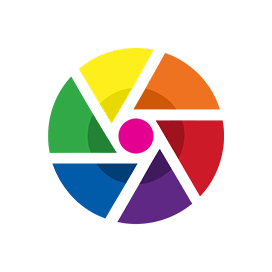
ImageSharp
Open Source C# .NET Library for 2D Graphics
Read, Write, Modify, Resize & Convert PNG, JPEG, GIF & TIFF Images using .NET API.
ImageSharp is a simple yet very powerful cross-platform open source library for the processing of image files inside C# applications. It is a fully managed and cross-platform 2D graphics API designed to permit the processing of images. ImageSharp is an open source image processing library that was released with the goal of providing an alternative to the System.Drawing APIs.
This API is comprehensive and supports advanced algorithms for image processing. The API is improved year by year to provide support for more advanced image processing. Its only dependency is .NET itself, which makes it extremely portable. The API has included support for advanced features like image resizing, image encoding and decoding, decoding image metadata only, image cloning, Drawing watermark on the image, Drawing text along a path, and many more.
Getting Started with ImageSharp
A Stable release is available on NuGet For beta versions, make sure that the Include Prerelease switch is enabled. Development releases are available via MyGet.
Install ImageSharp via NuGet
Install-Package SixLabors.ImageSharp -Version number
C# API to Resize Images
The ImageSharp library allows C# .NET programmers to resize images inside their own .NET applications. Resizing an image requires the process of generating and iterating through the pixels of a target image and sampling areas of a source image to choose what color to implement for each pixel. You can easily set the algorithm when processing images, such as Bicubic, Hermite, Box, CatmullRom, Lanczos2, and more. Apart from the basic resizing operations, ImageSharp also offers more advanced features.
Resize Images via C# API
// Load File
using (Image image = Image.Load("fileformat.jpg"))
{
// Resize file
image.Mutate(x => x
.Resize(image.Width / 2, image.Height / 2)
.greyscale());
// Save
image.Save("fileformat_out.jpg");
}
Draw Watermark on Image
Usually, people protect their images by putting a large watermark overlaid to prevent people from using the images without authorization. The ImageSharp library provides support for adding watermarks to images inside C++ applications. To start you need a font family and you can easily get one from the system font store. Drawn the text over the image & grey it with 50% opacity.
Draw Text Along a Path & Apply Effect to Image
The ImageSharp library allows C# .NET developers to draw some text following the contours of a path. It is recommended to create a font collection. First, let’s generate the text as a set of vectors drawn along the path. After drawing the path so we can see what the text is supposed to be following. The ImageSharp API also allows to apply various types of effects to images only inside a shape.
Apply Effect to Images
namespace CustomImageProcessor
{
static class Program
{
static void Main(string[] args)
{
System.IO.Directory.CreateDirectory("output");
using (Image image = Image.Load("fb.jpg"))
{
var outerRadii = Math.Min(image.Width, image.Height) / 2;
var star = new Star(new PointF(image.Width / 2, image.Height / 2), 5, outerRadii / 2, outerRadii);
using (var clone = image.Clone(p =>
{
p.GaussianBlur(15); // apply the effect here you and inside the shape
}))
{
clone.Mutate(x => x.Crop((Rectangle)star.Bounds));
var brush = new ImageBrush(clone);
// cloned image with the effects applied
image.Mutate(c => c.Fill(brush, star));
}
image.Save("output/fb.png");
}
}
}
}