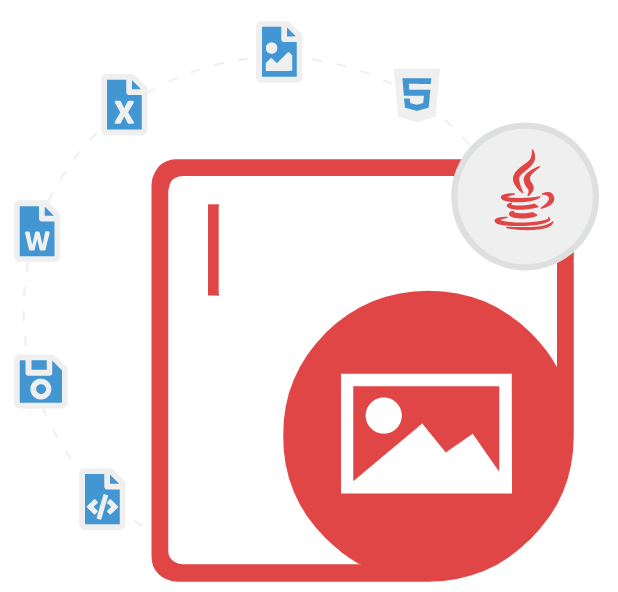
Aspose.Imaging for Java
Java API to Generate, Modify & Convert Images
A useful Java API enables software developers to create, compress, edit, load, manipulate and convert images to JPEG, BMP, TIFF, GIF, PNG, & more.
Aspose.Imaging for Java is a powerful image processing library that allows software developers to perform various image manipulation tasks inside their Java applications. The library allows users to effortlessly manipulate images, convert between various image formats, perform image resizing, cropping, and other modifications with ease. The library has included support for vector images such as SVG and EMF. It provides support for converting vector images to raster images, extracting information from vector images, and more.
Aspose.Imaging for Java empowers software developers to perform complex image processing tasks with ease, saving time and effort. The library supports optimizing images for better quality and smaller file sizes. It provides features to adjust the brightness, contrast, and gamma of images, as well as adjust the color palette of indexed images. The library also supports lossless and lossy compression of images in formats like JPEG and PNG. It provides functions for reading and writing various properties of images such as date and time, camera manufacturer and model, exposure time, and more.
Aspose.Imaging for Java is a comprehensive image processing library that provides a wide range of features for image manipulation, conversions, optimization, and meta-data management, such as drawing Images, image conversion to PDF, Vector Image to Vectorized PSD Image conversion, Set Transparent Image, convert vector image to vectorized PSD image, save a transparent image, transparent TIFF export to transparent PNG, Webp to PNG export, remove background from images, Merge images, convert WMF and EMF to Other Image Formats, Drawing Vector Images and many more.
Getting Started with Aspose.Imaging for Java
The recommend way to install Aspose.Imaging for Java is via Maven repository. You can easily use Aspose.Imaging for Java API directly in your Maven Projects with simple configurations.
Maven repository for Aspose.Imaging for Java
//First you need to specify Aspose Repository configuration / location in your Maven pom.xml as follows:
<repositories>
<repository>
<id>AsposeJavaAPI</id>
<name>Aspose Java API</name>
<url>https://releases.aspose.com/java/repo/</url>
</repository>
</repositories>
//Define Aspose.PDF for Java API Dependency
<dependencies>
<dependency>
<groupId>com.aspose</groupId>
<artifactId>aspose-imaging</artifactId>
<version>22.12</version>
<classifier>22.12</classifier>
</dependency>
</dependencies>
You can download the library directly from Aspose.Imaging product page
Generate & Edit Images inside Java Apps
Aspose.Imaging for Java enables software developers to create new images from the scratch with just a couple of lines of Java code. The library has provided several impotent classes for creating and managing images. It supports numerous options for creating images in various formats such as BMP, GIF, JPEG, PNG, TIFF, PSD, DICOM, TGA, ICO, EMZ, WMZ, and many more. Moreover, you can create images by setting a path, creating an image via stream, resizing images, drawing objects on the image, updating the content of an image and saving images to disk, adjusting image brightness, applying contrast or gamma to image, apply blur effects to an image, check image transparency and so on.
Creating an Image by Setting a Path via Java API?
// The path to the documents directory.
String dataDir = "D:/dataDir/";
// Creates an instance of BmpOptions and set its various properties
BmpOptions imageOptions = new BmpOptions();
imageOptions.setBitsPerPixel(24);
// Define the source property for the instance of BmpOptions Second boolean parameter determines if the file is temporal or not
imageOptions.setSource(new FileCreateSource(dataDir + "CreatingAnImageBySettingPath_out.bmp", false));
try
{
// Creates an instance of Image and call Create method by passing the BmpOptions object
try (Image image = Image.create(imageOptions, 500, 500))
{
image.save(dataDir + "CreatingAnImageBySettingPath1_out.bmp");
}
}
finally
{
imageOptions.close();
}
Image Conversion to Other Formats via Java API
Aspose.Imaging for Java gives software developers the power to convert different types of images to other supported file formats using Java commands. The library has provided several functions for converting images from one format to another, including JPEG, BMP, TIFF, GIF, PNG, DICOM, TGA, ICO, EMZ, WMZ, WebP, SVG, and more. The library also supports converting images to multi-page TIFFs, saving individual pages of a TIFF as separate images, and converting images to PDFs.
Convert TIFF to JPEG image via Java API
// The path to the documents directory.
String dataDir = Utils.getSharedDataDir(ConvertTIFFToJPEG.class) + "ManipulatingJPEGImages/";
TiffImage tiffImage = (TiffImage)Image.load(dataDir + "source2.tif");
try
{
int i = 0;
for (TiffFrame tiffFrame : tiffImage.getFrames())
{
JpegOptions saveOptions = new JpegOptions();
saveOptions.setResolutionSettings(new ResolutionSetting(tiffFrame.getHorizontalResolution(), tiffFrame.getVerticalResolution()));
TiffOptions frameOptions = tiffFrame.getFrameOptions();
if (frameOptions != null)
{
// Set the resolution unit explicitly.
switch (frameOptions.getResolutionUnit())
{
case TiffResolutionUnits.None:
saveOptions.setResolutionUnit(ResolutionUnit.None);
break;
case TiffResolutionUnits.Inch:
saveOptions.setResolutionUnit(ResolutionUnit.Inch);
break;
case TiffResolutionUnits.Centimeter:
saveOptions.setResolutionUnit(ResolutionUnit.Cm);
break;
default:
throw new RuntimeException("Current resolution unit is unsupported!");
}
}
String fileName = "source2.tif.frame." + (i++) + "."
+ ResolutionUnit.toString(ResolutionUnit.class, saveOptions.getResolutionUnit()) + ".jpg";
tiffFrame.save(dataDir + fileName, saveOptions);
}
}
finally
{
tiffImage.close();
}
Manipulate Images via Java API
Aspose.Imaging for Java makes it easy for computer programmer to access and manipulate existing images with ease. There are several functions part of the library for handling image manipulation, such as update image properties, draw vector graphics, multipage image handling, delete or update image background, images merging (JPG to JPG, JPG to PDF merge, JPG to PNG), image cropping, rotate images , images resizing, deskew images, adding watermark to image, draw raster images on vector images and so on.
Apply Median Filter on Images via Java API
// Load the noisy image
Image image = Image.load(dataDir + "aspose-logo.gif");
// caste the image into RasterImage
RasterImage rasterImage = (RasterImage) image;
if (rasterImage == null)
{
return;
}
// Create an instance of MedianFilterOptions class and set the size.
MedianFilterOptions options = new MedianFilterOptions(4);
// Apply MedianFilterOptions filter to RasterImage object.
rasterImage.filter(image.getBounds(), options);
// Save the resultant image
image.save(dataDir + "median_test_denoise_out.gif");
Rotate and Resize Images via Java API
Aspose.Imaging for Java allows software developers to programmatically rotate and resize images inside their own Java applications. Cropping is a very useful technique that can be used to cut out some portion of an image to increase the focus on a particular area. The library has provided several features related to images rotation and resizing, such as cropping images by shifts, images cropping by rectangle, vector image cropping, rotating images by 90/180/270 degrees, flip images horizontally or vertically, rotating images on a specified angle, resize webp image, resize an image proportionally and many more.
How to Crop an Image by Shifts via Java API?
u// The path to the documents directory.
String dataDir = "dataDir/jpeg/";
// Load an existing image into an instance of RasterImage class
try (RasterImage rasterImage = (RasterImage)Image.load(dataDir + "aspose-logo.jpg"))
{
// Before cropping, the image should be cached for better performance
if (!rasterImage.isCached())
{
rasterImage.cacheData();
}
// Define shift values for all four sides
int leftShift = 10;
int rightShift = 10;
int topShift = 10;
int bottomShift = 10;
// Based on the shift values, apply the cropping on image Crop method will shift the image bounds toward the center of image and Save the results to disk
rasterImage.crop(leftShift, rightShift, topShift, bottomShift);
rasterImage.save(dataDir + "CroppingByShifts_out.jpg");