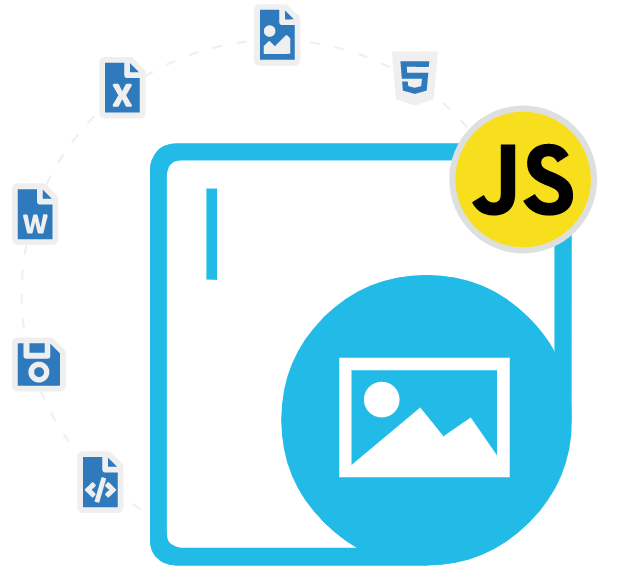
Aspose.Imaging Cloud SDK for JavaScript
JavaScript API to Create, Manage & Convert Images
A Powerful JavaScript Library allows Programmers to Create, Edit, Resize, Crop, Rotate & Convert PSD, PNG, JPG, BMP, TIFF, and GIF image file formats.
In today's digital age, image processing, and manipulation play a vital role in various industries, including web development, graphic design, and multimedia production. Aspose.Imaging SDK for JavaScript is a dominant software development kit (SDK) that provides comprehensive image processing capabilities enabling software developers to effortlessly incorporate advanced image creation, conversion, and manipulation functionalities into their web applications. The SDK offers seamless integration with popular JavaScript frameworks, making it suitable for both new as well as existing projects.
Aspose.Imaging Cloud SDK for JavaScript equips Software developers with a comprehensive set of features for advanced image processing. Whether it's resizing, cropping, rotating, flipping, watermarking or applying filters, the SDK offers an extensive range of methods and options to accomplish these tasks with ease. Software programmers can utilize these features to manipulate images dynamically on the client-side, providing an enhanced user experience and reducing server-side processing overhead. Whether developers need to resize an image for responsive web design or add watermarks to protect your intellectual property, Aspose.Imaging simplifies these tasks with its intuitive API.
The Aspose.Imaging Cloud SDK for JavaScript supports a wide array of image formats, ensuring compatibility and flexibility in handling diverse image files. From popular formats like JPEG, PNG, PSD, WEBP, and GIF to industry-standard formats such as TIFF, SVG, and BMP, developers can rely on the SDK to handle various image types without any hassle. There are some advanced image editing and transformation also part of the library such as applying artistic effects and filters as well as adding watermarks, annotations, and text overlays. With its broad range of features, cross-browser compatibility, and optimized performance, the SDK is a great tool for software developers to build robust image manipulation solutions that enhance user experience and drive innovation in various industries.
Getting Started with Aspose.Imaging Cloud SDK for JavaScript
The recommend way to install Aspose.Imaging Cloud SDK for JavaScript is using npm. Please use the following command for a smooth installation.
Install Aspose.Imaging Cloud SDK for JavaScript via NuGet
npm i @asposecloud/aspose-imaging-cloud
or
npm install aspose-imaging-cloud –save
You can download the library directly from Aspose.Imaging product page
Image Conversion to Other Formats via JS API
Aspose.Imaging Cloud SDK for JavaScript allows software developers to load and convert images to various other supported file formats with just a couple of lines of JavaScript code. The SDK supports conversion to various file formats such as BMP, GIF, DJVU, WMF, EMF, JPEG, JPEG2000, PSD, TIFF, WEBP, PNG, DICOM, CDR, CMX, ODG, DNG, SVG, PDF, and many more. The following example shows how to convert an image to a PNG file format via JavaScript.
How to Convert Image to a PNG Format via JavaScript API?
//Load Image
const Image = require('aspose.imaging');
const image = Image.load('path/to/input/image.jpg');
//Save image in PNG format
image.save('path/to/output/image.png', new ImageOptions.png());
//saves the image in JPEG format with a compression level of 75:
const options = new ImageOptions.jpeg();
options.setQuality(75);
image.save('path/to/output/image.jpg', options);
Get & Update Image Properties via JavaScript Apps
Aspose.Imaging Cloud SDK for JavaScript has provided complete support for handling image properties inside JavaScript applications. The API allows developers to update image properties such as background color, page width, page height, border width and border height. The library has included several other important features for working with various image properties, such as getting existing image properties, update EMG & WMF image properties, manage GIF properties, update EMF image properties and so on.
Get, Modify & Save Existing Image Properties via Java API
// load an image
const image = AsposeImaging.Image.load('path/to/image.jpg');
// access various properties of the loaded image
console.log('Image width:', image.getWidth());
console.log('Image height:', image.getHeight());
console.log('Image format:', image.getFileFormat());
console.log('Image resolution:', image.getResolutionSettings());
console.log('Image color mode:', image.getColorMode());
// modify certain image properties
const newWidth = 800;
const newHeight = 600;
image.resize(newWidth, newHeight);
// Save the modified image
const outputPath = 'path/to/output.jpg';
const options = new AsposeImaging.ImageOptions.JpegOptions();
options.setQuality(80); // Set JPEG quality to 80%
image.save(outputPath, options);
Apply Filters to Images via JavaScript API
Aspose.Imaging Cloud SDK for JavaScript make it easy for software developers to load an image from a remote location via URL and apply filters to the loaded image using JavaScript commands. There are various filter options available in the SDK, such as BigRectangular, SmallRectangular, Median, GaussWiener, MotionWiener, GaussianBlur, Sharpen, BilateralSmoothing and so on. The following example shows how software developers can load an image and apply different types to filters to it inside JavaScript applications.
How to Apply Filters to Images via JavaScript API?
//Load image
AsposeImaging.loadImage("path/to/image.jpg", function (image) {
// Image loaded successfully
image.filter(image.filterType.Grayscale); // Apply grayscale filter
image.filter(image.filterType.Brightness, 0.5); // Apply brightness filter
image.filter(image.filterType.Contrast, 1.2); // Apply contrast filter
// Save the modified image
image.save("path/to/modified_image.jpg", function () {
// Image saved successfully
console.log("Modified image saved");
}, function (error) {
// Error occurred while saving image
console.log(error);
});
}, function (error) {
// Error occurred while loading image
console.log(error);
});
Resize Images via JavaScript API
Aspose.Imaging Cloud SDK for JavaScript enables software developers to resize various types of images with just a couple of lines of JavaScript code. The API provides support for scaling, cropping, flipping and exporting of an image in a single API call. The SDK also provide support for saving image to other format after resizing the image. The following example shows how software developers can load and resize a JPG image inside JavaScript applications.
How to Resize a JPG Images via JavaScript API?
const inputFileName = "input.jpg";
const outputFileName = "output.jpg";
const newWidth = 800;
const newHeight = 600;
imagingApi.createResizedImage(
{ name: inputFileName, format: "jpg" },
newWidth,
newHeight,
null,
null,
null,
null,
null,
ResizeType.LanczosResample,
null,
{ folder: "inputFolder" }
).then((result) => {
console.log("Image resized successfully");
console.log(result);
}).catch((error) => {
console.log("Error occurred:", error);
});