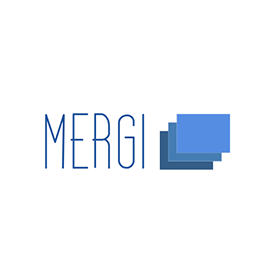
Mergi
GO API for Advanced Image Manipulation
Open Source Go library to programmatically merge, crop, and resize images. You can add watermarks and animation to images inside your own apps.
Mergi is a powerful go library that provides the capability to process images programmatically using GO language. The library is well-organized and can be easily integrated to perform numerous operations with just a couple of lines of Go code. The library is freely available under the MIT license and can be used inside your projects with ease.
The open source Mergi library has included complete support for several important image processing features such as merging images, image cropping support, resizing images, adding watermarks to your images, image animation facility, easing images, adding transition to images, and many more.
Getting Started with Mergi
The easiest way to install Mergi is by using via GitHub. Please use the following command for a smooth installation
Install Mergi via GitHub.
$ go get github.com/noelyahan/mergi
Animate Images via Go API
The open source Mergi library gives software developers the power to animate any given image array result inside their own GO applications. You need to provide the correct path of the image or URL of the file path. Then you need to pass the Images to an array and apply delay according to your need. In the end, you can export the final result via an animation exporter to get the resultant GIF file.
How to Animate Images via Go API
func Animate(imgs []image.Image, delay int) (gif.GIF, error) {
for i, v := range imgs {
if v == nil {
msg := fmt.Sprintf("Mergi found a error image=[%v] on %d", v, i)
return gif.GIF{}, errors.New(msg)
}
}
delays := make([]int, 0)
for i := 0; i < len(imgs); i++ {
delays = append(delays, delay)
}
images := encodeImgPaletted(&imgs)
return gif.GIF{
Image: images,
Delay: delays,
}, nil
}
Image Cropping via Go API
A perfect picture can speak a thousand words. Image cropping is the removal of an unwanted area of an image to create a focus or strengthen the image. The Mergi library enables software programmers to crop their images inside their own Go applications. The developer needs to provide the custom width and height of the required image and location.
Crop Image via Go API
var errCrop = errors.New("Mergi found a error image on Crop")
var errCropBound = errors.New("Mergi expects more than 0 value for bounds")
func Animate(imgs []image.Image, delay int) (gif.GIF, error) {
for i, v := range imgs {
if v == nil {
msg := fmt.Sprintf("Mergi found a error image=[%v] on %d", v, i)
return gif.GIF{}, errors.New(msg)
}
}
delays := make([]int, 0)
for i := 0; i < len(imgs); i++ {
delays = append(delays, delay)
}
images := encodeImgPaletted(&imgs)
return gif.GIF{
Image: images,
Delay: delays,
}, nil
}
Watermarks Addition to Images
The free Mergi library gives software programmers the capability to programmatically insert watermarks to images inside their Go applications with ease. By using the Watermarking feature we can easily protect our images from unauthorized copies creation and distribution. The library supports placing a logo as well as text watermarks using a couple of lines of Go code. The watermarks can be placed at any selected position of your choice as well as with the opacity of your choice.
Add Watermarks to Image via Go API
originalImage, _ := mergi.Import(impexp.NewFileImporter("./testdata/mergi_bg_1.png"))
watermarkImage, _ := mergi.Import(impexp.NewFileImporter("./testdata/glass-mergi_logo_watermark_90x40.jpg"))
res, _ := mergi.Watermark(watermarkImage, originalImage, image.Pt(250, 10))
mergi.Export(impexp.NewFileExporter(res, "watermark.png"))
Image Merging Support
The Mergi library facilitates developers to combine multiple images into a single image according to their needs using Go programming commands. The library supports merging multiple images according to given templates. It supports horizontal merging and vertical merging facilities. To combine two images you need to provide an image path or correct URL and after that, you can select a template to merge the images horizontally or vertically.
Merge Images via Go API
image1, _ := mergi.Import(impexp.NewFileImporter("./testdata/mergi_bg_1.png"))
image2, _ := mergi.Import(impexp.NewFileImporter("./testdata/mergi_bg_2.png"))
horizontalImage, _ := mergi.Merge("TT", []image.Image{image1, image2})
mergi.Export(impexp.NewFileExporter(horizontalImage, "horizontal.png"))
verticalImage, _ := mergi.Merge("TB", []image.Image{image1, image2})
mergi.Export(impexp.NewFileExporter(verticalImage, "vertical.png"))