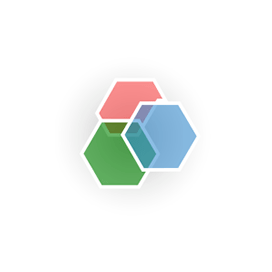
Boost.GIL
Open Source C++ Generic Image Library
C++ API that abstracts image representations from algorithms and supports working with simple and complex images. Generate a Histogram, compute image gradients, convolution & resampling, and so on.
Images are the fundamental part of many projects related to graphics, digital video, computer vision, and image processing. Moreover, images can be represented in many different ways (color space, bit depth, channel order, alignment policy, etc.). So working on a new image-related project that can be generic as well as efficient is very challenging. Boost Generic Image Library (GIL) is an open source library that gives software developers the capability to work with simple and complex images inside their own C++ applications.
The great thing about the Boost.GIL library is that it abstracts image representations from algorithms and allows writing code that can work on a variety of images with performance comparable to hand-writing for a particular image type. So it makes developer's jobs easy by allowing them to write code once and having it work for any image type.
The Boost.GIL library is designed as an STL and Boost compliment. Another great aspect of the library is speed and flexibility. Speed has been a key part of the design of the library. You can easily define any image parameter at run time for a very minor performance cost as compared to many other libraries. It provides support for several important features such as non-byte-aligned pixels, computing image gradients, Boosts integration, assigning a channel to a gray-scale pixel, convolution & resampling, and so on.
Getting Started with Boost.GIL
The easiest way to install Boost.GIL is by using GitHub. Please use the following command for a smooth installation
Install Boost.GILvia GitHub.
git clone --https://github.com/boostorg/gil
Reading and writing images
Histogram is the graphical representation of the tonal distribution in a digital image. In the image processing context, the histogram of an image normally refers to a histogram of the pixel intensity values. The Boost.GIL library enables software developers to generate a histogram inside their own application using C++ code. It can be generated by counting the number of pixel values that fall in each bin. You can also compute the luminosity histogram of the image with ease.
Writing Image via C++ API
#define png_infopp_NULL (png_infopp)NULL
#define int_p_NULL (int*)NULL
#include
#include
using namespace boost::gil;
int main()
{
rgb8_image_t img(512, 512);
rgb8_pixel_t red(255, 0, 0);
fill_pixels(view(img), red);
png_write_view("redsquare.png", const_view(img));
}
Pixel-Level Image Operations using C++ API
The open source library Boost.GIL has provided complete support for pixel-level image operations inside their own C++ applications. The library has included some useful operations that enable users to handle pixel values, pixel pointers, and pixel references, such as making a pixel colored, accessing a channel, comparing the two channels, constructing const planar pointer, converting gray l-value to RGB, and so on.
Convert Color Space to Grayscale via C++
template
void x_luminosity_gradient(SrcView const& src, DstView const& dst)
{
using gray_pixel_t = pixel::type, gray_layout_t>;
x_gradient(color_converted_view(src), dst);
}
Image Transformation inside C++ Apps
The open source Boost.GIL library gives software programmers the ability to rotate images with just a couple of lines of code. The Boost.GIL supports a variety of image transformation functions that can perform any axis-aligned rotation, flip image vertically or horizontally, extract a rectangular sub-image, apply color conversion, , special rotations by 90, 180, or 270 degrees & so on.
Image Transformation via C++ API
template
void y_gradient(const SrcView& src, const DstView& dst) {
x_gradient(rotated90ccw_view(src), rotated90ccw_view(dst));
}