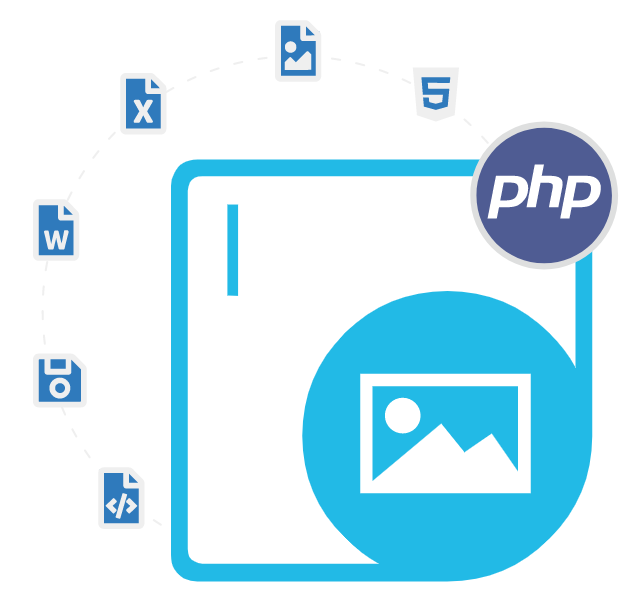
Aspose.Imaging Cloud SDK for PHP
PHP API to Create, Re-size, Rotate, & Convert Images
A Powerful REST API for image processing, enables software developers to create, edit, compress, manipulate, convert, and compress images.
As technology advances, the need for efficient image processing and manipulation grows even more important. However, performing these tasks on a large scale can be both time-consuming and resource-intensive. This is where Aspose.Imaging Cloud SDK for PHP shows his power and importance. This powerful cloud-based solution provides software developers with a wide range of image processing tools that can be easily integrated into their applications.
Aspose.Imaging Cloud SDK for PHP is a powerful and stable cloud-based image processing solution that allows software developers to perform a wide range of image manipulation tasks inside their PHP applications. It provides developers with a simplified interface for accessing these tools, making it easy to integrate image processing capabilities into their applications. The SDK supports a wide range of image formats, including BMP, GIF, DJVU, WMF, EMF, JPEG, JPEG2000, PSD, TIFF, WEBP, PNG, DICOM, CDR, ODG, DNG, SVG, and CMX.
Aspose.Imaging Cloud SDK for PHP offers a number of key features that make it an attractive solution for software developers looking to enhance their image processing capabilities in the cloud, such as variety of options for resizing images, setting image size in pixels or percentage, applying filters to images (blur, sharpen, color balance), cropping images, flipping images, image rotation, TIFF frames extraction, retrieve & update image properties, and many more. Software developers can also convert numerous images from one format to another in the cloud such as BMP, PSD, JPEG, TIFF, GIF, PNG, JPEG2000, WEBP, and PDF. Overall, Aspose.Imaging Cloud SDK for PHP is a great choice for handling image processing capabilities in the cloud.
Getting Started with Aspose.Imaging Cloud SDK for PHP
The recommend way to install Aspose.Imaging Cloud SDK for PHP is using Composer. Please use the following command for a smooth installation.
Install Aspose.Imaging Cloud SDK for PHP via Composer
composer require aspose/imaging-cloud-sdk-php
You can also download it directly from Aspose product page.Create & Edit Images via PHP API
Aspose.Imaging Cloud SDK for PHP gives software developers the power to create and manage images inside their own applications. The library has included support for reading and writing some popular image file formats such as BMP, GIF, JPEG, JPEG2000, PSD, TIFF, WEBP, PNG, WMF, EMF, SVG and many more. It also provides read only support images like DJVU, DICOM, CDR, CMX, ODG, DNG, EPS and more. The library also supports loading and converting EPS files to PDF/A format with just a couple of lines of PHP code. You can easily get image properties and update it according to your need inside PHP applications.
How to Create Images via PHP API?/h3>$imagingApi = new \Aspose\Imaging\ImagingApi($config);
// Create a new image
$newImage = $imagingApi->createImage(
new \Aspose\Imaging\Model\CreateImageRequest(
new \Aspose\Imaging\Model\JpegOptions(),
800, 600
)
);
// Upload an image
$file = fopen("image.jpg", "r");
$imagingApi->uploadFile(
new \Aspose\Imaging\Model\UploadFileRequest(
"image.jpg",
$file
)
);
$imagingApi = new \Aspose\Imaging\ImagingApi($config);
// Create a new image
$newImage = $imagingApi->createImage(
new \Aspose\Imaging\Model\CreateImageRequest(
new \Aspose\Imaging\Model\JpegOptions(),
800, 600
)
);
// Upload an image
$file = fopen("image.jpg", "r");
$imagingApi->uploadFile(
new \Aspose\Imaging\Model\UploadFileRequest(
"image.jpg",
$file
)
);
Convert Image to Other Formats via PHP API
Aspose.Imaging Cloud SDK for PHP enables software developers to convert an existing image to another file format using PHP commands. Software developers can easily upload an image to the cloud storage and convert it to desired image format in the cloud. The library has included support for loading and converting some popular image file formats such as BMP, GIF, DJVU, WMF, EMF, JPEG, JPEG2000, PSD, TIFF, WEBP, PNG, DICOM, CDR, CMX, ODG, DNG and SVG. The following example demonstrates how to load a JPG images and convert it to PNG file format using PHP code.
How to Convert JPG to PNG via PHP
// Get your ClientId and ClientSecret from https://dashboard.aspose.cloud (free registration required).
$config = new Configuration();
$config->setAppSid("MY_CLIENT_ID");
$config->setAppKey("MY_CLIENT_SECRET");
$api = new ImagingApi($config);
$request = new ConvertImageRequest("sample.jpg", "png", "tempFolder", "My_Storage_Name");
$result = $api->convertImage($request);
Resize, Compare and Crop Images via PHP API
Aspose.Imaging Cloud SDK for PHP has included several important features for working with images inside PHP applications. The SDK allows software developers to load an existing image, resize it and save it in the desired file format. It is also possible to crop an existing image by specifying the position as well as the dimensions of the cropping rectangle. The also supports comparing two images, appending a TIFF image to another TIFF image, applying filter to an Image, Merge multiple TIFF images, update image properties and so on.
Compare Two Images via PHP API
// optional parameters are base URL, API version and debug mode
$imagingConfig = new Configuration();
$imagingConfig->setClientSecret("ClientSecret");
$imagingConfig->setClientId("ClientId");
$imagingApi = new ImagingApi($imagingConfig);
// create search context or use existing search context ID if search context was created earlier
$apiResponse = $imagingApi->createImageSearch(new Requests\CreateImageSearchRequest());
$searchContextId = $apiResponse->getId();
// specify images for comparing (image ID is a path to image in storage)
$imageInStorage1 = "WorkFolder/Image1.jpg";
$imageInStorage2 = "WorkFolder/Image2.jpg";
// compare images
$response = $imagingApi->CompareImages(
new Requests\CompareImagesRequest($searchContextId,
$imageInStorage1, null, $imageInStorage2));
$similarity = $response->getResults()[0]->getSimilarity();
Similar Images Searching via PHP API
Aspose.Imaging Cloud SDK for PHP has included various features allowing software developers to search and find similar images inside their own PHP applications. To achieve the task first you need to upload the images to the cloud storage after that you need to call GetSimilarSearchImage method, wich will finds similar images to the provided one in the cloud storage. This method takes the path to the image and the folder where the similar images should be searched as input parameters. Once the process is completed, you can download the similar images from the cloud storage to the local machine.
Search Similar Images in Cloud via PHP API
$imagingConfig = new Configuration();
$imagingConfig->setClientSecret("ClientSecret");
$imagingConfig->setClientId("ClientId");
$imagingApi = new ImagingApi($imagingConfig);
// create search context or use existing search context ID if search context was created earlier
$apiResponse = $imagingApi->createImageSearch(new Requests\CreateImageSearchRequest());
$searchContextId = $apiResponse->getId();
// extract images features if it was not done before
$imagingApi->createImageFeatures(
new Requests\CreateImageFeaturesRequest(
$searchContextId, null, null, "WorkFolder"))
// wait 'till image features extraction is completed
while ($imagingApi->getImageSearchStatus(
new Requests\GetImageSearchStatusRequest($searchContextId))
->getSearchStatus() !== "Idle")
{
sleep(10);
}
$imageFromStorage = true;
$results = null;
if ($imageFromStorage)
{
// use search image from storage
$storageImageId = "searchImage.jpg";
$results = $imagingApi->findSimilarImages(
new Requests\FindSimilarImagesRequest(
$searchContextId, 90, 5, null, $storageImageId));
}
else
{
// load search image data
$imageData = file_get_contents("D:\\test\\localInputImage.jpg");
$results = $imagingApi->findSimilarImages(
new Requests\FindSimilarImagesRequest($searchContextId, 90, 5, $imageData));
}
// process search results
foreach ($results->getResults() as $searchResult)
{
echo "ImageName: " . $searchResult->getImageId() . "; Similarity: "
. $searchResult->getSimilarity() . "\r\n";
}