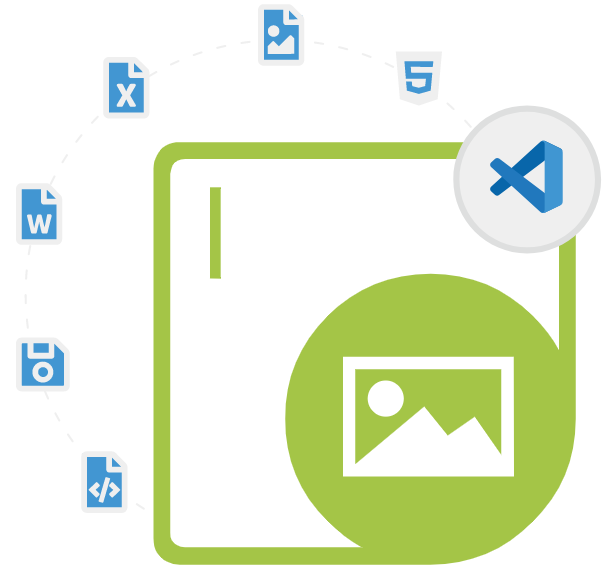
Aspose.Imaging for .NET
C# .NET API to Create, Edit & Convert Images
A useful C# ASP.NET advanced image processing API that allows software developers to create, edit, load, manipulate, convert, and compress images.
Aspose.Imaging for .NET is a powerful and flexible library that provides advanced image processing capabilities without installing any image editor on the machine. The library supports numerous image file formats, including JPEG, BMP, TIFF, GIF, PNG, DICOM, TGA, ICO, EMZ, WMZ, WebP, Svg, Svgz, and many more. The library is very flexible and can be used with ASP.NET web applications as well as Windows desktop applications.
Aspose.Imaging for .NET library has included various image drawing features such as Rotate, Flip, scale, crop, drawing and filling of basic shapes (Line, Polygon, Rectangle, Cubic Bézier, Curve, Arc, Ellipse, Pie and Path), clipping to rectangular region, matrix transformations, vector to raster export, custom user images and so on. It also provides a comprehensive set of APIs for working with EXIF and IPTC metadata, enabling developers to read and write image metadata, including image description, author, and more.
Aspose.Imaging for .NET is optimized for high-speed image processing and supports parallel processing allowing software developers to perform complex image manipulation tasks quickly and efficiently. The library is highly customizable, allowing developers to easily extend the library to meet their specific needs. For example, they can create custom image filters and apply them to images in a few simple steps. They can also create custom image format handlers, enabling them to work with unique image formats that are not supported by the library out of the box.
Getting Started with Aspose.Imaging for .NET
The recommend way to install Aspose.Imaging for .NET is using NuGet. Please use the following command for a smooth installation.
Install Aspose.Imaging for .NET via NuGet
NuGet\Install-Package Aspose.Imaging -Version 22.12.0
You can also download it directly from Aspose product page.Create and Manage Images via .NET API
Aspose.Imaging for .NET has included complete functionality for creating and managing new images inside .NET applications. The library allows software developers to create new images from the scratch, generate images by setting path, creating images by using stream and so on. The library also allows users to open existing images from the disk or from the stream, make desired changes to it and save it to the location of their choice. The library supports image color adjustments, set brightness and contrast, adjust gamma, apply Blur effect, applly image transparency, resizing images, extract text as shapes and much more.
How to Create Image using Stream via .NET API?
using Aspose.Imaging;
using Aspose.Imaging.ImageOptions;
using Aspose.Imaging.Sources;
using System.IO;
string templatesFolder = @"c:\Users\USER\Downloads\templates\";
string dataDir = templatesFolder;
// Creates an instance of BmpOptions and set its various properties
BmpOptions ImageOptions = new BmpOptions();
ImageOptions.BitsPerPixel = 24;
// Create an instance of System.IO.Stream
Stream stream = new FileStream(dataDir + "result1.bmp", FileMode.Create);
// Define the source property for the instance of BmpOptions Second boolean parameter determines if the Stream is disposed once get out of scope
ImageOptions.Source = new StreamSource(stream, true);
// Creates an instance of Image and call Create method by passing the BmpOptions object
using (Image image = Image.Create(ImageOptions, 500, 500))
{
// Do some image processing
image.Save(dataDir + "result2.bmp");
}
File.Delete(dataDir + "result1.bmp");
File.Delete(dataDir + "result2.bmp");
Image Conversion via C# .NET API
Aspose.Imaging for .NET enables software developers to convert different types of images inside their own applications. It is often required to convert colored images to Black & White or Grayscale for printing or archiving purposes. The library supports a wide range of image formats JPEG, BMP, TIFF, GIF, PNG, DICOM, TGA, ICO, EMZ, WMZ, WebP, SVG, SVGZ and many more. Some popular examples of images conversion are convert layers of a GIF Image to TIFF, Raster Image conversion to PDF, converting RGB color system to CMYK, convert SVG to Raster image, convert SVG to PNG, and convert SVG to Bmp and so on.
Convert Image to Grayscalevia via C# API
using Aspose.Imaging;
using System.IO;
string templatesFolder = @"c:\Users\USER\Downloads\templates\";
string dataDir = templatesFolder;
// Load an image in an instance of Image
using (Image image = Image.Load(dataDir + "template.jpg"))
{
// Cast the image to RasterCachedImage and Check if image is cached
RasterCachedImage rasterCachedImage = (RasterCachedImage)image;
if (!rasterCachedImage.IsCached)
{
// Cache image if not already cached
rasterCachedImage.CacheData();
}
// Binarize image with predefined fixed threshold and Save the resultant image
rasterCachedImage.Grayscale();
rasterCachedImage.Save(dataDir + "result.jpg");
}
File.Delete(dataDir + "result.jpg");
Image Manipulation using .NET API
Aspose.Imaging for .NET enables software developers to create new images and manipulate different types of images inside their own C# .NET applications. The library has provide several important features related to image manipulations, such as image background removal, applying median and wiener filters, convert images to different file formats, merge images, crop, rotate, and resize images, setting images properties, handle multipage image formats, and so on. Additionally the library also supports manipulating different image file formats, such as including JPEG, CMX, CorelDraw, GNG, TMA, SVG, BMP, TIFF, GIF, PNG, DICOM, TGA, ICO, EMZ, WMZ and so on.
Apply Filter on PNG Images via C# API
using Aspose.Imaging;
using Aspose.Imaging.FileFormats.Png;
using Aspose.Imaging.ImageOptions;
using System.IO;
string templatesFolder = @"c:\Users\USER\Downloads\templates\";
string dataDir = templatesFolder;
using (PngImage png = (PngImage)Image.Load(dataDir + "template.png"))
{
// Create an instance of PngOptions, Set the PNG filter method and Save changes to the disc
PngOptions options = new PngOptions();
options.FilterType = PngFilterType.Paeth;
png.Save(dataDir + "result.png", options);
}
File.Delete(dataDir + "result.png");
Draw Images & Shapes via .NET API
Aspose.Imaging for .NET has include complete support for drawing various types of shapes and images with ease. It allows software developers to draw different types of shapes and images such as lines, ellipse, rectangle, arc, Bezier and so on. The library also supports drawing images using core functionality such as, manipulating an image’s bitmap information, or uses the advanced features like Graphics and GraphicsPath to draw shapes on image surface with the help of different brushes and pens. The library can easily retrieve an image area’s pixel information manipulate it according to user’s need.
How to Add Rectangle Shape on Image Surface via .NET API?
using Aspose.Imaging;
using Aspose.Imaging.Brushes;
using Aspose.Imaging.ImageOptions;
using Aspose.Imaging.Sources;
using System.IO;
string templatesFolder = @"c:\Users\USER\Downloads\templates\";
string dataDir = templatesFolder;
// Creates an instance of FileStream
using (FileStream stream = new FileStream(dataDir + "result.bmp", FileMode.Create))
{
// Create an instance of BmpOptions and set its various properties
BmpOptions saveOptions = new BmpOptions();
saveOptions.BitsPerPixel = 32;
// Set the Source for BmpOptions and Create an instance of Image
saveOptions.Source = new StreamSource(stream);
using (Image image = Image.Create(saveOptions, 100, 100))
{
// Create and initialize an instance of Graphics class, Clear Graphics surface, Draw a rectangle shapes and save all changes.
Graphics graphic = new Graphics(image);
graphic.Clear(Color.Yellow);
graphic.DrawRectangle(new Pen(Color.Red), new Rectangle(30, 10, 40, 80));
graphic.DrawRectangle(new Pen(new SolidBrush(Color.Blue)), new Rectangle(10, 30, 80, 40));
image.Save();
}
}
File.Delete(dataDir + "result.bmp");