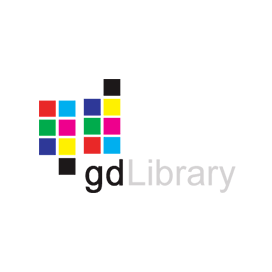
LibGD
Open Source C Library for Advanced Image Processing
Free C API that enables developers to quickly draw images, add lines, arcs, text, multiple colors, and write out the result as a PNG or JPEG file.
Digital images always attract more visitors than simple text. A picture is worth a thousand words. They have the power to attract 94% more views and engage more visitors. It is always more fruitful and convenient to deliver your messages using images. The open source library LibGD enables programmers to quickly draw images, add lines, arcs, text, multiple colors, and write out the result as a PNG or JPEG file.
LibGD is a powerful graphics library that helps s software developers to dynamically generate and manage images inside their C applications. The library can read and write many different image formats such as BMP, GIF, TGA, WBMP, JPEG, PNG, TIFF, WebP, XPM, and much more. The library LibGD is commonly used for website development but also can be used with any standalone application. The library has included several important features for image creation and manipulation such as generating charts, graphics, thumbnails, and most anything else, on the fly.
Getting Started with LibGD
The recommended way to install LibGD is using CMake. Please use the following command for smooth installations
Install LibGD via GitHub.
$ make install
Clone the latest sources using the following command
Install LibGD via GitHub.
$ git https://github.com/libgd/libgd.git
Generate & Modify Images via C
The LibGD library provides the capability to create and manipulate images with ease. It allows using the creation of images in BMP, GIF, TGA, WBMP, JPEG, PNG, TIFF, WebP, XPM file formats with just a couple of lines of C code. One great feature of the library is that it lets you create images on the fly. The library is known for creating images on the fly that can be used on Web pages. It enables you to programmatically create an image, color it, draw on it, and save it to disk with ease.
Generate & Modify Images via C API
gdImagePtr im;
int black, white;
FILE *out;
// Create the image
im = gdImageCreate(100, 100);
// Allocate background
white = gdImageColorAllocate(im, 255, 255, 255);
// Allocate drawing color
black = gdImageColorAllocate(im, 0, 0, 0);
// Draw rectangle
gdImageRectangle(im, 0, 0, 99, 99, black);
// Open output file in binary mode
out = fopen("rect.jpg", "wb");
// Write JPEG using default quality
gdImageJpeg(im, out, -1);
// Close file
fclose(out);
// Destroy image
gdImageDestroy(im);
Image Resizing via C Library
The open source LibGD library enables computer programmers to resize their images on the fly using C commands inside their applications. To start you need to provide the complete path and name of the image for loading. Once it is done you need to provide the width and height of your new image and the output location of your choice where you want to save it. Please make sure all the provided information is correct other than the library not accepting it and throwing an exception.
Resize Image via C API
int main (int argc, char *argv[]) {
FILE *fp;
gdImagePtr in, out;
int w, h;
/* Help */
if (argc<=4) {
printf("%s input.jpg output.jpg width height\n", argv[0]);
return 1;
}
/* Size */
w = atoi(argv[3]);
h = atoi(argv[4]);
if (w<=0 || h<=0) {
fprintf(stderr, "Bad size %dx%d\n", h, w);
return 2;
}
/* Input */
fp = fopen(argv[1], "rb");
if (!fp) {
fprintf(stderr, "Can't read image %s\n", argv[1]);
return 3;
}
in = gdImageCreateFromJpeg(fp);
fclose(fp);
if (!in) {
fprintf(stderr, "Can't create image from %s\n", argv[1]);
return 4;
}
/* Resize */
gdImageSetInterpolationMethod(in, GD_BILINEAR_FIXED);
out = gdImageScale(in, w, h);
if (!out) {
fprintf(stderr, "gdImageScale fails\n");
return 5;
}
/* Output */
fp = fopen(argv[2], "wb");
if (!fp) {
fprintf(stderr, "Can't save image %s\n", argv[2]);
return 6;
}
gdImageJpeg(out, fp, 90);
fclose(fp);
/* Cleanups */
gdImageDestroy(in);
gdImageDestroy(out);
return 0;
}
Crop, Flip, Convert or Rotate Images via C API
The LibGD library has provided complete support for programmatically cropping and Flipping images using C commands. The library has provided multiple functions for flipping the image, such as flipping the image horizontally or vertically as well as both. Same like flipping you can also easily rotate it according to your own needs. Once done you can save the images with ease. It also provides support for converting PNG and JPEG images to other supported file formats.
Convert PNG Image to AVIF via C API
int main(int argc, char **argv)
{
gdImagePtr im;
FILE *in, *out;
if (argc != 3) {
fprintf(stderr, "Usage: png2avif infile.png outfile.avif\n");
exit(1);
}
printf("Reading infile %s\n", argv[1]);
in = fopen(argv[1], "rb");
if (!in) {
fprintf(stderr, "Error: input file %s does not exist.\n", argv[1]);
exit(1);
}
im = gdImageCreateFromPng(in);
fclose(in);
if (!im) {
fprintf(stderr, "Error: input file %s is not in PNG format.\n", argv[1]);
exit(1);
}
out = fopen(argv[2], "wb");
if (!out) {
fprintf(stderr, "Error: can't write to output file %s\n", argv[2]);
gdImageDestroy(im);
exit(1);
}
fprintf(stderr, "Encoding...\n");
gdImageAvifEx(im, out, 100, 0);
printf("Wrote outfile %s.\n", argv[2]);
fclose(out);
gdImageDestroy(im);
return 0;
}
Image Loading from Buffer in Memory
The open source library LibGD allows software developers to load an entire image to buffer in memory inside their own C applications. Once the image is loaded developers can easily perform different operations on the image, such as reading the image from the buffer, modifying the image, saving the image to a particular location, and so on. Once done please remember to free the buffer with normal memory management functions.