handling, allowing software developers to focus on building their applications.
Aspose.HTML Cloud SDK for Python seamlessly integrates with other popular libraries and frameworks, making it an ideal choice for various development scenarios. By simplifying complex tasks like parsing, rendering, and conversion, this SDK empowers developers to create more robust and feature-rich applications, ultimately enhancing the end-user experience. Its comprehensive feature set, ease of use, and robust functionality make it an excellent choice for tasks ranging from simple HTML parsing to complex content management. By integrating this SDK into your Python applications, you can streamline HTML-related processes and enhance the efficiency of your projects.
Getting Started with Aspose.HTML Cloud SDK for Python
The recommend way to install Aspose.HTML Cloud SDK for Python via PyPi. You can easily use the API directly in your Maven Projects with simple configurations.
Install Aspose.HTML Cloud SDK for PHP via PyPi
pip install asposehtmlcloud
You can download the library directly from Aspose.HTML Cloud SDK for Python product page
HTML to Image/PDF Conversion
Converting HTML to PDF or image formats is a common requirement in applications dealing with document generation. Aspose.HTML Cloud SDK for Python has included complete support for converting HTML documents to some leading file formats. The SDK facilitates this conversion process, allowing software developers to generate high-quality PDFs, XPS, DOCX, MHTML, as well as images like JPEG, BMP, PNG, TIFF, GIF and many more from HTML content. The SDK supports conversion of whole document as well as an online HTML page inside Python applications. The following example demonstrates how software developers can converts the HTML document from storage to PDF using Python code.
How to Convert the HTML Document from Storage to PDF via Python API?
name = "test1.html"
result_name = "putHtmlToPdf.pdf"
folder = "HtmlTestDoc"
out_path = folder + "/" + result_name
try:
# Convert document to pdf in storage
self.api.put_convert_document_to_pdf(
name, out_path=out_path, width=800, height=1000, left_margin=50, right_margin=100,
top_margin=150, bottom_margin=200, folder=folder, storage="")
# Download result
res = Helper.download_file(out_path)
save_file = Helper.dst + result_name
Helper.move_file(res, save_file)
except ApiException as ex:
print("Exception")
print("Info: " + str(ex))
raise ex
Parse & Render HTML Content
Aspose.HTML Cloud SDK for Python gives software developers the capability to load and parse an existing HTML document with just a couple of lines of Python code. Parsing HTML documents can be a daunting task due to the complexity of the markup language. The SDK abstracts away the complexities of HTML parsing, allowing software developers to effortlessly extract meaningful data from HTML documents. The following example shows how software developers can get all images from the HTML document inside Python applications.
How to Get All HTML Document Images using Python API?
name = "test3.html.zip"
try:
# Upload file to storage
res = Helper.upload_file(name)
self.assertTrue(len(res.uploaded) == 1)
self.assertTrue(len(res.errors) == 0)
# Get images from document on remote storage
res = self.api.get_document_images(name=name, storage="", folder=Helper.folder)
self.assertTrue(isinstance(res, str), "Error get images from document")
# Move to test folder
Helper.move_file(str(res), Helper.dst)
except ApiException as ex:
print("Exception")
print("Info: " + str(ex))
raise ex
HTML File Manipulation via Python API
The Aspose.HTML Cloud SDK for Python is a useful tool for software professionals who require HTML manipulation capabilities in their applications. It enables software developers to manipulate HTML documents programmatically. Software developers can programmatically modify HTML content, insert or remove elements, update attributes, and perform various transformations, making it an ideal solution for content management applications.
Web Scraping & XPath Navigation Support
Navigating and manipulating specific elements within an HTML document often requires the use of XPath expressions. The Aspose.HTML Cloud SDK for Python has included support for XPath navigation, making it easier to locate and manipulate elements within the document's structure. For projects involving data extraction from websites, the SDK can be used for web scraping tasks. You can parse and extract data from HTML pages, making it a valuable tool for collecting information from the web.
How to Get List of HTML Fragments Matching the Specified XPath Query via Python API?
# For complete examples and data files, please go to https://github.com/aspose-html-cloud/aspose-html-cloud-python
name = "test2.html.zip"
x_path = ".//p"
out_format = "plain"
try:
# Upload file to storage
res = Helper.upload_file(name)
self.assertTrue(len(res.uploaded) == 1)
self.assertTrue(len(res.errors) == 0)
# Get fragment document from remote storage
res = self.api.get_document_fragment_by_x_path(name=name, x_path=x_path, out_format=out_format,
storage="", folder=Helper.folder)
self.assertTrue(isinstance(res, str), "Error get fragment document from remote storage")
# Move to test folder
Helper.move_file(str(res), Helper.dst)
except ApiException as ex:
print("Exception")
print("Info: " + str(ex))
raise ex