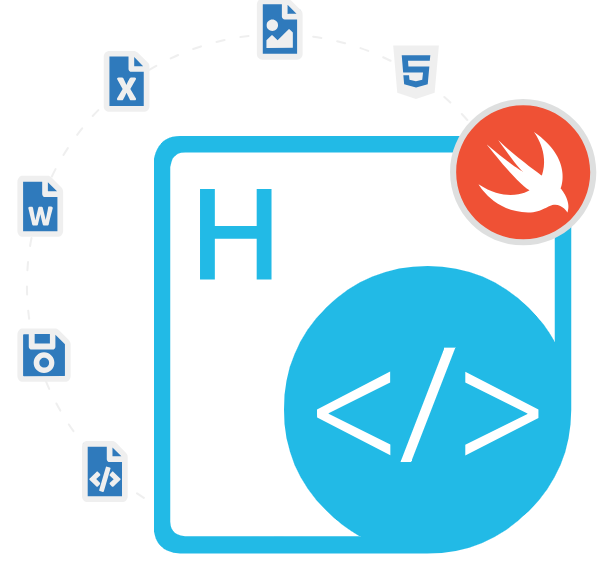
Aspose.HTML Cloud SDK for Swift
Swift HTML SDK to Create, Validate & Convert HTML Files
A Powerful HTML Processing API that Empowers Software Programmers to Create, Edit, Validate, Manipulate and Convert HTML Content to PDF & Images Format
In today's fast-paced digital world, generating and managing HTML documents is a common requirement for many businesses and software developers. To simplify this task and enhance the capabilities of Swift developers, Aspose has introduced the Aspose.HTML Cloud SDK for Swift. This powerful tool opens up a world of possibilities for manipulating HTML documents in the cloud, making it easier than ever to generate, convert, and extract content from HTML files. The library streamlines HTML document manipulation tasks, saving developers time and effort.
Aspose.HTML Cloud SDK for Swift is a software development kit that empowers Swift developers to work with HTML documents in the cloud. It provides a wide range of features, including HTML to PDF conversion, HTML to image conversion, and HTML content extraction. With this SDK, software professionals can automate the creation of PDFs from HTML templates, capture screenshots of web pages, and extract data from HTML documents effortlessly. The SDK is cloud-based, making it platform-agnostic. Developers can use it on various platforms, including iOS, macOS, and Linux, without worrying about compatibility issues.
The Aspose.HTML Cloud SDK for Swift SDK provides a user-friendly and intuitive interface, allowing software developers to work with HTML documents without extensive coding. It empowers software developers to harness the power of the cloud for HTML document manipulation. With features like HTML to PDF conversion, HTML to image conversion, and HTML content extraction, it offers a versatile toolkit for a wide range of applications. By leveraging this SDK, Swift developers can enhance productivity, reduce operational costs, and deliver high-quality HTML document processing solutions. Whether you're building web apps, mobile apps, or desktop applications, the Aspose.HTML Cloud SDK for Swift is a valuable tool in your development toolkit. Explore its capabilities and streamline your HTML document management processes today.
Getting Started with Aspose.HTML Cloud SDK for Swift SDK
The recommend way to install Aspose.HTML Cloud SDK for Swift is using GitHub. Please use the following command for a smooth installation.
Install Aspose.HTML for .NET via GitHub
Clone https://github.com/aspose-html-cloud/aspose-html-cloud-swift.git
You can download the library directly from Aspose.HTML Cloud SDK for Swift SDK product page
HTML to Word Documents Conversion via Swift
Aspose.HTML Cloud SDK for Swift gives software developers the power to load and convert HTML documents to Microsoft Office Word DOC and DOCX file formats. These are very popular documents formats and can encompass a wide range of data, including text, tables, raster and vector graphics, video, sounds and diagrams. The library allows developers to load an HTML document from storage location by its name, or via a URL or a local file on user’s drive. The following example shows how to convert a webpage to Word DOCX file format and save the result on a local drive.
How to Convert a Webpage to DOCX File using Swift Code?
import Alamofire
import Foundation
import XCTest
import AsposeHtmlCloud
static let fm = FileManager.default
let resultDir = fm.homeDirectoryForCurrentUser.appendingPathComponent("Documents/Aspose.HTML.Cloud.SDK.Swift/Tests/AsposeHtmlCloudTests/TestResult")
func fileExist(name: String) -> Bool {
return FileManager.default.fileExists(atPath: name)
}
ClientAPI.setConfig(
basePath: "https://api.aspose.cloud/v4.0",
authPath: "https://api.aspose.cloud/connect/token",
apiKey: "XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX",
appSID: "XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX",
debugging: true
)
let format = "docx"
let src = "https://example.com"
let dst = resultDir.appendingPathComponent("Output.\(format)").absoluteString
let expectation = self.expectation(description: "testConvert to \(format)")
HtmlAPI.convertUrlToLocal(src: src, dst: dst, options: nil) { (data, error) in
guard error == nil else {
XCTFail("Error convert web site to \(format)). Error=\(error!.localizedDescription)")
return
}
let resultPath = data!.file!
XCTAssertTrue(fileExist(name: resultPath))
expectation.fulfill()
}
self.waitForExpectations(timeout: 100.0, handler: nil)
HTML Validation and Parsing via Swift
Aspose.HTML Cloud SDK for Swift has included very useful features for working with HTML documents. The SDK ensures the validity of your HTML documents by using built-in validation features. This helps you maintain high-quality content and avoid rendering issues. Moreover, Software developers can extract specific data from HTML documents effortlessly, enabling them to work with structured content and improve data extraction processes. It supports extracting text and metadata from HTML documents as well as retrieving specific elements or data from HTML content using XPath queries.
HTML to PDF Conversion via Swift API
Aspose.HTML Cloud SDK for Swift allows software developers to convert their HTML documents to PDF format effortlessly inside their Swift applications. The API supports specifying custom settings for PDF generation, such as page size, margins, headers/footers, and orientation. Using the conversion capabilities software developers can generate high-quality reports, invoices, and documentation directly from HTML content. The library also supports conversion to other popular file formats, like DOC, DOCX, MHTML, XPS, EPUB, and many more. The following example demonstrates, how software developers can convert HTML to PDF using Swift commands.
How to Convert HTML to PDF using Swift API?
let fileName = "test.html"
let format = "pdf"
let src = url(forResource: fileName).absoluteString
let expectation = self.expectation(description: "testConvert to \(format)")
let dst = resultDir.appendingPathComponent("Output.\(format)").absoluteString
HtmlAPI.convertLocalToLocal(src: src, dst: dst, options: nil) { (data, error) in
guard error == nil else {
XCTFail("Error convert html to \(format)). Error=\(error!.localizedDescription)")
return
}
let resultPath = data!.file!
XCTAssertTrue(fileExist(name: resultPath))
expectation.fulfill()
}
self.waitForExpectations(timeout: 100.0, handler: nil)
HTML Document Generation via Swift API
Aspose.HTML Cloud SDK for Swift has provided complete functionality for creating a HTML document programmatically inside their Swift applications. There are several important features part of the library for working with HTML documents, such as adding or modifying HTML elements, managing HTML attributes, inserting or modifying content, loading HTML file via URL, Loading HTML from a file, extracting images from HTML documents, handling hyperlinks, working with CSS styles, and many more.