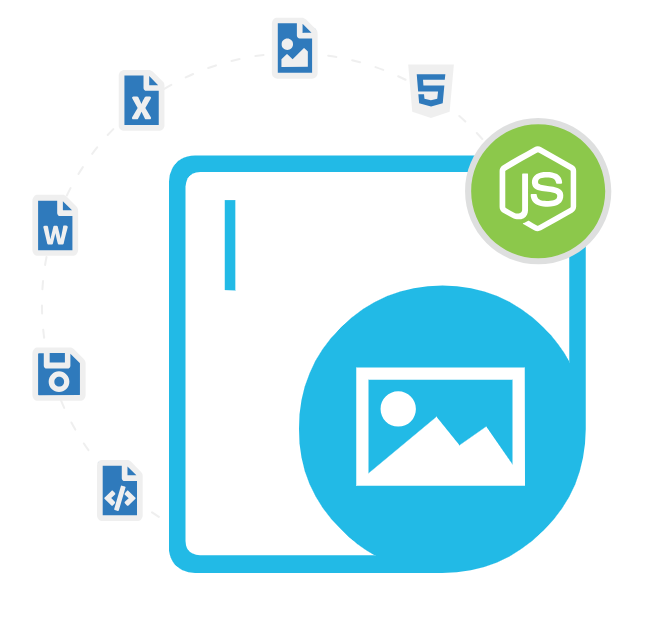
Aspose.Imaging Cloud SDK for Node.js
Node.js API to Create, Manage & Convert Images
A Powerful Node.js allows Programmers to Create, Edit, Resize, Crop, Rotate & Convert PSD, PNG, JPG, BMP, TIFF, and GIF image file formats.
In today's digital age, image processing has become an essential aspect of various industries, including healthcare, finance, and e-commerce. For developers using Node.js, the challenge often lies in finding a robust and scalable solution that integrates seamlessly with their existing workflows. With the increasing demand for efficient and scalable image processing solutions, Aspose has introduced the Aspose.Imaging Cloud SDK for Node.js, a powerful open source API that enables software developers to create, edit, convert and manipulate images in the cloud.
Aspose.Imaging Cloud SDK for Node.js stands out as a comprehensive solution for developers who need to perform a variety of image processing tasks within their applications. Unlike traditional libraries that require local resources and complex setup, this SDK leverages cloud infrastructure to provide a scalable and high-performance environment for image manipulation. This SDK provides a wide range of features, such as new images creation in different formats, image compression, resizing images to fit specific dimensions, cropping images to remove unwanted areas, rotating images to adjust their orientation and conversion to other file formats, making it an ideal solution for software developers who need to integrate image processing capabilities into their applications.
Aspose.Imaging Cloud SDK for Node.js is very easy to handle and supports all major image formats, including JPEG, PNG, GIF, BMP, TIFF, PSD, SVG, and many more. With cloud-based image processing, your application can scale effortlessly to handle large volumes of image processing tasks without the need for additional infrastructure. The SDK is built to deliver high-quality output, ensuring that images are processed without losing fidelity, which is particularly important for professional-grade applications. With its broad range of features, cross-browser compatibility, and high-quality output, the SDK is a great tool for software developers to build robust image processing solutions that enhance user experience and drive innovation in various industries.
Getting Started with Aspose.Imaging Cloud SDK for Node.js
The recommend way to install Aspose.Imaging Cloud SDK for Node.js t is using npm. Please use the following command for a smooth installation.
Install Aspose.Imaging Cloud SDK for Node.js via NPM
npm i @asposecloud/aspose-imaging-cloud
or
npm install aspose-imaging-cloud –save
You can download the library directly from Aspose.Imaging product page
Effortless Image Conversion in Node.js Apps
Converting images from one format to another is a common requirement in many software applications. Aspose.Imaging Cloud SDK for Node.js simplifies this process with its straightforward API, allowing software developers to convert images between formats with just a few lines of code. The conversion process maintains the integrity and quality of the original image, ensuring that no details are lost. Here's a simple example that shows, how software developers can convert an image from JPEG to PNG inside Node.js Apps.
How to Convert an Image from JPEG to PNG inside Node.js Apps?
// Get your ClientId and ClientSecret from https://dashboard.aspose.cloud (free registration required).
const imagingApi = new ImagingApi("MY_CLIENT_SECRET", "MY_CLIENT_ID");
const request = new ConvertImageRequest({ "sample.jpg", "png", "tempFolder", "My_Storage_Name" });
imagingApi.convertImage(request).then((response) => {
fs.writeFile("sample.png", response.body, (err) => {
if (err) throw err;
});
});
Advanced Image Comparison & Manipulation in Node.js
Aspose.Imaging Cloud SDK for Node.js provides a robust set of functions for comparison and manipulating various types of image formats inside Node.js applications. Software Developers can easily compare different images and perform operations such as resizing, cropping, rotating, and flipping images. Additionally, the SDK supports applying filters and effects like grayscale conversion, dithering, and edge detection, enabling developers to enhance or transform images directly within their applications. The following examples shows, how software developers can compare two images inside their Node.js applications.
How to Compare Two Images inside Node.js Applications?
const imagingApi = new imaging.ImagingApi("yourClientSecret", "yourClientId");
// create search context or use existing search context ID if search context was created earlier
const apiResponse = await imagingApi.createImageSearch(
new imaging.CreateImageSearchRequest());
const searchContextId = apiResponse.id;
// specify images for comparing (image ID is a path to image in storage)
const imageInStorage1 = "WorkFolder\Image1.jpg";
const imageInStorage2 = "WorkFolder\Image2.jpg";
// compare images
const response = await imagingApi.compareImages(
new imaging.CompareImagesRequest({
searchContextId, imageId1: imageInStorage1, imageId2: imageInStorage2 }));
const similarity = response.results[0].similarity;
High-Quality Image Rendering in Node.js
Maintaining the quality of images during processing is critical, especially for professional and high-resolution images. Aspose.Imaging Cloud SDK for Node.js ensures high-fidelity rendering of images, preserving the original quality and details, which is essential for applications that require precise image processing. When rendering images, you can specify parameters such as resolution, compression, and quality settings to ensure the output meets your requirements. In the following example shows, how developers can render a JPEG image with specific quality settings to ensure high fidelity.
How to Render a JPEG Image with Specific Quality Settings via Node.js API?
const inputImage = "high-res-image.jpg";
const outputImage = "rendered-image.jpg";
const folder = "images";
const renderImage = async () => {
const quality = 100; // Set quality to maximum (100)
const compressionType = "Baseline"; // Use baseline compression
const request = new CreateModifiedJpegRequest({
name: inputImage,
quality: quality,
compressionType: compressionType,
folder: folder,
storage: null, // Optional storage parameter
});
try {
const result = await imagingApi.createModifiedJpeg(request);
console.log("Image rendered successfully:", result);
} catch (error) {
console.error("Error during image rendering:", error);
}
};
renderImage();
Work with Multiple Image Formats in Node.js
One of the most notable features of Aspose.Imaging Cloud SDK for Node.js API is its extensive support for a wide array of image formats. Software developers can create, edit, resize, and convert popular image file formats like JPEG, PNG, GIF, BMP, TIFF, or even more specialized formats like PSD and SVG, with just a couple of lines of code. This versatility allows developers to handle different image formats without needing to rely on 3d-party libraries and tools.