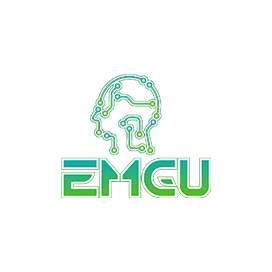
Emgu CV
Open Source .NET Image Processing Library
C# API enables software developers to capture images from either camera or video file, geometric transformation support & much more.
Emgu CV is an open source cross-platform .NET wrapper to the OpenCV image processing library that gives software developers the capability to perform simple and advanced image creation and manipulation inside their own .NET apps. It can be smoothly run on Windows, Linux, Mac OS, iOS, and Android with ease.
Emgu CV is a pure C# implementation that can be used in several different languages, including C#, VB.NET, C++, and IronPython. The library has included several important features related to image processing such as generating a new image, modifying images, capturing images from either camera or video file, writing images to video format, Background Segmentation, geometric transformation support, Optical character recognition support, Image stitching, and many more.
Getting Started with Emgu CV
To run your project using Emgu CV, first of all, you need to install .NET runtime. After that, you can manually download the repository from GitHub. Use the following command to install it.
Install Emgu CV via GitHub
git clone https://github.com/emgucv/emgucv.git
Creating and Managing Image via .NET
The free Emgu CV library enables software programmers to create apps that can create and process images with ease. You can create an image with ease but it is suggested to construct an image object. Using the managed class you can have several advantages such as automatic memory management, automatic garbage collection and contains advanced methods such as generic operation on image pixels, conversion to Bitmap, and so on. You can easily adjust the size, apply color, specify image depth, and much more
Create image via .NET
//Create an image of 400x200 of Blue color
using (Image img = new Image(400, 200, new Bgr(255, 0, 0)))
{
//Create the font
MCvFont f = new MCvFont(CvEnum.FONT.CV_FONT_HERSHEY_COMPLEX, 1.0, 1.0);
//Draw "Hello, world." on the image using the specific font
img.Draw("Hello, world", ref f, new Point(10, 80), new Bgr(0, 255, 0));
//Show the image using ImageViewer from Emgu.CV.UI
ImageViewer.Show(img, "Test Window");
}
Traffic Sign Detection via .NET API
The open source Emgu CV API has included support for detecting traffic sing with ease using .NET commands. The library helps developers to detect stop signs from images captured by cameras which is an important part of the autonomous vehicle navigation system. It is very useful and can help the automobile to navigate itself safely in an urban environment. In the first step, developers need to extract red octagons of the traffic signals and they can use SURF to match features on the candidate region to match it.
Perform Image Stitching via CSharp
The Emgu CV library helps developers to perform image stitching inside their own apps using C# code. It is a very useful process for combining multiple photographic images to generate a segmented panorama or high-resolution image. The library just requires a couple of lines of code to perform it but it is important to remember that for accurate results nearly exact overlaps between images and identical exposures are required.
Perform Image Stitching via .NET
using (Stitcher stitcher = new Stitcher(
//This indicate if the Stitcher should use GPU for processing.
//There is currently a bug in Open CV such that GPU processing cannot produce the correct result.
//Must specify false as parameter. Hope this will be fixed soon to enable GPU processing
false
))
{
Image result = stitcher.Stitch(sourceImages);
// code to display or save the result
}